Java设计模式与软件重构
发布时间: 2024-08-30 06:53:16 阅读量: 62 订阅数: 34 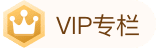
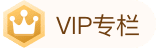
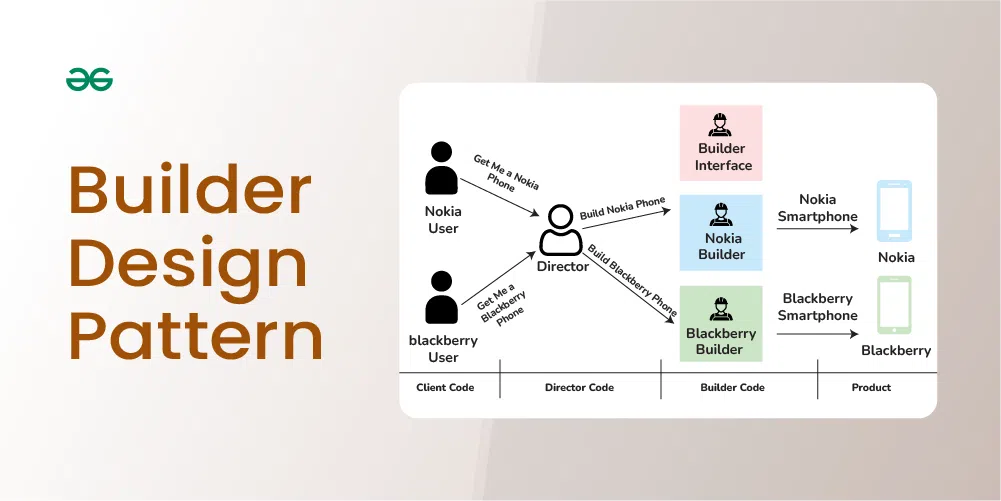
# 1. 设计模式概述
设计模式是软件工程中一种被广泛认可和应用的实践方法,它提供了一套被普遍接受的解决方案来处理在软件开发过程中重复出现的问题。设计模式不仅仅是一段代码,更是一种解决问题的思维模式。理解它们可以帮助我们以一种更加系统和标准化的方式来构建软件,从而提高代码的可读性、可维护性和扩展性。
## 1.1 设计模式的分类和重要性
设计模式被分为三类:创建型模式、结构型模式和行为型模式。创建型模式关注于对象的创建和初始化,结构型模式关注于对象间的组织和管理,而行为型模式则关注于对象间的交互和职责分配。
理解这些模式对于任何想要深入软件工程领域的开发者来说至关重要。它们不仅能够帮助我们避免重新发明轮子,还能帮助我们在设计软件时遵循最佳实践,这将大大减少项目风险并提升产品质量。
## 1.2 设计模式的学习路径
学习设计模式的路径可以分为理论学习、案例分析、实践应用和复盘优化四个阶段。理论学习涉及模式的定义、结构和用例;案例分析则侧重于如何在实际项目中应用这些模式;实践应用是通过实际编码来巩固理论知识;而复盘优化阶段则是回顾和评估所应用模式的效果,思考如何改进。
在设计模式的学习过程中,我们将会深入探讨和分析每个模式的内在机制,探索如何根据不同的应用场景选择和使用合适的设计模式,以及如何将这些模式有效地融入到现有的或新的软件架构中。通过这个过程,你将学会如何利用设计模式来解决复杂的编程问题,并提升软件架构的水平。
# 2. 创建型设计模式
创建型设计模式主要解决了对象创建的细节问题,使创建和使用对象的代码分离,以增加系统的灵活性和可复用性。这一章节将深入探讨单例模式、工厂方法模式和抽象工厂模式这三种创建型设计模式。
## 2.1 单例模式
### 2.1.1 单例模式理论基础
单例模式是一种常见的设计模式,确保一个类只有一个实例,并提供一个全局访问点。单例模式的特点包括:
- 单一职责:一个类只有一个责任,即创建它自己的唯一实例。
- 全局访问:无论何处需要,都可以通过全局访问点访问到这个唯一的实例。
- 控制实例化:控制了实例化的流程,避免产生多个实例。
在单例模式中,有两种常见的实现方式:懒汉式和饿汉式。
```java
// 懒汉式单例模式
public class SingletonLazy {
private static SingletonLazy instance = null;
private SingletonLazy() {}
public static SingletonLazy getInstance() {
if (instance == null) {
instance = new SingletonLazy();
}
return instance;
}
}
// 饿汉式单例模式
public class SingletonEager {
private static final SingletonEager instance = new SingletonEager();
private SingletonEager() {}
public static SingletonEager getInstance() {
return instance;
}
}
```
### 2.1.2 单例模式在实际中的应用
单例模式在实际开发中有很多应用场景,例如:
- 配置管理器:项目中可能存在多个配置文件,单例模式可以保证配置管理器实例的唯一性。
- 线程池:线程池的管理涉及到资源分配和线程的生命周期管理,使用单例模式能够确保线程池实例的唯一性。
- 日志记录器:日志记录器通常需要全局访问,使用单例模式可以确保日志记录的统一性和同步。
## 2.2 工厂方法模式
### 2.2.1 工厂方法模式概述
工厂方法模式是一种创建型设计模式,它定义了一个创建对象的接口,但让子类决定实例化哪一个类。工厂方法把类的实例化推迟到子类中进行。
```java
// 抽象产品
public abstract class Product {
}
// 具体产品A
public class ConcreteProductA extends Product {
}
// 具体产品B
public class ConcreteProductB extends Product {
}
// 抽象工厂
public abstract class Creator {
public abstract Product factoryMethod();
}
// 具体工厂A
public class ConcreteCreatorA extends Creator {
public Product factoryMethod() {
return new ConcreteProductA();
}
}
// 具体工厂B
public class ConcreteCreatorB extends Creator {
public Product factoryMethod() {
return new ConcreteProductB();
}
}
```
### 2.2.2 实践中的工厂方法模式
工厂方法模式在实际中的应用包括:
- 在系统扩展时,只需增加新的具体产品类和对应的工厂类即可,符合开闭原则。
- 当产品类有复杂的逻辑,且创建过程依赖于复杂的条件判断时,使用工厂方法模式可以将创建逻辑封装在工厂方法中,便于理解和维护。
## 2.3 抽象工厂模式
### 2.3.1 抽象工厂模式原理
抽象工厂模式是工厂方法模式的扩展,提供了一个接口,用于创建相关或依赖对象的家族,而不需要明确指定具体类。抽象工厂模式适合用于一个系统要独立于它的产品的创建、组合和表示时。
```java
// 抽象产品A
public interface AbstractProductA {
}
// 抽象产品B
public interface AbstractProductB {
}
// 具体产品A1
public class ProductA1 implements AbstractProductA {
}
// 具体产品A2
public class ProductA2 implements AbstractProductA {
}
// 具体产品B1
public class ProductB1 implements AbstractProductB {
}
// 具体产品B2
public class ProductB2 implements AbstractProductB {
}
// 抽象工厂
public interface AbstractFactory {
AbstractProductA createProductA();
AbstractProductB createProductB();
}
// 具体工厂1
public class ConcreteFactory1 implements AbstractFactory {
public AbstractProductA createProductA() {
return new ProductA1();
}
public AbstractProductB createProductB() {
return new ProductB1();
}
}
// 具体工厂2
public class ConcreteFactory2 implements AbstractFactory {
public AbstractProductA createProductA() {
return new ProductA2();
}
public AbstractProductB createProductB() {
return new ProductB2();
}
}
```
### 2.3.2 抽象工厂模式的实际运用
抽象工厂模式的实际应用包括:
- 在系统需要独立于它的产品的创建、组合和表示时。
- 当一个系统要提供多个产品系列中的产品对象,而系统只消费其中某一产品系列。
- 当需要提供一个产品类库,而只希望客户端依赖于抽象而非具体类时。
在了解了创建型设计模式的理论基础和实际应用后,下一章节将继续深入探讨结构型设计模式,并通过案例分析来强化理解和实践技巧。
# 3. 结构型设计模式
结构型设计模式关注如何将类或对象结合在一起,形成更大的结构。这些模式涉及一些技巧,如封装对象,以便我们可以灵活地将它们组合成复杂的系统。本章将探讨三种常用的结构型设计模式:适配器模式、装饰器模式和代理模式。
## 3.1 适配器模式
适配器模式允许我们使用一个接口与另一个接口兼容,解决不兼容接口间如何协同工作的问题。
### 3.1.1 适配器模式的定义与组成
适配器模式定义了一个对象,它封装了另一个对象,并通过将方法调用转换为另一种接口的调用来实现兼容。这种模式主要涉及以下角色:
- **目标接口(Target)**:客户端代码所期望的接口。
- **适配者类(Adaptee)**:一个已经存在并需要被适配的类。
- **适配器类(Adapter)**:用于将适配者接口转换为客户端所期望的接口的类。
适配器类可以实现目标接口,或者继承目标类,并在适配器类中封装一个适配者类的对象。
### 3.1.2 适配器模式在代码重构中的应用
在代码重构过程中,适配器模式可用于整合第三方库或者旧代码,从而避免对大量现有代码进行修改。
#### 实际案例分析
假设我们有一个第三方库提供的API,其接口不符合我们当前项目的需求,而我们又不希望或者无法修改原始库的代码。这时,我们可以创建一个适配器,实现我们期望的接口,并在内部使用第三方库。
```java
public class ThirdPartyLibrary {
public void legacyMethod() {
// 现有的第三方库方法
}
}
public interface Target {
void modernMethod();
}
public class Adapter implements Target {
private ThirdPartyLibrary adaptee = new ThirdPartyLibrary();
public void modernMethod() {
// 将现代接口适配为旧接口的方法
adaptee.legacyMethod();
}
}
```
在这个例子中,我们创建了一个`Adapter`类,它实现了我们期望的`Target`接口,并在`modernMethod`方法内部调用了第三方库的`legacyMethod`方法。这样,我们的新代码可以通过`Target`接口调用方法,而旧的第三方库代码不需要任何改动。
### 3.1.3 适配器模式的代码逻辑解读与参数说明
适配器模式的关键在于适配器类的实现。在上述Java代码中,`Adapter`类通过内部包装一个`ThirdPartyLibrary`对象,并将其转换为`Target`接口,实现了不同接口的适配。这种模式使得我们可以使用`Target`接口中的`modernMethod`方法,而不需要修改`ThirdPartyLibrary`类。
## 3.2 装饰器模式
装饰器模式通过给对象动态添加额外职责,而不改变对象本身。
### 3.2.1 装饰器模式的核心概念
装饰器模式允许用户将对象包装在装饰器中,以添加新的功能。装饰器模式涉及以下角色:
- **组件(Component)**:接口或抽象类,可以设置装饰者。
- **具体组件(Concrete Component)**:实现了组件接口的具体类。
- **装饰者(Decorator)**:维持一个组件对象的引用,并实现了与组件相同的接口。
- **具体装饰者(Concrete Decorator)**:具体的装饰者,实现了额外的行为。
### 3.2.2 装饰器模式的实践技巧
当需要向对象添加额外功能,而这些功能又需要动态地添加时,可以使用装饰器模式。装饰器模式提供了一个灵活的方式来增加对象的行为,而无需创建子类。
#### 实践案例
假设有一个图形界面组件库,我们要为其中的组件添加边框装饰,而不改变原有组件代码。
```java
public interface Component {
void draw();
}
public class ConcreteComponent implements Component {
public void draw() {
System.out.println("Drawing a concrete component.");
}
}
public abstract class Decorator implements Component {
protected Component component;
public Decorator(Component compone
```
0
0
相关推荐
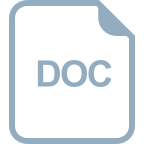
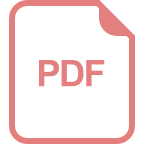
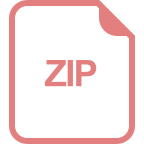





