Java设计模式在框架中的应用
发布时间: 2024-08-30 06:15:04 阅读量: 103 订阅数: 34 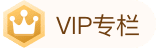
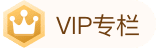
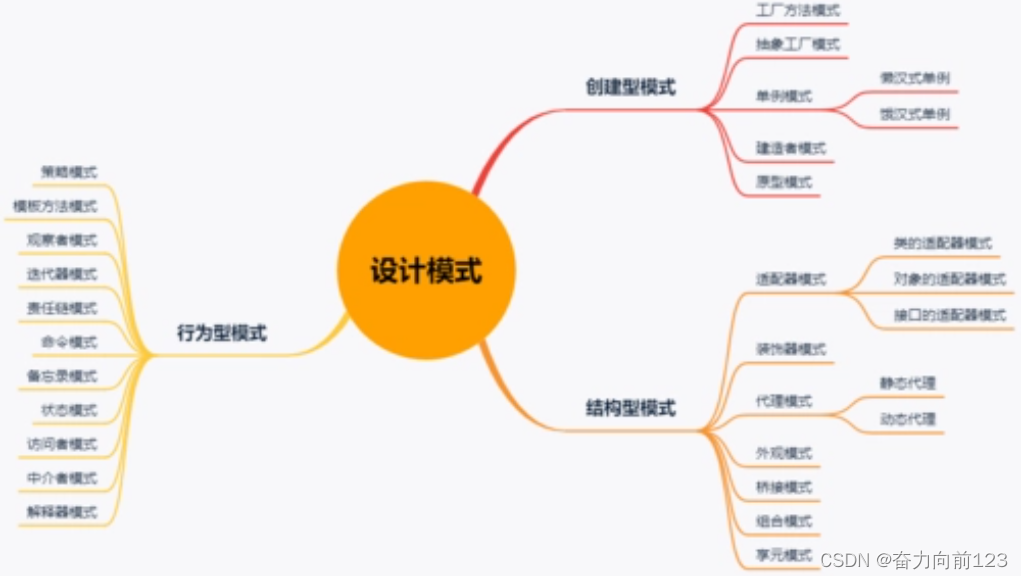
# 1. 设计模式的理论基础
设计模式是软件工程领域中解决常见问题的模板,它们是被广泛认可和使用的设计方法。理解设计模式的基础原理对于构建高质量、可维护和可扩展的软件至关重要。
## 1.1 设计模式的起源与发展
设计模式的概念源自Christopher Alexander在建筑领域的著作,而在软件工程中则由Erich Gamma、Richard Helm、Ralph Johnson和John Vlissides(通常被称为“四人帮”)在《设计模式:可复用面向对象软件的基础》一书中首次提出。
## 1.2 设计模式的分类
设计模式按照其用途和目的被分为三大类:创建型、结构型和行为型。每种类型下包含不同的模式,如单例模式属于创建型,策略模式属于行为型。
## 1.3 设计模式的原则
设计模式遵循SOLID原则,即单一职责、开闭原则、里氏替换、接口隔离以及依赖倒置。这些原则指导着设计模式的应用,保证软件的灵活性和可维护性。
设计模式不仅是一套规则,它更是一种思维方式,帮助开发者在面对复杂问题时,能够拥有清晰和一致的解决方案。在接下来的章节中,我们将深入探讨各类设计模式的具体应用和实践。
# 2. 创建型设计模式的应用与实践
在软件工程中,创建型设计模式提供了对象创建的优雅解决方案,能够提高代码的可维护性和灵活性。本章节将深入探讨几种常用的创建型设计模式,包括单例模式、工厂方法模式和抽象工厂模式,并分析它们在实际应用中的案例和优化策略。
## 2.1 单例模式
### 2.1.1 单例模式的定义与特点
单例模式是一种确保一个类仅有一个实例,并提供一个全局访问点来获取这个实例的设计模式。该模式涉及到一个单一的类,该类负责创建自己的唯一实例,并提供一个访问它的全局方法。
单例模式的主要特点包括:
- **全局访问**:类有一个全局访问点,通常是一个静态方法。
- **延迟初始化**:单例实例是在第一次被引用时才被创建。
- **线程安全**:在多线程环境下,需要保证单例的唯一性不受线程影响。
### 2.1.2 单例模式在框架中的实际应用
在实际的软件开发中,单例模式被广泛应用于框架和库中,以确保某些资源的唯一性。例如,在日志系统中,通常只需要一个日志记录器实例来进行日志的记录。
下面是一个简单的单例模式实现示例:
```java
public class Logger {
// 利用一个静态变量保存Logger实例
private static Logger instance;
// 私有化构造方法,阻止外部直接创建实例
private Logger() {}
// 提供一个静态方法来访问单例实例
public static Logger getInstance() {
if (instance == null) {
instance = new Logger();
}
return instance;
}
// 日志记录方法
public void log(String message) {
// 日志记录逻辑...
}
}
```
为了保证线程安全,可以采用双重检查锁定模式(Double-Checked Locking)来改进上面的代码:
```java
public class SingletonWithDoubleCheckedLocking {
private volatile static SingletonWithDoubleCheckedLocking instance;
private SingletonWithDoubleCheckedLocking() {}
public static SingletonWithDoubleCheckedLocking getInstance() {
if (instance == null) {
synchronized (SingletonWithDoubleCheckedLocking.class) {
if (instance == null) {
instance = new SingletonWithDoubleCheckedLocking();
}
}
}
return instance;
}
}
```
在上述代码中,我们使用了`volatile`关键字来确保实例的可见性,并通过双重检查来减少锁的开销。
## 2.2 工厂方法模式
### 2.2.1 工厂方法模式的原理与结构
工厂方法模式是一种创建型设计模式,它定义了一个创建对象的接口,但让子类决定实例化哪一个类。工厂方法把类的实例化推迟到子类中进行。
工厂方法模式主要包含以下参与者:
- **抽象工厂(Creator)**:声明工厂方法,用于返回产品类型的对象。
- **具体工厂(Concrete Creator)**:重写工厂方法,返回一个具体的产品实例。
- **抽象产品(Product)**:为构成产品族的基类或接口。
- **具体产品(Concrete Product)**:具体工厂的创建目标。
### 2.2.2 工厂方法模式在不同框架中的使用案例
在各种框架中,工厂方法模式用于封装对象的创建过程,使得创建对象和使用对象分离,增加了系统的可扩展性和灵活性。
以JDBC数据库连接为例,不同数据库厂商提供的驱动类就是通过工厂方法模式来创建和使用的:
```java
public interface Connection {
// 数据库连接的方法和属性定义...
}
public class MySQLConnection implements Connection {
// MySQL连接的具体实现...
}
public class OracleConnection implements Connection {
// Oracle连接的具体实现...
}
public class ConnectionFactory {
public static Connection getConnection(String driverName) throws ClassNotFoundException, SQLException {
Class.forName(driverName);
if(driverName.equals("com.mysql.jdbc.Driver")) {
return new MySQLConnection();
} else if(driverName.equals("oracle.jdbc.driver.OracleDriver")) {
return new OracleConnection();
}
return null;
}
}
```
在这个例子中,`ConnectionFactory` 类的 `getConnection` 方法就是一个工厂方法,根据不同的驱动名称创建不同的数据库连接对象。
## 2.3 抽象工厂模式
### 2.3.1 抽象工厂模式的概念与优势
抽象工厂模式是工厂方法模式的升级版本,在有多个业务品种、业务分类时,通过抽象工厂方法,可以产生出一系列相关的对象。抽象工厂模式为创建一组相关或相互依赖的对象提供了一个接口,而不需要指定它们具体的类。
抽象工厂模式的关键点在于:
- **封装接口**:提供一个创建一系列相关或相互依赖对象的接口,无需指定它们具体的类。
- **隔离变化**:将系统中的产品族与产品种类的依赖问题从具体实现中解耦。
- **系统扩展性**:增加新产品族容易,增加新的产品种类困难。
### 2.3.2 如何在框架构建中应用抽象工厂模式
在框架的构建中,抽象工厂模式常用于实现多个组件之间的一致性,比如界面组件、数据库访问组件等。
例如,在一个GUI框架中,可以使用抽象工厂模式来创建跨平台的UI组件:
```java
public interface SkinFactory {
Button createButton();
TextField createTextField();
// 其他组件的创建方法...
}
public class WindowsSkinFactory implements SkinFactory {
public Button createButton() {
// 创建Windows风格的按钮
}
public TextField createTextField() {
// 创建Windows风格的文本框
}
}
public class LinuxSkinFactory implements SkinFactory {
public Button createButton() {
// 创建Linux风格的按钮
}
public TextField createTextField() {
// 创建Linux风格的文本框
}
}
public class SkinFactoryProducer {
public static SkinFactory getFactory(String osType) {
if (osType.equalsIgnoreCase("WINDOWS")) {
return new WindowsSkinFactory();
} else if (osType.equalsIgnoreCase("LINUX")) {
return new LinuxSkinFactory();
}
return null;
}
}
```
在这个例子中,`SkinFactory` 是一个抽象工厂接口,它定义了创建UI组件的方法。`WindowsSkinFactory` 和 `LinuxSkinFactory` 是具体工厂实现,根据不同的操作系统类型创建相应风格的UI组件。通过抽象工厂模式,系统可以在不修改现有代码的情况下引入新的UI组件风格。
通过本章节的介绍,我们了解了创建型设计模式的几种形式,及其在框架设计中的应用和实践案例。在后续章节中,我们将继续探讨结构型和行为型设计模式,并深入了解它们在现代Java框架中的综合应用,以及设计模式的未来趋势与挑战。
# 3. 结构型设计模式的应用与实践
结构型设计模式涉及如何组合类和对象以获得更大的结构。在本章节中,我们将深入了解适配器模式、装饰器模式和代理模式这三种结构型设计模式,并探讨它们在框架整合、性能优化和功能增强方面的实际应用。
## 3.1 适配器模式
适配器模式是结构型设计模式之一,其目的是解决两个不兼容接口之间的通信问题。通过创建一个适配器,将一个类的接口转换成客户期望的另一个接口。
### 3.1.1 适配器模式的实现原理
适配器模式通过适配器接口将一个类的接口适配为另一个接口。该模式主要包含以下四个角色:
- **目标接口(Target)**:客户所期望的接口。
- **需要适配的类(Adaptee)**:一个已经存在的接口,但它的接口不符合客户的需求。
- **适配器类(Adapter)**:将适配的接口转换为所需的接口。
- **客户端(Client)**:与符合目标接口的对象交互。
适配器模式可以使用类适配器模式或对象适配器模式实现。类适配器模式使用多重继承来实现适配,而对象适配器模式则使用组合。
### 3.1.2 适配器模式在框架整合中的作用
适配器模式在框架整合中的应用非常广泛。例如,当需要将一个已存在的框架或者组件整合到新的系统中,而新系统所期望的接口与已存在的接口不一致时,适配器模式就显得尤为重要。
#### 示例代码:
```java
// 一个简单的示例,展示如何使用对象适配器模式
// 目标接口
public interface Target {
void request();
}
// 已存在的类
public class Adaptee {
public void specificRequest() {
System.out.println("Adaptee specific request method.");
}
}
// 适配器类
public class Adapter implements Target {
private Adaptee adaptee = new Adaptee();
@Override
public void request() {
adaptee.specificRequest();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Target target = new Adapter();
target.re
```
0
0
相关推荐
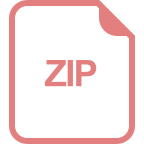
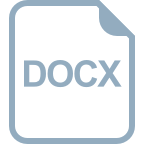
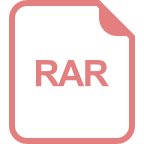
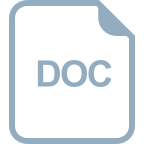
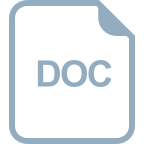
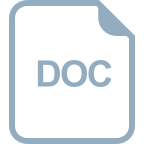
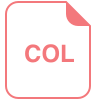
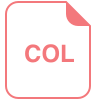
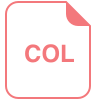