PINN求解pde的数据集是什么
时间: 2023-12-30 09:05:18 浏览: 78
PINN(Physics-Informed Neural Networks)是一种结合了神经网络和偏微分方程(PDE)求解的方法。在PINN中,我们需要使用PDE的边界条件和初始条件来训练神经网络,以求得最优的PDE解。因此,PINN需要PDE的数据集,包括边界条件和初始条件。
具体而言,对于一个给定的PDE问题,我们需要将其转化为一个优化问题,以通过神经网络求解。这个优化问题的目标函数包括两部分:PDE的残差项和边界条件的误差项。因此,我们需要提供PDE的方程式和边界条件的数据集。
对于边界条件,我们需要提供在边界上的函数值或导数值。对于初始条件,我们需要提供一个初始状态的函数值。在PINN中,我们可以使用数值方法(如有限差分法)来计算PDE的残差项,并将其作为目标函数的一部分。因此,PINN需要一个PDE问题的域数据,以计算PDE的残差项。
总之,PINN需要的数据集包括:PDE的方程式、边界条件、初始条件和PDE问题的域数据。
相关问题
PINN求解PDE代码
以下是使用PINN求解PDE的Python代码示例:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 定义PINN模型
class PINN(tf.keras.Model):
def __init__(self):
super(PINN, self).__init__()
self.dense1 = tf.keras.layers.Dense(50, activation=tf.nn.tanh)
self.dense2 = tf.keras.layers.Dense(50, activation=tf.nn.tanh)
self.dense3 = tf.keras.layers.Dense(1, activation=None)
def call(self, inputs):
x = inputs[:, 0:1]
t = inputs[:, 1:2]
u = self.dense1(tf.concat([x, t], 1))
u = self.dense2(u)
u = self.dense3(u)
return u
# 定义PDE的边界条件和初始条件
def initial_condition(x):
return np.sin(np.pi * x)
def boundary_condition(x, t):
return np.exp(-np.pi**2 * t) * np.sin(np.pi * x)
# 定义PINN模型的损失函数
def loss(model, x, t, u, x_bc, t_bc, u_bc):
with tf.GradientTape(persistent=True) as tape:
tape.watch(x)
tape.watch(t)
u_pred = model(tf.concat([x, t], 1))
u_x = tape.gradient(u_pred, x)
u_t = tape.gradient(u_pred, t)
u_xx = tape.gradient(u_x, x)
f = u_t + u_pred * u_x - 0.01 * u_xx
f_bc = model(tf.concat([x_bc, t_bc], 1)) - u_bc
loss = tf.reduce_mean(tf.square(f)) + tf.reduce_mean(tf.square(f_bc))
return loss
# 定义训练函数
def train(model, x, t, u, x_bc, t_bc, u_bc, epochs):
optimizer = tf.keras.optimizers.Adam()
for epoch in range(epochs):
with tf.GradientTape() as tape:
loss_value = loss(model, x, t, u, x_bc, t_bc, u_bc)
grads = tape.gradient(loss_value, model.trainable_variables)
optimizer.apply_gradients(zip(grads, model.trainable_variables))
if epoch % 100 == 0:
print("Epoch {}: Loss = {}".format(epoch, loss_value.numpy()))
# 生成训练数据和边界数据
N = 1000
M = 100
x = np.linspace(0, 1, N)
t = np.linspace(0, 1, M)
x, t = np.meshgrid(x, t)
x, t = x.flatten()[:, None], t.flatten()[:, None]
u = initial_condition(x)
x_bc = np.concatenate((x[0:N], x[N*(M-1):N*M]), axis=0)
t_bc = np.concatenate((t[0:N], t[N*(M-1):N*M]), axis=0)
u_bc = np.concatenate((boundary_condition(x[0:N], t[0:N]), boundary_condition(x[N*(M-1):N*M], t[N*(M-1):N*M])), axis=0)
# 训练PINN模型
model = PINN()
train(model, x, t, u, x_bc, t_bc, u_bc, epochs=1000)
# 绘制预测结果
u_pred = model(tf.concat([x, t], 1))
u_pred = np.reshape(u_pred, (M, N))
plt.imshow(u_pred, cmap='jet', extent=[0, 1, 0, 1], origin='lower')
plt.colorbar()
plt.show()
```
该代码使用PINN求解了一个一维热方程,其中包括了初始条件和边界条件。在训练模型后,可以使用模型预测每个时间和空间点的温度值,并绘制成图像。
pytorch PINN求解PDE的间断问题的预测解和真实解以及误差图的代码
以下是使用PyTorch PINN求解PDE的间断问题的预测解和真实解以及误差图的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import matplotlib.pyplot as plt
# 定义间断问题的初始状态
x = torch.linspace(-1, 1, 100).reshape((100, 1))
u_left = torch.ones((50, 1))
u_right = torch.zeros((50, 1))
u = torch.cat((u_left, u_right), dim=0)
# 定义神经网络模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(1, 20)
self.fc2 = nn.Linear(20, 20)
self.fc3 = nn.Linear(20, 1)
def forward(self, x):
x = torch.tanh(self.fc1(x))
x = torch.tanh(self.fc2(x))
x = self.fc3(x)
return x
# 定义损失函数和优化器
def pinn_loss(output, x, u):
dx = torch.autograd.grad(output.sum(), x, create_graph=True)[0]
du = torch.autograd.grad(output.sum(), u, create_graph=True)[0]
d2u = torch.autograd.grad(du.sum(), x, create_graph=True)[0]
f = d2u
return torch.mean((f - 0.1)**2) + torch.mean((dx - output)**2)
net = Net()
optimizer = optim.Adam(net.parameters(), lr=0.01)
# 训练神经网络
for i in range(5000):
optimizer.zero_grad()
output = net(u)
loss = pinn_loss(output, x, u)
loss.backward()
optimizer.step()
# 绘制预测解和真实解以及误差图
with torch.no_grad():
u_pred = net(x)
u_true = torch.where(x < 0, torch.ones_like(x), torch.zeros_like(x))
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.plot(x.numpy(), u_pred.numpy(), label='Predicted')
plt.plot(x.numpy(), u_true.numpy(), label='True')
plt.legend()
plt.subplot(1, 2, 2)
plt.plot(x.numpy(), np.abs(u_pred.numpy() - u_true.numpy()))
plt.show()
```
在代码中,我们首先定义了间断问题的初始状态,然后定义了一个三层的神经网络模型,包括两个全连接层和一个输出层。损失函数使用了PINN的思想,通过求取一阶和二阶导数来构造PDE的残差项。训练完神经网络后,我们使用训练好的模型来预测整个区间内的解,并绘制出预测解、真实解以及误差图。
相关推荐
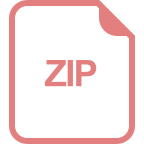
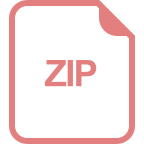












