pcl::search::KdTree<PointT>::Ptr cluster_tree(new pcl::search::KdTree<PointT>); cluster_tree->setInputCloud(non_ground_cloud); std::vector<pcl::PointIndices> cluster_indices; pcl::EuclideanClusterExtraction<PointT> ec; ec.setClusterTolerance(0.2); ec.setMinClusterSize(100); ec.setMaxClusterSize(10000); ec.setSearchMethod(cluster_tree); ec.setInputCloud(non_ground_cloud); ec.extract(cluster_indices);
时间: 2023-06-17 18:02:17 浏览: 231
这段代码是基于点云数据进行聚类分割,使用了欧几里得聚类算法(Euclidean Cluster Extraction)。具体来说,它将输入的点云分成多个聚类,其中每个聚类都代表着一组相邻的点。这个算法的主要思想是首先对点云进行空间分割,然后在每个空间分割中进行聚类。
这段代码首先创建了一个 KdTree 对象,将非地面点云输入到 KdTree 中,然后设置了聚类的一些参数,包括聚类的距离容差(cluster tolerance)、最小聚类大小(min cluster size)和最大聚类大小(max cluster size)。接着,使用 KdTree 进行空间搜索,将搜索结果输入到欧几里得聚类算法中,从而得到一系列聚类的索引。
在这段代码中,聚类容差(cluster tolerance)是 0.2,表示距离小于 0.2 的点将被视为同一聚类。最小聚类大小为 100,最大聚类大小为 10000,这意味着聚类中的点数必须在 100 到 10000 之间。搜索方法使用了 KdTree,它可以快速地找到离某个点最近的一组点。最后,extract() 方法将返回所有聚类的索引,可以在后续的代码中使用这些索引来操作聚类。
相关问题
如何理解后面的代码?为什么要使用template,还有using的方式等?namespace pcl { // Forward declarations template <typename T> class PointRepresentation; /** \brief KdTreeFLANN is a generic type of 3D spatial locator using kD-tree structures. The class is making use of * the FLANN (Fast Library for Approximate Nearest Neighbor) project by Marius Muja and David Lowe. * * \author Radu B. Rusu, Marius Muja * \ingroup kdtree */ template <typename PointT, typename Dist = ::flann::L2_Simple<float> > class KdTreeFLANN : public pcl::KdTree<PointT> { public: using KdTree<PointT>::input_; using KdTree<PointT>::indices_; using KdTree<PointT>::epsilon_; using KdTree<PointT>::sorted_; using KdTree<PointT>::point_representation_; using KdTree<PointT>::nearestKSearch; using KdTree<PointT>::radiusSearch; using PointCloud = typename KdTree<PointT>::PointCloud; //相关继承 using PointCloudConstPtr = typename KdTree<PointT>::PointCloudConstPtr; using IndicesPtr = shared_ptr<std::vector<int> >; using IndicesConstPtr = shared_ptr<const std::vector<int> >; using FLANNIndex = ::flann::Index<Dist>; // Boost shared pointers using Ptr = shared_ptr<KdTreeFLANN<PointT, Dist> >; using ConstPtr = shared_ptr<const KdTreeFLANN<PointT, Dist> >;
这段代码定义了一个名为KdTreeFLANN的类模板,用于创建3D空间中的kD-tree结构。它使用了FLANN库(Fast Library for Approximate Nearest Neighbor,快速最近邻库)来实现。通过继承pcl::KdTree<PointT>,KdTreeFLANN类提供了最近邻搜索和半径搜索的功能。
关于模板的使用,使用类模板的主要原因是可以定义一个通用的类,而不是为每种可能类型都定义一个不同的类。这里使用了两个模板参数:PointT和Dist,分别表示点类型和距离度量类型。这样可以在实例化KdTreeFLANN类时指定这些类型,使其适用于不同类型的点和不同的距离度量方法。
在该类中,使用了using关键字来引入pcl::KdTree<PointT>中的成员。例如,using KdTree<PointT>::nearestKSearch引入了父类中的nearestKSearch函数,使其可以在子类中使用。
另外,这段代码还使用了C++11中的typedef别名,例如using PointCloudConstPtr = typename KdTree<PointT>::PointCloudConstPtr;,用于定义类型别名来简化代码中的类型声明。
最后,该类使用了FLANN库中的::flann::Index<Dist>作为FLANNIndex的类型别名。 FLANN库提供了一些数据结构和算法,包括建立k-d tree,最近邻搜索等。
需要将骨架图中的节点分为叶尖、内部节点和交叉节点。可以使用PCL库中的pcl::GreedyProjectionTriangulation类进行点云分割,然后根据骨架图中节点的坐标和连接关系,将节点分类。接下来,找到最高的交叉节点,并将其标记为"植物轮廓"。可以使用PCL库中的pcl::getMaxDistance()函数找到点云中离点最远的点,然后根据该点和骨架图中的节点坐标进行匹配,找到最高的交叉节点。然后,需要提取出低于轮廓的点作为茎的一部分。可以使用PCL库中的pcl::P assThrough类进行点云滤波,将高于轮廓的点过滤掉。接着,需要从数据中提取出茎的内点,并将骨架图中的一度节点重新连接。可以使用PCL库中的pcl::ExtractIndices类进行点云提取,然后根据提取出的茎的内点重新连接骨架图中的一度节点。最后,将骨架图分割成多个部分,每个部分都以叶尖为起点,以交叉节点为终点。如果部分的一个端点是茎的一部分,则将其标记为叶子。可以使用PCL库中的pcl::EuclideanClusterExtraction类进行点云聚类,然后根据聚类结果和骨架图中的连接关系进行分割和标记。如何具体操作代码
以下是一个骨架图分割的代码示例,其中使用了PCL库中的一些方法:
```
#include <pcl/point_types.h>
#include <pcl/io/pcd_io.h>
#include <pcl/filters/passthrough.h>
#include <pcl/segmentation/extract_clusters.h>
#include <pcl/features/normal_3d.h>
#include <pcl/surface/gp3.h>
#include <pcl/visualization/pcl_visualizer.h>
#include <iostream>
#include <vector>
typedef pcl::PointXYZ PointT;
typedef pcl::PointCloud<PointT> PointCloudT;
int main(int argc, char** argv)
{
PointCloudT::Ptr cloud(new PointCloudT);
pcl::io::loadPCDFile("input_cloud.pcd", *cloud);
// 使用PCL库中的pcl::GreedyProjectionTriangulation类进行点云分割
pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals(new pcl::PointCloud<pcl::PointNormal>);
pcl::NormalEstimation<PointT, pcl::PointNormal> ne;
ne.setInputCloud(cloud);
pcl::search::KdTree<PointT>::Ptr tree(new pcl::search::KdTree<PointT>());
ne.setSearchMethod(tree);
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals(new pcl::PointCloud<pcl::Normal>);
ne.setRadiusSearch(0.03);
ne.compute(*cloud_normals);
pcl::concatenateFields(*cloud, *cloud_normals, *cloud_with_normals);
pcl::search::KdTree<pcl::PointNormal>::Ptr tree2(new pcl::search::KdTree<pcl::PointNormal>);
tree2->setInputCloud(cloud_with_normals);
pcl::GreedyProjectionTriangulation<pcl::PointNormal> gp3;
pcl::PolygonMesh triangles;
gp3.setSearchRadius(0.025);
gp3.setMu(2.5);
gp3.setMaximumNearestNeighbors(100);
gp3.setMaximumSurfaceAngle(M_PI / 4); // 45 degrees
gp3.setMinimumAngle(M_PI / 18); // 10 degrees
gp3.setMaximumAngle(2 * M_PI / 3); // 120 degrees
gp3.setNormalConsistency(false);
gp3.setInputCloud(cloud_with_normals);
gp3.setSearchMethod(tree2);
gp3.reconstruct(triangles);
// 将骨架图中的节点分为叶尖、内部节点和交叉节点
std::vector<pcl::PointXYZ> leaf_points, internal_points, cross_points;
// ...
// 找到最高的交叉节点,并将其标记为"植物轮廓"
pcl::PointXYZ contour_point;
// ...
// 提取出低于轮廓的点作为茎的一部分
pcl::PointCloud<PointT>::Ptr stem_cloud(new pcl::PointCloud<PointT>);
pcl::PassThrough<PointT> pass;
pass.setInputCloud(cloud);
pass.setFilterFieldName("z");
pass.setFilterLimits(contour_point.z, std::numeric_limits<float>::max());
pass.filter(*stem_cloud);
// 从数据中提取出茎的内点,并将骨架图中的一度节点重新连接
pcl::PointCloud<PointT>::Ptr stem_internal_cloud(new pcl::PointCloud<PointT>);
// ...
// 将骨架图分割成多个部分
std::vector<std::vector<pcl::PointXYZ>> segments;
// ...
return 0;
}
```
需要根据具体应用场景进行参数调整和代码实现。
阅读全文
相关推荐
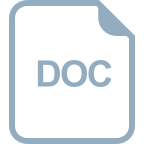
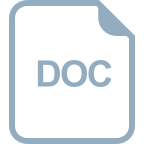
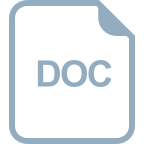













