如果mat,map,vector有不是固定的维度呢?
时间: 2024-03-13 22:45:01 浏览: 20
如果`std::vector`、`std::map`或`cv::Mat`类型的数据不是固定的维度,我们可以使用递归调用`print`函数,并在每一层递归中判断当前元素的类型,如果是容器类型则继续递归输出它的元素,否则直接输出当前元素。下面是一个示例代码:
```c++
#include <iostream>
#include <map>
#include <vector>
#include <type_traits>
#include <opencv2/core.hpp>
template<typename T>
void print(const T& t)
{
std::cout << t << std::endl;
}
template<typename T>
void print_container(const T& t)
{
std::cout << "{";
for (const auto& e : t) {
print(e);
}
std::cout << "}" << std::endl;
}
template<typename T>
void print_container(const cv::Mat_<T>& m)
{
std::cout << "{";
for (int i = 0; i < m.rows; ++i) {
for (int j = 0; j < m.cols; ++j) {
print(m(i, j));
}
std::cout << std::endl;
}
std::cout << "}" << std::endl;
}
template<typename T>
void print(const T& t)
{
if constexpr (std::is_container_v<T> && !std::is_same_v<T, std::string>) {
print_container(t);
} else {
std::cout << t << std::endl;
}
}
template<typename T, typename... Args>
void print(const T& t, const Args&... args)
{
print(t);
print(args...);
}
int main()
{
std::vector<int> v1 = {1, 2, 3};
std::vector<std::vector<int>> v2 = {{1, 2}, {3, 4, 5}, {6}};
std::map<std::string, std::vector<int>> m = {{"a", {1, 2, 3}}, {"b", {4, 5}}, {"c", {6}}};
cv::Mat_<double> mat1 = (cv::Mat_<double>(2, 2) << 1.0, 2.0, 3.0, 4.0);
cv::Mat_<cv::Vec3b> mat2 = (cv::Mat_<cv::Vec3b>(2, 2) << cv::Vec3b(1, 2, 3), cv::Vec3b(4, 5, 6), cv::Vec3b(7, 8, 9), cv::Vec3b(10, 11, 12));
print(v1, v2, m, mat1, mat2);
return 0;
}
```
在上面的代码中,我们添加了一个新的函数模板`print_container`,用于输出容器类型的元素。在`print`函数中,我们使用`if constexpr`语句来判断当前元素是否是容器类型,如果是,则递归调用`print_container`函数输出它的元素,否则直接输出当前元素。
这样,我们就可以处理任意维度的`std::vector`、`std::map`和`cv::Mat`类型的数据了。注意,对于`std::string`类型的数据,我们需要特殊处理,否则会出现编译错误。
相关推荐
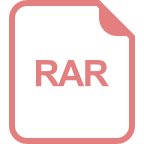
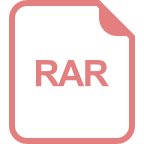














