绘制动图,显示蒙特卡洛求解过程中不同点的精度。python代码,曲线
时间: 2024-02-24 13:57:57 浏览: 24
好的,我会尽力回答你的问题。首先需要明确一下,你是想要绘制蒙特卡洛求解过程中不同点的精度曲线,还是想要绘制动图来展示这个过程呢?
如果是前者,那么可以使用 Python 中的 Matplotlib 库来绘制曲线图。具体实现代码可参考以下示例:
```python
import matplotlib.pyplot as plt
import random
# 定义求解函数
def monte_carlo(n):
count = 0
for i in range(n):
x = random.uniform(0, 1)
y = random.uniform(0, 1)
if (x ** 2 + y ** 2) <= 1:
count += 1
return 4 * count / n
# 绘制曲线图
x = [i for i in range(10, 10000, 10)]
y = [monte_carlo(i) for i in x]
plt.plot(x, y)
plt.xlabel('Number of Points')
plt.ylabel('Estimation of Pi')
plt.title('Monte Carlo Estimation of Pi')
plt.show()
```
上述代码中,我们定义了一个求解函数 `monte_carlo`,它接受一个参数 `n` 表示采样点的数量,然后利用蒙特卡洛方法求解圆周率的估计值。接着,我们使用 `Matplotlib` 库绘制了一条曲线,横轴表示采样点的数量,纵轴表示圆周率的估计值。最后,我们使用 `plt.show()` 函数将曲线图显示出来。
如果是后者,那么可以使用 Python 中的 Matplotlib 和 FuncAnimation 库来绘制动图。具体实现代码可参考以下示例:
```python
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import random
# 设置动图参数
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
xdata, ydata = [], []
# 定义求解函数
def monte_carlo(n):
count = 0
for i in range(n):
x = random.uniform(0, 1)
y = random.uniform(0, 1)
if (x ** 2 + y ** 2) <= 1:
count += 1
xdata.append(x)
ydata.append(y)
return 4 * count / n
# 定义更新函数
def update(frame):
n = 10 * (frame + 1)
pi = monte_carlo(n)
line.set_data(xdata, ydata)
ax.set_title('Estimation of Pi: %.4f' % pi)
return line,
# 绘制动图
ani = animation.FuncAnimation(fig, update, frames=100, interval=100, blit=True)
plt.show()
```
上述代码中,我们首先设置了动图的参数,包括画布 `fig`、坐标轴 `ax`、线条 `line` 等。然后,我们定义了一个求解函数 `monte_carlo`,它接受一个参数 `n` 表示采样点的数量,然后利用蒙特卡洛方法求解圆周率的估计值,并将每个采样点的坐标记录下来。最后,我们利用 `FuncAnimation` 函数来实现动图的绘制,其中 `update` 函数用于更新每一帧的内容,`frames` 参数表示动图的帧数,`interval` 参数表示每帧之间的间隔时间,`blit` 参数表示是否使用 blitting 技术来提高绘制效率。
希望以上示例对你有所帮助!如果还有其他问题,请随时提出。
相关推荐
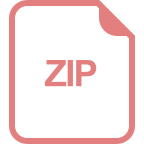














