Java设计模式与系统设计
发布时间: 2024-08-30 06:49:09 阅读量: 90 订阅数: 34 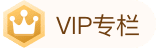
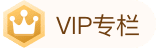
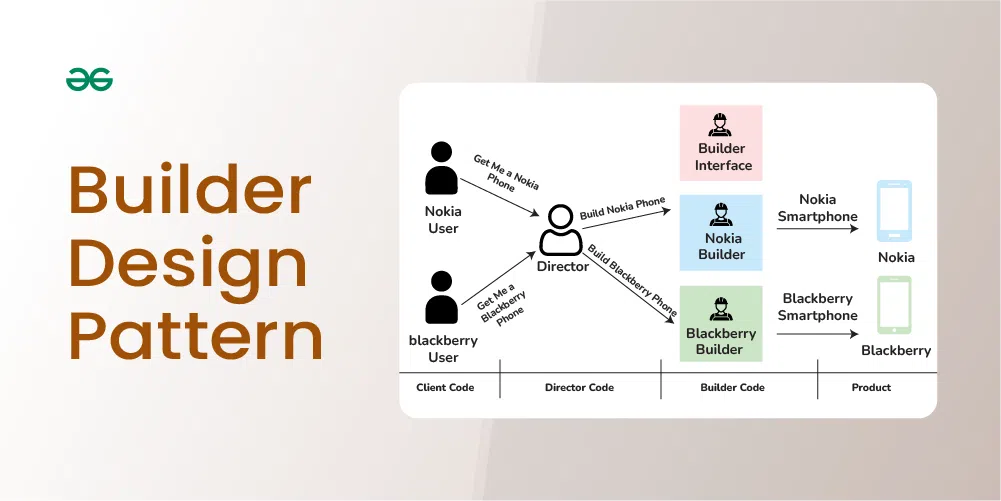
# 1. 设计模式与系统设计概述
设计模式是软件工程中一个重要的概念,它们是前人智慧的结晶,提供了一套经过验证的解决方案,用于应对软件开发中常见的设计问题。理解设计模式能够帮助开发者编写出更加灵活、可维护且易于扩展的代码。
系统设计则是将这些设计模式融入到实际项目中,构建出高效、稳定、易扩展的系统架构。设计模式不仅仅是一些固定模式,更多的是一种思维方式,通过对现有模式的深入理解,开发者可以创造出适合特定问题的解决方案。
设计模式与系统设计紧密相关,设计模式为系统设计提供了丰富的工具箱。掌握这些工具箱的关键在于理解它们的适用场景,以及如何将它们有效地应用于实际开发中。在后续章节中,我们将逐一探讨设计模式,并通过具体案例了解它们在系统设计中的应用。接下来,让我们深入探讨创建型设计模式,它是我们构建系统架构时的基石。
# 2. 创建型设计模式
创建型设计模式关注对象创建机制,帮助一个系统独立于如何创建、组合和表示其对象。这样的设计提升了代码的灵活性和可重用性。
## 2.1 单例模式
单例模式确保一个类只有一个实例,并提供一个全局访问点。
### 2.1.1 单例模式的定义与实现
单例模式的特点是类构造器的私有化,确保外部无法通过new创建对象实例。通常通过一个静态方法获取类的唯一实例。
```java
public class Singleton {
private static Singleton instance;
// 私有构造器防止通过new创建对象
private Singleton() {}
// 公有静态方法提供获取实例的方式
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
### 2.1.2 单例模式在系统设计中的应用
在需要控制实例数量的场景中,单例模式尤为重要,例如配置管理器或日志记录器。它可以确保整个应用中只有一个配置管理器实例,使得全局配置的修改能够即时反映在各部分中。
## 2.2 建造者模式
建造者模式是创建一个复杂对象的算法的抽象。一个构建过程涉及多个步骤,并且可以创建不同表示的对象。
### 2.2.1 建造者模式的原理和组成
建造者模式主要包含四个角色:产品、指导者、建造者接口和具体建造者。
```java
// 产品类
class Product {
// 产品由多个部件组成,此处省略部件的定义...
}
// 建造者接口
interface Builder {
void buildPartA();
void buildPartB();
Product getResult();
}
// 具体建造者
class ConcreteBuilder implements Builder {
private Product product = new Product();
public void buildPartA() {
// 构建产品的部件A
}
public void buildPartB() {
// 构建产品的部件B
}
public Product getResult() {
return product;
}
}
// 指导者
class Director {
public void construct(Builder builder) {
builder.buildPartA();
builder.buildPartB();
}
}
```
### 2.2.2 实际案例分析:建造者模式的实践
在UI组件的创建过程中,建造者模式可以用来一步一步地构建复杂的UI组件。例如,Android中AlertDialog的构建就是建造者模式的典型应用。
## 2.3 工厂方法模式
工厂方法模式定义了一个创建对象的接口,但由子类决定要实例化的类是哪一个。
### 2.3.1 工厂方法模式的核心概念
工厂方法模式通过定义一个用于创建对象的接口,让子类决定实例化哪一个类。工厂方法将创建对象的职责推迟到子类。
```java
// 抽象产品类
abstract class Product {
// 产品的方法...
}
// 具体产品类A
class ConcreteProductA extends Product {
// 实现产品的方法...
}
// 具体产品类B
class ConcreteProductB extends Product {
// 实现产品的方法...
}
// 抽象工厂类
abstract class Factory {
abstract Product createProduct();
}
// 具体工厂类A
class ConcreteFactoryA extends Factory {
Product createProduct() {
return new ConcreteProductA();
}
}
// 具体工厂类B
class ConcreteFactoryB extends Factory {
Product createProduct() {
return new ConcreteProductB();
}
}
```
### 2.3.2 系统设计中的工厂模式实例
在基于插件的系统中,工厂方法模式常用来创建不同类型的插件对象。例如,IDE(集成开发环境)允许通过插件扩展其功能,每个插件都可以有自己的工厂来创建相应的对象。
## 2.4 抽象工厂模式
抽象工厂模式提供一个接口,用于创建相关或依赖对象的家族,而不需要明确指定具体类。
### 2.4.1 抽象工厂模式的特点与结构
抽象工厂模式是一种创建型设计模式,用于创建一系列相关或相互依赖的对象,而无需指定它们具体的类。
```java
// 抽象产品族
interface AbstractProductA {
// 产品A的方法
}
interface AbstractProductB {
// 产品B的方法
}
// 具体产品A
class ConcreteProductA implements AbstractProductA {
// 实现产品A的方法
}
// 具体产品B
class ConcreteProductB implements AbstractProductB {
// 实现产品B的方法
}
// 抽象工厂
interface AbstractFactory {
AbstractProductA createProductA();
AbstractProductB createProductB();
}
// 具体工厂
class ConcreteFactory implements AbstractFactory {
public AbstractProductA createProductA() {
return new ConcreteProductA();
}
public AbstractProductB createProductB() {
return new ConcreteProductB();
}
}
```
### 2.4.2 应用抽象工厂模式解决复杂系统问题
在需要提供一个产品系列的环境中,抽象工厂模式可以大显身手。比如,一个UI库可能需要提供不同风格的组件,例如暗黑风格、明亮风格等,抽象工厂模式可以用来统一创建这些风格的组件,以保证一致性。
以上就是本章的详细内容,通过本章节的介绍,我们将创建型设计模式进行了全面的探讨。在下一章,我们将继续深入结构型设计模式的探讨。
# 3. 结构型设计模式
## 3.1 适配器模式
适配器模式是一种结构型设计模式,它允许将一个类的接口与另一个类的接口相匹配,即使这两个接口不兼容。适配器模式经常用于将遗留代码集成到新的系统中,或者在使用第三方库时需要适配已有的接口。
### 3.1.1 适配器模式的工作机制
适配器模式的主体思想是创建一个新的类,也就是适配器类,它实现了一个接口,该接口与目标接口兼容。适配器类内部包含一个需要被适配的类的实例,通过调用这个实例的方法,适配器类提供了与目标接口兼容的实现。
适配器模式的关键组件包括:
- **目标接口(Target)**:客户端需要实现的接口。
- **被适配者(Adaptee)**:拥有客户端不需要的接口方法的现有类。
- **适配器(Adapter)**:适配器类,它实现了目标接口,并通过内部的被适配者实例来调用被适配者的方法。
### 3.1.2 在系统集成中使用适配器模式
在系统集成过程中,适配器模式提供了一种优雅的方法来桥接不同组件之间的接口不兼容问题。例如,假设有一个第三方库提供了一套日志记录器接口,但是当前系统使用的是自己定义的日志接口。在这种情况下,可以创建一个适配器类来适配第三方库的日志接口到自己的接口,从而无缝集成第三方库。
代码实现适配器模式可能如下所示:
```java
// 目标接口
public interface Target {
void request();
}
// 被适配者接口
public class Adaptee {
public void specificRequest() {
System.out.println("Adaptee specific request");
}
}
// 适配器类
public class Adapter implements Target {
private Adaptee adaptee = new Adaptee();
@Override
public void request() {
adaptee.specificRequest();
}
}
// 客户端代码
public class Client {
public void execute(Target target) {
target.request();
}
}
// 测试代码
public class AdapterPatternTest {
public static void main(String[] args) {
Client client = new Client();
Target target = new Adapter();
client.execute(target); // 输出: Adaptee specific request
}
}
```
在上述代码中,`Adapter` 类实现了 `Target` 接口,并在 `request()` 方法中调用了 `Adaptee` 类的 `specificRequest()` 方法。客户端代码通过 `Target` 接口与 `Adapter` 类交互,无需直接与 `Adaptee` 接口打交道。
通过使用适配器模式,可以确保系统在不修改原有代码的基础上,通过增加适配器层来引入新的第三方库或组件。这不仅减少了代码的复杂性,也提高了系统的可维护性和可扩展性。
# 4. 行为型设计模式
## 4.1 观察者模式
### 4.1.1 观察者
0
0
相关推荐
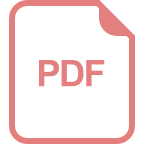
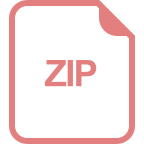
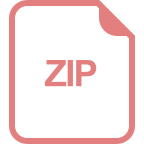





