vis = o3d.visualization.Visualizer() vis.create_window() 修改
时间: 2023-06-19 10:03:23 浏览: 638
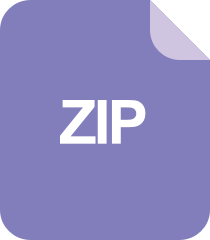
neovis.js:Neo4j + vis.js = neovis.js。 使用Neo4j中的数据在浏览器中图形化可视化-源码

这行代码的作用是创建一个可视化窗口。如果要修改窗口的大小或者标题,可以在`create_window()`函数中传入相应的参数。例如:
```python
vis.create_window(
width=800, height=600,
window_name='My Window'
)
```
这样就会创建一个宽度为800,高度为600,标题为"My Window"的窗口。
阅读全文
相关推荐
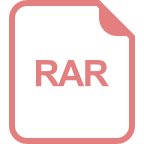
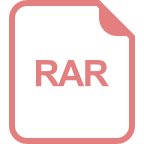















