Java设计模式与并发编程
发布时间: 2024-08-30 06:20:02 阅读量: 137 订阅数: 45 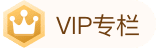
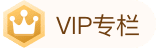
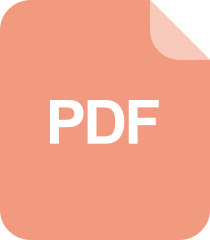
java 并发编程设计原则与模式第二版

# 1. Java设计模式与并发编程概述
## 1.1 设计模式与并发编程的交集
在现代软件开发中,Java设计模式与并发编程是两个极其重要的概念。设计模式提供了一组经过验证的解决方案,用于处理软件开发中常见的问题,而并发编程则涉及到在多线程环境下实现高效率与性能优化的编程技术。两者的结合,不仅能优化系统设计,还可以提升代码的可维护性和扩展性。
## 1.2 设计模式在并发环境中的价值
设计模式是软件工程中的最佳实践,它们能够帮助开发者以一种结构化和标准化的方式解决问题。在并发编程的上下文中,合理地运用设计模式能够有效管理线程间的交互,减少资源竞争和死锁的风险,从而提升应用的稳定性和性能。
## 1.3 并发编程的挑战与设计模式的作用
随着多核处理器的普及,多线程并发编程变得日益重要,但同时也带来了诸多挑战,如线程安全、死锁等问题。设计模式能够为这些问题提供框架级别的解决方案,使得并发代码更加清晰、易于理解和维护。例如,使用单例模式可以确保系统中某一资源的唯一性,而观察者模式可以灵活处理线程间的消息传递和事件驱动。
在下一章节,我们将深入探讨并发编程的基础理论,包括线程与进程的基本概念、同步机制与并发控制以及并发工具类的应用。了解这些基础知识是掌握设计模式在并发编程中应用的前提。
# 2. 并发编程基础理论
## 2.1 线程与进程的基本概念
### 2.1.1 线程和进程的定义及其区别
在操作系统中,进程是一个正在执行的程序的实例。它包含了一组用于执行程序指令的系统资源,包括代码、数据、内存、文件句柄和I/O设备。每个进程都有自己的地址空间,运行一个完全独立的程序。进程是资源分配的基本单位,由操作系统进行调度和管理。
线程是进程中的一个单一顺序控制流,是CPU调度和分派的基本单位。线程依赖于进程而存在,一个进程可以包含多个线程。线程之间共享进程资源,但拥有自己的调用栈和程序计数器。在多核处理器中,多个线程可以同时执行,提高了程序的并发性。
进程和线程的区别主要体现在以下几个方面:
- 资源分配:进程拥有独立的地址空间和资源,而线程共享进程的资源。
- 系统开销:进程切换比线程切换的开销要大,因为需要保存和恢复整个进程的状态。
- 通信机制:进程间通信(IPC)比较复杂,而线程间通信(如使用共享变量或队列)相对简单。
### 2.1.2 Java中的线程实现和管理
Java中的线程可以通过两种方式实现:继承Thread类或者实现Runnable接口。实现Runnable接口的方式更灵活,因为Java不支持多重继承,但可以实现多个接口。无论是哪种方式,最终都需要覆盖run()方法来定义线程要执行的任务。
```java
public class MyThread extends Thread {
@Override
public void run() {
System.out.println("MyThread is running.");
}
}
public class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("MyRunnable is running.");
}
}
```
为了管理线程,Java提供了Thread类的一些方法,比如start()用于启动线程,join()用于等待线程终止,interrupt()用于中断线程等。此外,通过Thread类的静态方法,如sleep(), yield()等,可以在多线程程序中实现线程调度和控制。
```java
public class ThreadManagement {
public static void main(String[] args) throws InterruptedException {
MyThread thread = new MyThread();
thread.start(); // 启动线程
MyRunnable runnable = new MyRunnable();
Thread thread2 = new Thread(runnable);
thread2.start();
thread.join(); // 等待线程结束
// 中断线程
thread2.interrupt();
// 线程休眠
Thread.sleep(1000);
}
}
```
## 2.2 同步机制与并发控制
### 2.2.1 同步块和同步方法
在多线程环境中,共享资源的访问可能导致数据不一致。Java提供了synchronized关键字来实现同步机制,以保证同一时间只有一个线程能够访问同步块或同步方法。
```java
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
```
在上面的例子中,increment()和getCount()方法都加上了synchronized关键字,确保了它们在多线程访问时的线程安全。
### 2.2.2 Locks和Condition接口
Java的java.util.concurrent.locks包提供了一个更灵活的同步机制——Locks。相比于synchronized关键字,Locks提供了更多高级功能,如尝试获取锁的非阻塞方式、可中断的锁获取操作等。Condition接口与Locks配合使用,提供了类似于Object监视器的等待/通知机制。
```java
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
import java.util.concurrent.locks.Condition;
public class ConditionExample {
private Lock lock = new ReentrantLock();
private Condition condition = lock.newCondition();
private boolean isReady = false;
public void await() {
lock.lock();
try {
while (!isReady) {
condition.await();
}
System.out.println("Thread is ready to continue.");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
lock.unlock();
}
}
public void signal() {
lock.lock();
try {
isReady = true;
condition.signalAll();
} finally {
lock.unlock();
}
}
}
```
### 2.2.3 线程间协作:wait/notify机制
wait/notify机制是Java对象监视器(monitor)的一部分,用于实现线程间的协作。当一个线程调用wait()方法时,它会释放当前对象的锁并等待,直到另一个线程调用同一个对象的notify()或notifyAll()方法。这两种方法必须在同步块或同步方法中调用。
```java
public class WaitNotifyExample {
private final Object lock = new Object();
private boolean isDone = false;
public void doWait() {
synchronized (lock) {
while (!isDone) {
try {
lock.wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
System.out.println("Continuing work...");
}
}
public void doNotify() {
synchronized (lock) {
isDone = true;
lock.notifyAll();
}
}
}
```
## 2.3 并发工具类的应用
### 2.3.1 线程池的原理及应用
线程池是一种池化技术,可以有效地管理线程资源,避免频繁创建和销毁线程带来的开销。Java中的Executor框架提供了一种灵活的方式来使用线程池。
```java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
System.out.println("Task is running.");
});
}
executor.shutdown();
try {
executor.awaitTermination(10, TimeUnit.SECONDS);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
```
### 2.3.2 并发集合框架
Java并发集合框架如ConcurrentHashMap、ConcurrentLinkedQueue等,是专为多线程环境设计的集合类,提供了更高的并发性能。它们通常使用更细粒度的锁或者无锁算法,减少线程竞争。
```java
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.putIfAbsent("key", 1);
System.out.println(map.get("key"));
}
}
```
### 2.3.3 原子变量与并发算法
原子变量如AtomicInteger、AtomicBoolean等,是使用底层硬件提供的原子性操作实现的线程安全变量。这些变量通过非阻塞算法来保证多线程环境下的原子操作,适用于需要细粒度并发控制的场景。
```java
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicIntegerExample {
private AtomicInteger atomicInteger = new AtomicInteger(0);
public void increment() {
atomicInteger.incrementAndGet();
}
public int getValue() {
return atomicInteger.get();
}
}
```
通过上述例子,我们了解了Java并发编程的基础理论,包括线程与进程的区别、同步机制和并发工具类的应用。这些理论是实现高效并发程序的基础。在接下来的章节中,我们将继续深入探讨并发编程在实际应用中的高级用法,以及并发编程与设计模式的结合。
# 3. Java设计模式基础
## 3.1 设计模式的分类和原则
设计模式是软件工程中用于解决常见问题的典型解决方案。它们提供了一套共有的词汇,让开发人员能够更容易地交流设计方案与经验。设计模式主要可以分为三类:创建型、结构型和行为型模式。理解这些模式和它们背后的设计原则是构建可维护和可扩展软件系统的基础。
### 3.1.1 创建型、结构型和行为型模式
创建型模式主要关注对象的创建过程,使得创建对象更加灵活和低耦合。结构型模式则关注对象和类的组合,以提供更丰富的结构,有助于组织代码和
0
0
相关推荐
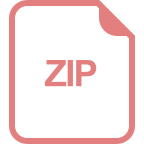
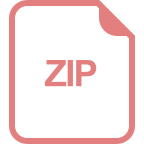
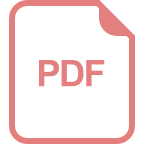
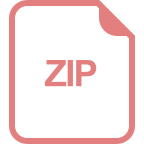
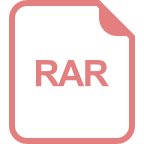