中缀算式转化为后缀算式并求值c语言
时间: 2023-05-08 19:00:01 浏览: 187
中缀算式转化为后缀算式:
1. 首先初始化一个栈和一个结果数组
2. 遍历中缀算式,如果是数字则将其直接加入结果数组(可以通过判断字符是否为数字实现),如果是运算符则判断其与栈顶运算符的优先级,如果栈顶运算符优先级大于当前运算符则将栈顶运算符弹出并加入结果数组,然后继续比较新的栈顶运算符和当前运算符,直到遇到优先级小于等于当前运算符的栈顶运算符或者栈为空时,将当前运算符加入栈中
3. 遍历完中缀算式后,如果栈中仍有运算符,则依次弹出并加入结果数组
4. 最终结果数组中存储着后缀算式
后缀算式求值:
1. 初始化一个栈
2. 遍历后缀算式,如果是数字则将其入栈,如果是运算符则弹出栈顶的两个数字进行运算,然后将结果压入栈中
3. 遍历完后缀算式之后,栈中仅剩一个数字,该数字即为所求结果
C语言实现:
中缀算式转后缀:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
int cmp(char a, char b) {
if ((a == '+' || a == '-') && (b == '*' || b == '/')) return -1;
else return 1;
}
void infix2postfix(char *infix, char *postfix) {
char stack[1000];
int top = -1, i = 0, j = 0;
while (infix[i] != '\0') {
if (isdigit(infix[i])) {
postfix[j++] = infix[i++];
} else {
while (top >= 0 && cmp(stack[top], infix[i]) == -1) {
postfix[j++] = stack[top--];
}
stack[++top] = infix[i++];
}
}
while (top >= 0) {
postfix[j++] = stack[top--];
}
postfix[j] = '\0';
}
int eval(char op, int a, int b) {
if (op == '+') return a + b;
else if (op == '-') return a - b;
else if (op == '*') return a * b;
else return a / b;
}
int postfix_eval(char *postfix) {
int stack[1000], top = -1, i = 0;
while (postfix[i] != '\0') {
if (isdigit(postfix[i])) {
stack[++top] = postfix[i++] - '0';
} else {
int b = stack[top--];
int a = stack[top--];
stack[++top] = eval(postfix[i++], a, b);
}
}
return stack[top];
}
int main() {
char infix[1000], postfix[1000];
scanf("%s", infix);
infix2postfix(infix, postfix);
printf("%d\n", postfix_eval(postfix));
return 0;
}
```
注意:实现时可以将操作符的优先级需要值设为更高的整数,以免在处理除法时因为优先级问题导致结果错误。
阅读全文
相关推荐

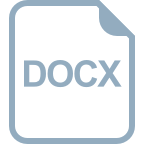
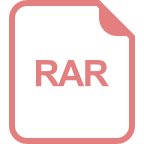
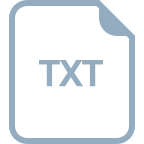
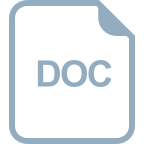







