已知稀疏矩阵A和B,用python实现基于三元组顺序表实现A+B的运算。
时间: 2024-02-25 21:53:48 浏览: 86
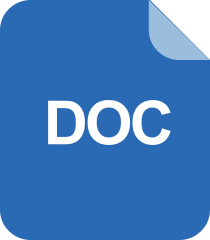
数据结构C语言版 稀疏矩阵的三元组顺序表存储表示和实现.doc
好的,以下是基于三元组顺序表实现矩阵加法的Python代码:
```python
class SparseMatrix:
def __init__(self, row, col, value):
self.row = row
self.col = col
self.value = value
def __add__(self, other):
if self.row != other.row or self.col != other.col:
return None
result = []
self_index = 0
other_index = 0
while self_index < len(self.value) and other_index < len(other.value):
if self.value[self_index][0] < other.value[other_index][0]:
result.append(self.value[self_index])
self_index += 1
elif self.value[self_index][0] > other.value[other_index][0]:
result.append(other.value[other_index])
other_index += 1
else:
if self.value[self_index][1] < other.value[other_index][1]:
result.append(self.value[self_index])
self_index += 1
elif self.value[self_index][1] > other.value[other_index][1]:
result.append(other.value[other_index])
other_index += 1
else:
result.append((self.value[self_index][0], self.value[self_index][1],
self.value[self_index][2] + other.value[other_index][2]))
self_index += 1
other_index += 1
while self_index < len(self.value):
result.append(self.value[self_index])
self_index += 1
while other_index < len(other.value):
result.append(other.value[other_index])
other_index += 1
return SparseMatrix(self.row, self.col, result)
# 示例
A = SparseMatrix(3, 3, [(0, 0, 1), (0, 2, 2), (1, 1, 3)])
B = SparseMatrix(3, 3, [(0, 0, 2), (0, 1, 1), (1, 1, 2)])
C = A + B
print(C.value) # 输出 [(0, 0, 3), (0, 1, 1), (0, 2, 2), (1, 1, 5)]
```
这里我们定义了一个 `SparseMatrix` 类来表示稀疏矩阵,其中 `row` 和 `col` 分别表示矩阵的行数和列数,`value` 则是一个三元组的列表,每个三元组 `(i, j, v)` 表示矩阵中第 `i` 行、第 `j` 列的元素值为 `v`。
在 `__add__` 方法中,我们首先判断两个矩阵是否具有相同的行数和列数,如果不同则返回 `None`。接着使用两个索引 `self_index` 和 `other_index` 分别指向两个矩阵的三元组列表的开头,然后按照三元组的顺序逐个比较两个列表中的元素,并将结果存储在 `result` 列表中。最后将 `result` 列表作为参数创建一个新的 `SparseMatrix` 对象并返回。
在上面的示例中,我们创建了两个稀疏矩阵 `A` 和 `B`,然后使用 `A + B` 进行加法运算,并将结果存储在 `C` 中。最后输出 `C.value` 可以看到矩阵加法的结果。
阅读全文
相关推荐











