osg如何将一个不规则的几何图元生成一张图片
时间: 2024-02-26 08:59:15 浏览: 170
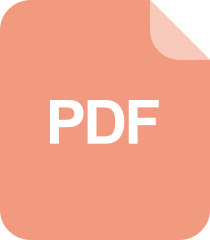
几何体绘制过程-imx219pqh5 datasheet
生成一张不规则几何图元的图像需要使用到OpenSceneGraph(OSG)库中的Geometry类和StateSet类。以下是一个简单的步骤:
1. 创建一个osg::Geometry对象,并添加几何图元数据。
2. 创建一个osg::Geode对象,并将osg::Geometry对象添加到其中。
3. 创建一个osg::StateSet对象,并设置渲染状态。
4. 将osg::StateSet对象设置为osg::Geode对象的状态集。
5. 创建一个osg::Group对象,并将osg::Geode对象添加为其子节点。
6. 创建一个osgViewer::Viewer对象,并将osg::Group对象设置为场景数据。
7. 使用osgDB::writeImageFile函数将场景渲染到图像文件中。
以下是一个简单的示例代码,演示如何使用OSG库中的Geometry类和StateSet类生成一张图像:
```cpp
#include <osg/Geometry>
#include <osg/Geode>
#include <osg/Group>
#include <osgViewer/Viewer>
#include <osgDB/WriteFile>
#include <osg/StateSet>
#include <osg/BlendFunc>
int main()
{
// 创建一个不规则几何图元
osg::ref_ptr<osg::Geometry> irregularGeometry = new osg::Geometry();
// 设置几何图元顶点数据
osg::ref_ptr<osg::Vec3Array> vertices = new osg::Vec3Array();
vertices->push_back(osg::Vec3(-1.0f, 0.0f, 0.0f));
vertices->push_back(osg::Vec3(0.0f, 1.0f, 0.0f));
vertices->push_back(osg::Vec3(1.0f, 0.0f, 0.0f));
vertices->push_back(osg::Vec3(0.0f, -1.0f, 0.0f));
irregularGeometry->setVertexArray(vertices.get());
// 设置几何图元颜色数据
osg::ref_ptr<osg::Vec4Array> colors = new osg::Vec4Array();
colors->push_back(osg::Vec4(1.0f, 0.0f, 0.0f, 1.0f));
colors->push_back(osg::Vec4(0.0f, 1.0f, 0.0f, 1.0f));
colors->push_back(osg::Vec4(0.0f, 0.0f, 1.0f, 1.0f));
colors->push_back(osg::Vec4(1.0f, 1.0f, 0.0f, 1.0f));
irregularGeometry->setColorArray(colors.get());
irregularGeometry->setColorBinding(osg::Geometry::BIND_PER_VERTEX);
// 设置几何图元绘制模式
osg::ref_ptr<osg::DrawElementsUInt> irregularPrimitive = new osg::DrawElementsUInt(osg::PrimitiveSet::QUADS, 0);
irregularPrimitive->push_back(0);
irregularPrimitive->push_back(1);
irregularPrimitive->push_back(2);
irregularPrimitive->push_back(3);
irregularGeometry->addPrimitiveSet(irregularPrimitive.get());
// 创建一个osg::Geode对象,并将osg::Geometry对象添加到其中
osg::ref_ptr<osg::Geode> geode = new osg::Geode();
geode->addDrawable(irregularGeometry.get());
// 创建一个osg::StateSet对象,并设置渲染状态
osg::ref_ptr<osg::StateSet> stateSet = new osg::StateSet();
stateSet->setMode(GL_BLEND, osg::StateAttribute::ON);
stateSet->setRenderingHint(osg::StateSet::TRANSPARENT_BIN);
osg::ref_ptr<osg::BlendFunc> blendFunc = new osg::BlendFunc();
blendFunc->setFunction(osg::BlendFunc::SRC_ALPHA, osg::BlendFunc::ONE_MINUS_SRC_ALPHA);
stateSet->setAttributeAndModes(blendFunc.get(), osg::StateAttribute::ON);
// 将osg::StateSet对象设置为osg::Geode对象的状态集
geode->setStateSet(stateSet.get());
// 创建一个osg::Group对象,并将osg::Geode对象添加为其子节点
osg::ref_ptr<osg::Group> root = new osg::Group();
root->addChild(geode.get());
// 创建一个osgViewer::Viewer对象,并将osg::Group对象设置为场景数据
osg::ref_ptr<osgViewer::Viewer> viewer = new osgViewer::Viewer();
viewer->setSceneData(root.get());
// 渲染场景,并将场景渲染到图像文件中
viewer->frame();
osgDB::writeImageFile(*(viewer->getCamera()->captureRenderToImage()), "output.png", osgDB::Registry::instance()->getWriteFileOptions());
return 0;
}
```
运行代码后,将在程序所在目录下生成名为“output.png”的图像文件,其中包含了一个不规则几何图元。在示例代码中,使用osg::BlendFunc设置了几何图元的渲染状态,使其支持透明度。
阅读全文
相关推荐
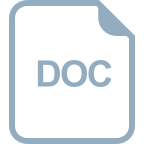
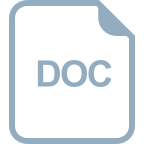
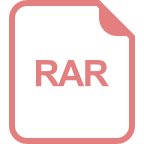
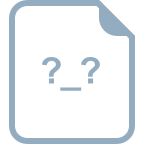
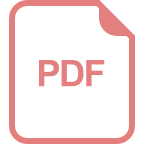
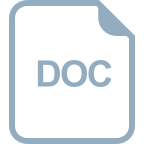
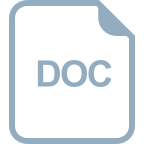








