Java设计模式在微服务架构中的角色
发布时间: 2024-08-30 06:45:33 阅读量: 132 订阅数: 45 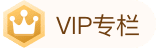
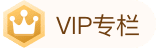
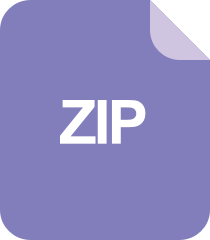
【Java设计模式】微服务API网关模式
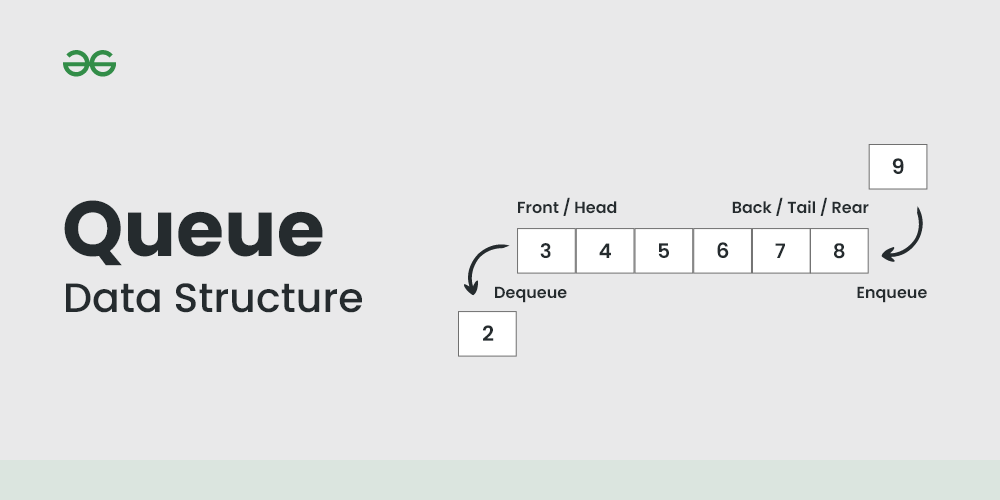
# 1. 微服务架构设计概述
微服务架构是现代软件开发中一种流行的方法论,它通过将复杂的应用程序分解为一组小的、独立的服务来提高开发的灵活性和可维护性。每一个微服务围绕特定的业务能力构建,并且可以独立开发、测试和部署。本章节将对微服务架构的概念进行基础性介绍,并探讨其与传统单体架构的不同,以及微服务架构设计中的核心原则和常见挑战。
## 微服务架构与传统单体架构对比
微服务架构与传统的单体架构在多个方面存在明显差异:
- **模块化与独立部署**: 微服务架构中的每个服务都是独立的,它们可以被独立部署和扩展,与之对比,单体架构的应用通常作为一个整体部署和管理。
- **技术栈的多样性**: 在微服务架构中,各个服务可以根据其特定需求选择最合适的编程语言、数据库和其他技术组件,而单体应用往往需要使用统一的技术栈。
- **复杂性管理**: 微服务通过将复杂性分解到各个服务中,使得每个团队可以集中精力处理特定服务的复杂性,从而降低整个系统的复杂性。单体架构则通常需要团队处理整个系统的复杂性,这可能导致“技术债务”。
在微服务架构设计中,需要特别注意服务之间的通信机制、服务发现、负载均衡、配置管理等问题。下一章节将深入探讨Java设计模式理论基础,并阐述如何在微服务架构中应用这些设计模式以解决实际问题。
# 2. Java设计模式理论基础
在软件工程中,设计模式是一套被反复使用、多数人知晓、经过分类编目、代码设计经验的总结。使用设计模式是为了可重用代码、让代码更容易被他人理解、保证代码可靠性。本章将详细探讨设计模式的分类、原理以及它们与面向对象设计原则之间的关系。
## 2.1 设计模式的分类和原理
设计模式通常被划分为三类:创建型模式、结构型模式和行为型模式。我们逐一探讨这些模式的内涵与应用场景。
### 2.1.1 创建型模式
创建型模式主要关注对象的创建过程,目的是使对象的创建独立于使用该对象的代码。常见的创建型模式有单例模式、工厂方法模式、抽象工厂模式、建造者模式、原型模式等。
```java
// 示例代码:单例模式的实现
public class Singleton {
// 使用私有构造函数和一个静态方法来实现单例模式
private static Singleton instance = null;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
在单例模式中,对象创建的过程通过一个静态方法封装,确保了全局只能创建一个实例。这是一种简单而有效的设计,常用于实现日志记录器、数据库连接池等。
### 2.1.2 结构型模式
结构型模式涉及如何组合类和对象以获得更大的结构。结构型模式主要包括适配器模式、桥接模式、组合模式、装饰模式、外观模式、享元模式和代理模式。
```java
// 示例代码:适配器模式的实现
public interface Target {
void request();
}
public class Adaptee {
public void specificRequest() {
System.out.println("具体请求由 Adaptee 实现");
}
}
public class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
public void request() {
adaptee.specificRequest();
}
}
```
适配器模式允许将一个类的接口转换成客户期望的另一个接口。它可以工作在类适配器和对象适配器模式中。适配器在系统升级和维护时非常有用,比如在不修改原有接口的情况下,增加新功能。
### 2.1.3 行为型模式
行为型模式关注对象之间的通信,涉及类或对象如何交互和分配职责。常见的行为型模式包括责任链模式、命令模式、解释器模式、迭代器模式、中介者模式、备忘录模式、观察者模式、状态模式、策略模式、模板方法模式和访问者模式。
```java
// 示例代码:观察者模式的实现
import java.util.ArrayList;
import java.util.List;
public interface Subject {
void registerObserver(Observer o);
void removeObserver(Observer o);
void notifyObservers();
}
public interface Observer {
void update(float temp, float humidity, float pressure);
}
public class WeatherData implements Subject {
private List<Observer> observers;
private float temperature;
private float humidity;
private float pressure;
public WeatherData() {
observers = new ArrayList<>();
}
public void measurementsChanged() {
notifyObservers();
}
public void setMeasurements(float temperature, float humidity, float pressure) {
this.temperature = temperature;
this.humidity = humidity;
this.pressure = pressure;
measurementsChanged();
}
public void registerObserver(Observer o) {
observers.add(o);
}
public void removeObserver(Observer o) {
int i = observers.indexOf(o);
if (i >= 0) {
observers.remove(i);
}
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(temperature, humidity, pressure);
}
}
}
```
在上述代码中,`Subject` 是被观察的对象,负责维护观察者列表,并在状态改变时通知所有观察者。`Observer` 是观察者的接口,定义了更新方法。这是一种灵活的设计,广泛应用于UI组件、事件处理系统等领域。
## 2.2 设计模式与面向对象设计原则
设计模式不是独立存在的,它们是面向对象设计原则的具体实现。理解这些原则有助于更好地应用设计模式。
### 2.2.1 SOLID原则简介
SOLID 是面向对象设计的五个基本原则的首字母缩写,由 Robert C. Martin 提出。这些原则有助于创建易于理解和维护的软件系统。
- 单一职责原则(SRP):一个类应该只有一个引起变更的原因。
- 开放/封闭原则(OCP):软件实体应该对扩展开放,对修改关闭。
- 里氏替换原则(LSP):子类型必须能够替换掉它们的父类型。
- 接口隔离原则(ISP):不应该强迫客户依赖于它们不用的方法。
- 依赖倒置原则(DIP):高层模块不应该依赖于低层模块,两者都应该依赖于抽象。
0
0
相关推荐
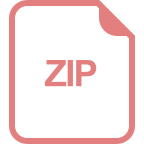
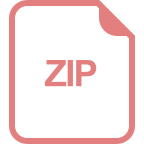
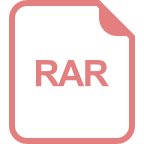
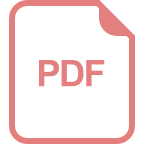
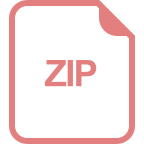
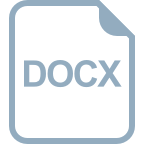
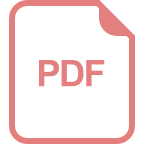
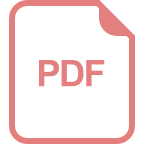