NPC.set_velocity(carla.Vector3D(x=50, y=0, z=0)) AttributeError: 'Vehicle' object has no attribute 'set_velocity'
时间: 2024-03-24 07:36:04 浏览: 293
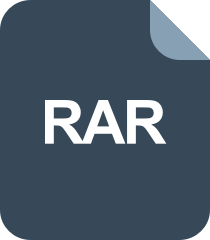
vtl.rar_VTL.rar_velocity
非常抱歉,我的回答有误。在Carla 0.9.13中,Vehicle类没有set_velocity方法,而是使用apply_control方法来控制车辆的行驶。可以通过Carla提供的Control类来创建控制信号,从而控制车辆的速度、转向等行驶行为。具体代码如下:
```python
import carla
# 连接到Carla服务器
client = carla.Client('localhost', 2000)
client.set_timeout(10.0)
# 获取世界
world = client.get_world()
# 获取所有的NPC角色
npc_list = world.get_actors().filter('vehicle.*')
# 设置NPC的速度
for npc in npc_list:
control = carla.VehicleControl(throttle=1.0, steer=0.0)
npc.apply_control(control)
```
上述代码中,我们首先连接到Carla服务器,然后获取世界中所有的NPC角色。接着,我们创建一个Control对象,设置throttle为1.0,表示车辆全速行驶,steer为0.0,表示车辆直行。最后,我们通过npc.apply_control(control)方法将控制信号应用到NPC角色上,从而控制其行驶。您也可以根据需要调整Control对象的属性值,从而控制NPC角色的行驶行为。
阅读全文
相关推荐
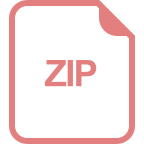
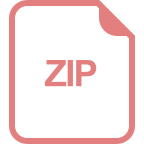

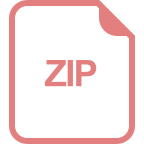
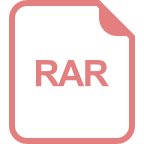
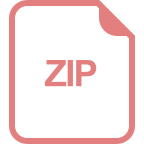
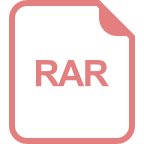
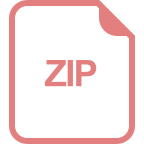
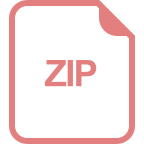
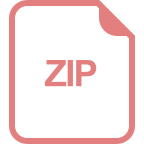
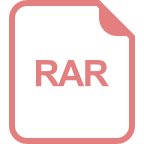
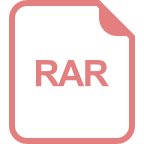
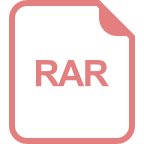
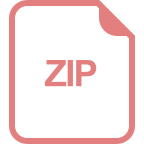
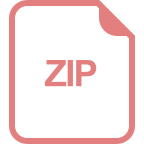