异步IO深度解析:Python asyncio的高效使用技巧
发布时间: 2024-09-01 02:47:59 阅读量: 291 订阅数: 108 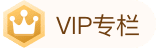
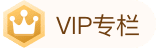
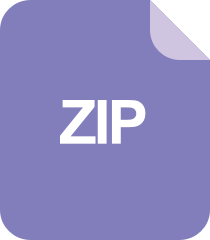
搜寻器:python的网络搜寻器
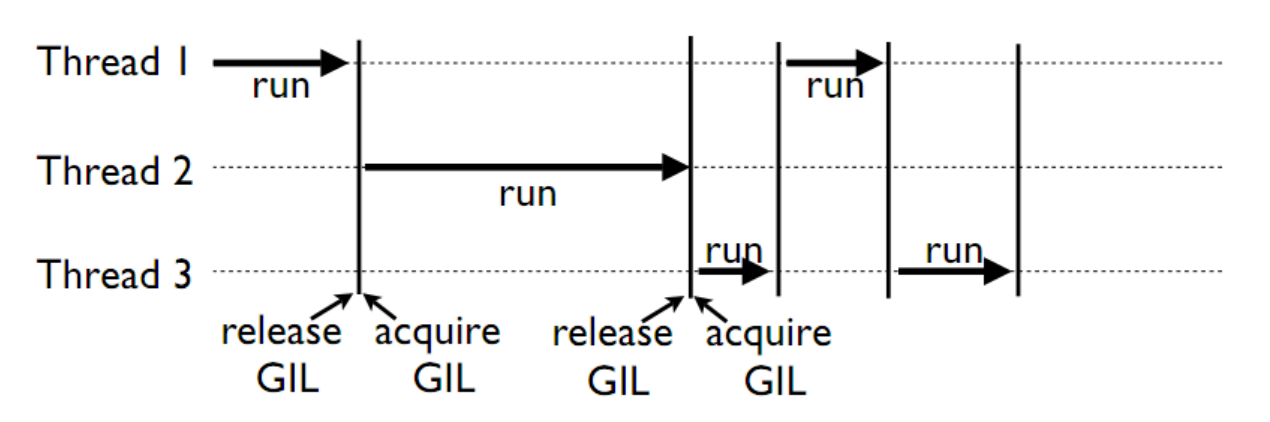
# 1. 异步IO与Python asyncio简介
在现代计算机编程中,异步IO(异步输入/输出)是一种强大的并发模型,它允许程序在等待诸如文件读写、网络请求等耗时操作完成时继续执行其他任务。Python中的`asyncio`模块是实现异步IO编程的核心库,它提供了一系列基础设施,使得编写基于事件循环的异步代码变得简单而高效。
Python 3.5引入了`async`和`await`关键字,这使得编写异步代码更加直观。`asyncio`库的出现标志着Python异步编程的一大步,其提供了全面的API,支持TCP和UDP套接字,以及子进程和定时器等。
本章将深入介绍`asyncio`库的基础概念和用途,旨在为读者建立对异步编程和`asyncio`模块的初步理解。我们会从异步IO的基本概念开始,逐步探讨Python中异步编程的历史与发展,最终通过实际的代码示例,展示如何在Python中运用`asyncio`来编写异步代码。通过这一章的学习,读者应能掌握异步编程的基础,并在自己的项目中尝试使用`asyncio`。
```python
import asyncio
async def main():
print('Hello')
await asyncio.sleep(1)
print('... asyncio')
asyncio.run(main())
```
上述代码展示了如何使用`asyncio`模块来创建一个异步程序,其中`asyncio.sleep(1)`模拟了一个异步操作。通过这一简单的例子,我们可以开始探索`asyncio`世界的大门。接下来的章节会更深入地剖析`asyncio`,引导你成为异步编程的行家里手。
# 2. 深入理解异步IO模型
## 2.1 异步IO的基本概念
### 2.1.1 同步与异步的区别
在深入探讨Python中的异步IO之前,我们首先需要了解同步与异步的基本区别。在传统的同步编程模型中,每次只能执行一个任务,一个任务的完成必须等待前一个任务结束。这种方式简单直观,但存在效率问题,尤其是在遇到I/O密集型任务时,CPU资源可能会被大量闲置。
异步编程则允许程序在等待一个长时间运行的任务(如I/O操作)时,继续执行其他操作,而不是简单地等待。这种方式提高了程序在等待期间的资源利用率,使得应用程序可以更有效地处理并发任务。
举一个简单的例子,想象一个网络请求的场景。在一个同步模型中,程序发起一个请求后,会阻塞等待响应,这段时间内CPU资源会被闲置。而在异步模型中,程序在发起请求后,可以立即继续执行其他代码,当请求响应到来时,通过回调函数或其他机制来处理结果。
### 2.1.2 异步IO的工作原理
异步IO的工作原理基于非阻塞I/O操作和事件循环机制。非阻塞I/O允许程序在发起I/O请求后立即返回,继续执行其他任务,而不是挂起等待I/O操作完成。当I/O操作完成后,会通知程序并提供数据。
事件循环(Event Loop)是异步IO模型的核心,它负责管理所有的事件和回调函数。每当一个事件(如I/O完成)发生时,事件循环就会调用相应的回调函数来处理这个事件。一个简单的事件循环伪代码如下:
```python
while True:
event = event_queue.get()
handle_event(event)
```
在这个循环中,`event_queue`是一个事件队列,`handle_event`是一个处理事件的函数。程序不断从队列中获取事件并处理,这样就实现了对多个并发任务的管理。
## 2.2 Python中异步编程的历史与发展
### 2.2.1 Python的异步编程变迁
Python语言在早期并没有提供原生的异步编程支持。直到Python 3.4,asyncio库的出现,才正式将异步编程引入Python的标准库中。在asyncio之前,Python开发者通常会使用基于线程的并发或者使用第三方库如gevent来实现异步行为。
早期的Python异步编程主要依赖于回调函数,这虽然可以实现非阻塞的I/O操作,但随着时间的推移,人们发现这种模式可读性差,维护成本高,代码中充满了所谓的"回调地狱"(callback hell)。
### 2.2.2 asyncio库的出现与特点
asyncio库的出现,使得Python开发者能够使用基于协程的异步编程模型。asyncio提供了一种全新的方式来编写单线程并发代码,使得异步编程变得更加直观和高效。
asyncio的几个核心特点如下:
- 协程(Coroutines):asyncio使用协程来表示轻量级的线程,这些协程通过`async`和`await`关键字进行定义和调用。
- 事件循环(Event Loop):是asyncio库的核心组件,负责管理所有协程的运行。
- Future对象:用于表示异步操作的最终结果,可以被协程等待。
- Task对象:是对Future的封装,提供了一些额外的功能,比如取消操作。
在asyncio中,开发者可以写出类似同步代码的异步版本,这大大降低了异步编程的学习曲线,同时也改善了代码的可读性和可维护性。
## 2.3 asyncio的核心组件
### 2.3.1 Event Loop的内部机制
Event Loop是asyncio中的核心组件,负责管理所有的协程和I/O事件。在深入理解Event Loop之前,我们先来看一个简单的伪代码示例:
```python
import asyncio
async def main():
# 这里可以调用其他协程
pass
# 创建事件循环
loop = asyncio.get_event_loop()
# 运行协程
loop.run_until_complete(main())
# 关闭事件循环
loop.close()
```
事件循环通过一系列的步骤来处理事件:
1. 创建一个事件循环对象。
2. 通过`run_until_complete`或`run_forever`等方法启动事件循环。
3. 事件循环不断等待事件到来,例如I/O操作完成、定时器触发等。
4. 当事件到来时,事件循环将调用相应的回调函数或协程来处理事件。
5. 处理完事件后,事件循环继续等待下一个事件。
在事件循环中,每个事件都有其优先级,事件循环会按照优先级来处理。Python 3.7引入的`asyncio.run()`函数简化了事件循环的使用,是运行顶级脚本或快速试验asyncio的推荐方法。
### 2.3.2 Future和Task对象详解
在asyncio中,Future对象表示一个尚未完成但预期会完成的操作的结果。Future对象可以被协程等待,直到它完成。
Future对象通常不需要直接创建,而是通过异步函数返回或由事件循环的某些操作(如网络操作)自动创建。一旦Future对象的状态变为"完成",就可以通过`await`来获取结果。
Task对象是对Future的封装,它具有额外的功能。创建Task通常通过`asyncio.create_task()`函数完成。Task对象允许协程与其他任务并发运行,并提供了取消任务的方法。
下面是一个使用Future和Task的例子:
```python
import asyncio
async def wait_for(future):
await future
print("Future完成")
async def main():
# 创建一个Future对象
fut = asyncio.Future()
# 创建一个Task对象
task = asyncio.create_task(wait_for(fut))
# 模拟Future完成
await asyncio.sleep(1)
fut.set_result('已设置结果')
# 等待Task完成
await task
asyncio.run(main())
```
在这个例子中,`wait_for`是一个等待Future完成的协程。在`main`协程中,我们创建了一个未完成的Future对象和一个Task对象,然后模拟Future对象的完成,并等待Task对象的结束。
### 2.3.3 异步迭代器和异步上下文管理器
异步迭代器和异步上下文管理器是Python 3.6中引入的两个新概念,它们允许开发者编写可以处理异步迭代和异步上下文管理的代码。
异步迭代器通过实现`__aiter__()`和`__anext__()`方法来支持`async for`循环。这样的迭代器可以让异步代码以简洁的方式遍历元素:
```python
class AsyncRange:
def __init__(self, start, stop):
self.current = start
self.stop = stop
async def __aiter__(self):
return self
async def __anext__(self):
if self.current < self.stop:
value = self.current
self.current += 1
return value
else:
raise StopAsyncIteration
async def main():
async for i in AsyncRange(0, 5):
print(i)
asyncio.run(main())
```
异步上下文管理器通过实现`__aenter__()`和`__aexit__()`方法来支持`async with`语句。这在需要异步操作来建立或释放资源时特别有用,例如异步数据库连接或异步文件操作:
```python
import asyncio
class AsyncContextManager:
async def __aenter__(self):
print("进入异步上下文管理器")
await asyncio.sleep(1)
return self
async def __aexit__(self, exc_type, exc_val, exc_tb):
print("退出异步上下文管理器")
await asyncio.sleep(1)
async def main():
async with AsyncContextManager() as manager:
print("在上下文管理器中")
asyncio.run(main())
```
在这个例子中,`AsyncContextManager`类定义了一个异步上下文管理器,当使用`async with`时,会自动调用`__aenter__`和`__aexit__`方法来管理资源的获取和释放。
在异步编程中,使用这些高级特性可以帮助开发者编写更加高效、可读性更强的代码。
# 3. Python asyncio的使用技巧
## 3.1 编写基础的异步程序
编写异步程序是掌握异步IO的关键步骤,涉及理解事件循环(Event Loop)
0
0
相关推荐
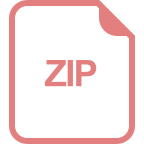
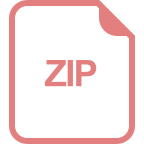
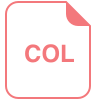
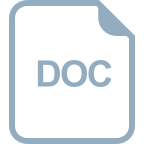
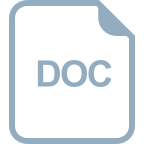
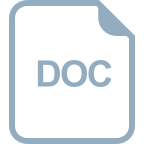
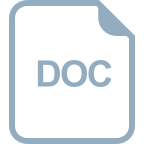
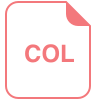