public class DottedLine : MonoBehaviour { public List<GameObject> gameObjects; public List<string> wharfId; public bool showBerthingArea = true; public bool showNotifyArea = true; public string currentId = null; void Start() { Transform transforms = transform.GetComponentInChildren<Transform>(); foreach (Transform tf in transforms) { gameObjects.Add(tf.gameObject); } gameObjects.Remove(gameObject); } public void UpdateHeightLight(string id, bool berthingArea, bool notifyArea) { foreach (GameObject go in gameObjects) { if (id == null || go.name.Contains(id)) { LineRenderer line = go.GetComponent<LineRenderer>(); if (line != null) { bool show = false; if (go.name.Contains("BerthingArea")) { show = berthingArea; } else if (go.name.Contains("NotifyArea")) { show = notifyArea; } line.enabled = show; } } } } public void ShowAssignId(string id) { currentId = id; Debug.LogError($"current:{ currentId}"); UpdateHeightLight(id, showBerthingArea, showNotifyArea); } public void ShowAllHeightLight() { currentId = null; UpdateHeightLight(null, true, true); } public void HideAllHeightLight() { currentId = null; UpdateHeightLight(null, false, false); } public void ToggleBerthingArea() { showBerthingArea = !showBerthingArea; UpdateHeightLight(currentId, showBerthingArea, showNotifyArea); } public void ToggleNotifyArea() { showNotifyArea = !showNotifyArea; UpdateHeightLight(currentId, showNotifyArea, showNotifyArea); } 和public class LineRendererController : MonoBehaviour { public DottedLine dottedLine; void Start() { GameObject dock = GameObject.Find("604"); if (dock != null) { // 将游戏对象的名称作为id传递给dottedLine.ShowAssignId()方法 dottedLine.ShowAssignId(dock.name); // 显示该游戏对象的停靠区 dock.GetComponent<DottedLine>().showBerthingArea = true; // 隐藏该游戏对象的通知区 dock.GetComponent<DottedLine>().showNotifyArea = false; }这两段代码要想实现,unity中该怎么操作
时间: 2024-02-10 08:33:18 浏览: 85
这两段代码是 Unity 中的 C# 脚本,需要将它们添加到 Unity 的 GameObject 上,然后通过在代码中调用 GameObject 的属性和方法来操作它们。具体步骤如下:
1. 创建一个空的 GameObject,给它一个有意义的名称,例如 "DottedLineController" 或 "LineRendererController"。
2. 将 DottedLine 脚本和 LineRendererController 脚本添加到 GameObject 上,可以通过拖拽脚本文件到 Inspector 视图中的 Add Component 按钮来完成。
3. 在场景中找到需要操作的 GameObject,例如上面代码中的 "604"。
4. 将该 GameObject 上的 DottedLine 组件的 showBerthingArea 和 showNotifyArea 属性设置为需要的值,可以在 Inspector 视图中找到这两个属性。
5. 在 LineRendererController 脚本中,通过 GameObject.Find() 方法找到需要操作的 GameObject,并将它的名称传递给 dottedLine.ShowAssignId() 方法。
6. 在 Unity 编辑器中运行场景,可以通过调用 DottedLine 和 LineRendererController 脚本中的方法来显示、隐藏、切换停靠区和通知区。
相关问题
public class IBMonoSingleton<T> : MonoBehaviour where T: IBMonoSingleton<T>
这是一个泛型类 IBMonoSingleton,它继承自 MonoBehaviour,并且要求 T 类型也必须继承自 IBMonoSingleton<T>。
这个类的作用是实现单例模式(Singleton pattern),保证某个类在程序中只存在一个实例。在泛型类中使用单例模式,可以让任何继承自 IBMonoSingleton<T> 的类都拥有单例的能力。
同时,这个类还继承自 MonoBehaviour,意味着它可以作为 Unity 中的组件使用。由于 Unity 中的组件都必须继承自 MonoBehaviour,因此这个泛型类也要求 T 必须继承自 MonoBehaviour。
using System.Collections; using System.Collections.Generic; using UnityEngine; using Assets; public class DottedLine : MonoBehaviour, ILineRendererController { public List<GameObject> gameObjects; public void ToggleLineRenderer() { foreach (GameObject item in gameObjects) { LineRenderer line = item.GetComponent<LineRenderer>(); line.enabled = !line.enabled; } } void Start() { var transforms = transform.GetComponentInChildren<Transform>(); foreach (Transform tf in transforms) { gameObjects.Add(tf.gameObject); } gameObjects.Remove(gameObject); } } 这是之前写的控制一个码头的现实与隐藏。现在的需求是在unity中,怎么通过控制开关来控制每个密码头戒线的高亮,其中警戒线分为两个块区域(berthingArea和notifyArea),每个密码头都有自己的id,然后后面的方法里都有这些参数,可以只显示一个id的一个区域,也可以显示两个区域,也可以显示所有id的高亮,也可以隐藏所有id的高亮,请详细的写一下完整代码
以下是修改后的代码:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DottedLine : MonoBehaviour
{
public List<GameObject> gameObjects;
public List<string> ids; // 存储所有密码头的id
public bool showBerthingArea = true; // 是否显示停靠区域
public bool showNotifyArea = true; // 是否显示通知区域
public string currentId = ""; // 当前选择的密码头id
private void Start()
{
Transform[] transforms = GetComponentsInChildren<Transform>();
foreach (Transform tf in transforms)
{
GameObject go = tf.gameObject;
if (go != gameObject)
{
gameObjects.Add(go);
}
}
}
// 根据id和显示选项,更新高亮状态
private void UpdateHighlight(string id, bool showBerthing, bool showNotify)
{
foreach (GameObject go in gameObjects)
{
if (id == "" || go.name.Contains(id))
{
LineRenderer line = go.GetComponent<LineRenderer>();
if (line != null)
{
bool show = false;
if (go.name.Contains("BerthingArea"))
{
show = showBerthing;
}
else if (go.name.Contains("NotifyArea"))
{
show = showNotify;
}
line.enabled = show;
}
}
}
}
// 显示指定id的高亮
public void ShowById(string id)
{
currentId = id;
UpdateHighlight(id, showBerthingArea, showNotifyArea);
}
// 显示所有id的高亮
public void ShowAll()
{
currentId = "";
UpdateHighlight("", showBerthingArea, showNotifyArea);
}
// 隐藏所有id的高亮
public void HideAll()
{
currentId = "";
UpdateHighlight("", false, false);
}
// 显示或隐藏停靠区域
public void ToggleBerthingArea()
{
showBerthingArea = !showBerthingArea;
UpdateHighlight(currentId, showBerthingArea, showNotifyArea);
}
// 显示或隐藏通知区域
public void ToggleNotifyArea()
{
showNotifyArea = !showNotifyArea;
UpdateHighlight(currentId, showBerthingArea, showNotifyArea);
}
}
```
在这个脚本中,我们添加了一些新的变量和方法来实现新的需求:
- `ids`:存储所有密码头的id,可以在Inspector中进行设置。
- `showBerthingArea`和`showNotifyArea`:控制是否显示停靠区域和通知区域。
- `currentId`:保存当前选择的密码头id。
- `UpdateHighlight`方法:根据id和显示选项,更新高亮状态。
- `ShowById`方法:显示指定id的高亮。
- `ShowAll`方法:显示所有id的高亮。
- `HideAll`方法:隐藏所有id的高亮。
- `ToggleBerthingArea`和`ToggleNotifyArea`方法:显示或隐藏停靠区域和通知区域。
在Inspector中,可以添加所有密码头的id和需要高亮的LineRenderer:

接下来,可以在代码中调用这些方法来实现不同的显示效果:
```csharp
public class Example : MonoBehaviour
{
public DottedLine dottedLine;
void Start()
{
// 显示指定id的停靠区域和通知区域
dottedLine.ShowById("001");
dottedLine.showBerthingArea = true;
dottedLine.showNotifyArea = true;
// 显示所有id的通知区域
dottedLine.ShowAll();
dottedLine.showBerthingArea = false;
dottedLine.showNotifyArea = true;
// 隐藏所有id的高亮
dottedLine.HideAll();
}
}
```
这样就可以根据需求控制每个密码头的高亮了。
阅读全文
相关推荐
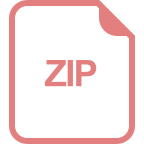
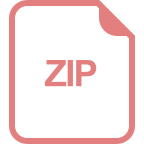
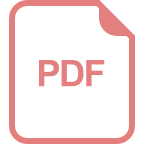














