Java设计模式与代码复用
发布时间: 2024-08-30 06:42:08 阅读量: 74 订阅数: 34 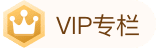
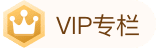
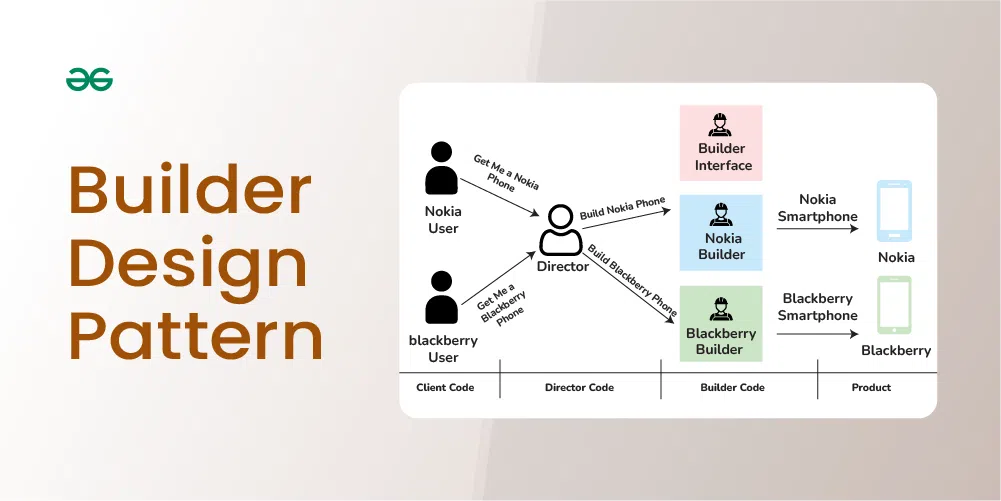
# 1. 设计模式概念与原则
设计模式是软件工程中用于解决常见问题的模板,它们代表了在特定上下文中经过时间检验的最佳实践。理解设计模式的核心概念及其背后的原则是成为高效软件开发者的关键。在本章中,我们将探究设计模式的基础知识,理解其为何对提升软件设计质量至关重要,并介绍SOLID原则,这些原则是应用设计模式的基石。通过掌握这些原则,读者能够更好地识别和应用各种设计模式,从而构建出更加健壮、易维护和可扩展的软件系统。
## 1.1 设计模式的目的和重要性
设计模式通过提供一种通用的解决特定问题的方法,帮助开发者减少重复工作,并促进代码重用。它们是经过反复实践并被广泛接受的解决方案,能够有效解决软件开发中的设计挑战。
## 1.2 设计模式的类型概述
设计模式大致可以分为三类:创建型、结构型和行为型。创建型模式主要涉及对象实例化过程中的问题解决,结构型模式关注对象和类的组合,而行为型模式则专注于对象之间的通信。
## 1.3 SOLID原则的基础
SOLID原则是设计模式遵循的核心理念。它们包括单一职责、开闭原则、里氏替换、接口隔离和依赖倒置,这些原则指导我们如何创建出更容易理解和维护的代码。
理解了第一章的内容,开发者将能够识别问题场景并准备好选择适当的设计模式来优化代码结构。接下来的章节将深入到各种设计模式的细节,包括实现技巧、最佳实践和案例分析,以进一步加深理解。
# 2. 创建型设计模式
### 2.1 单例模式的实现与应用
单例模式是一种常见的设计模式,它确保一个类只有一个实例,并提供一个全局访问点。在软件开发中,单例模式常用于管理资源,如数据库连接或日志对象。
#### 2.1.1 单例模式的基本概念
在单例模式中,类负责创建自己的唯一实例,并提供一个访问这个实例的全局访问点。这个类被称为单例类。单例模式的核心在于确保全局只有一个访问点,同时严格控制实例化的过程。
#### 2.1.2 单例模式的实现技巧
实现单例模式有多种方法,但它们都遵循相同的原则:确保类只能被实例化一次。
**懒汉式(线程不安全)**
```java
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
**懒汉式(线程安全)**
```java
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
**饿汉式**
```java
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
```
这些是实现单例模式的基本技巧。在选择实现方式时,需要考虑多线程环境下的安全性以及性能开销。
#### 2.1.3 实际案例分析
考虑一个日志管理系统的实现,日志对象通常需要全局访问,且实例化代价较高。使用单例模式可以确保日志系统只创建一个实例,同时避免多线程环境下的并发问题。
### 2.2 工厂模式的实现与应用
工厂模式是创建型设计模式中最常用的一种,它抽象了创建对象的过程,使得创建对象和使用对象的过程分离。
#### 2.2.1 工厂方法与抽象工厂
工厂方法模式通过定义一个创建对象的接口,让子类决定实例化哪一个类。抽象工厂模式则为一组相关或相互依赖的对象提供一个接口,而不需要指定它们具体的类。
#### 2.2.2 工厂模式的实践技巧
工厂模式有多个变体,如简单工厂、工厂方法和抽象工厂,每种变体适用于不同场景。例如,简单工厂适用于创建对象种类较少的情况;工厂方法适用于对象创建逻辑可以单独抽象出来的场景;抽象工厂适用于需要提供多个产品系列的场景。
#### 2.2.3 案例研究:灵活的依赖注入
在复杂的应用程序中,使用工厂模式可以实现依赖注入,从而提高系统的灵活性和可测试性。例如,在一个框架中,可以通过工厂方法注入具体的数据库连接对象。
### 2.3 建造者模式的实现与应用
建造者模式通过逐步构建复杂对象并提供一个建造者类,使得可以灵活地创建具有多个组件的不同表示的对象。
#### 2.3.1 建造者模式的适用场景
建造者模式适用于创建一个复杂对象,这个对象的创建过程需要多个步骤,且这些步骤彼此依赖或需要以特定顺序执行。
#### 2.3.2 实现链式调用的秘诀
链式调用是建造者模式的一个关键特性,它通过返回 `this` 关键字实现连续调用。在Java中,可以通过以下方式实现链式调用:
```java
public class ProductBuilder {
private String partA;
private String partB;
private String partC;
public ProductBuilder setPartA(String partA) {
this.partA = partA;
return this;
}
public ProductBuilder setPartB(String partB) {
this.partB = partB;
return this;
}
public ProductBuilder setPartC(String partC) {
this.partC = partC;
return this;
}
public Product build() {
// 在此处完成产品的构建逻辑
return new Product(partA, partB, partC);
}
}
```
#### 2.3.3 实战演示:复杂对象的构建过程
在实际项目中,如UI组件的构建,可以使用建造者模式来逐步配置组件的各个部分,然后一次性构建出一个完整的UI组件。
# 3. 结构型设计模式
结构型设计模式关注如何将对象和类组装成更大的结构,并保持这些结构的灵活和高效。本章将探讨适配器、装饰器和代理模式的实现与应用,分析这些模式如何在不同场景下解决具体问题,并介绍代码实现的关键点。
## 3.1 适配器模式的实现与应用
### 3.1.1 适配器模式的基本原理
适配器模式是一种结构型设计模式,它允许将一个类的接口转换成客户期望的另一个接口。这样原本因为接口不兼容而不能一起工作的类可以一起工作。适配器模式通过创建一个中间层(适配器)来协调两个不兼容接口之间的通信。
在实际应用中,适配器模式通常用于以下场景:
- 你想使用一个已存在的类,而其接口不符合你的需求。
- 你想创建一个可以复用的类,该类可以与其他不相关的类或不可预见的类协同工作。
- 你想使用多个已存在的子类,但不可能对每一个都进行子类化以匹配它们的接口。对象适配器可以适配它的父类接口。
### 3.1.2 类适配器与对象适配器的差异
适配器模式有两种实现方式:类适配器和对象适配器。
**类适配器**:通过多重继承对一个接口与另一个接口进行匹配。它依赖于语言的多重继承机制。
```java
// 类适配器示例
public class Adapter extends Adaptee implements Target {
@Override
public void request() {
// 转换逻辑
super.specificRequest();
}
}
```
**对象适配器**:通过组合一个对象来适配另一个对象的接口。
```java
// 对象适配器示例
public class Adapter implements Target {
private Adaptee adaptee = new Adaptee();
@Override
public void request() {
// 转换逻辑
adaptee.specificRequest();
}
}
```
### 3.1.3 解决遗留系统整合问题
适配器模式特别适合解决遗留系统整合问题。遗留系统通常有固定的接口,而新系统往往需要与这些系统交互。通过适配器可以为遗留系统的接口提供现代的接口封装,使得新系统能够轻松地与之交互。
#### 实际案例分析
假设我们需要将一个遗留的日志系统整合到一个现代的应用程序中,该遗留系统只能通过一个较老的日志格式进行输入。我们可以创建一个适配器来将现代日志消息转换为遗留系统所期望的格式。
```java
public class LegacyLoggerAdapter implements ModernLogger {
private LegacyLogger legacyLogger;
public LegacyLoggerAdapter(LegacyLogger legacyLogger) {
this.legacyLogger = legacyLogger;
}
@Override
public void log(String message) {
String formattedMessage = convertToLegacyFormat(message);
legacyLogger.log(formattedMessage);
}
private String convertToLegacyFormat(String message) {
// 将现代日志格式转换为遗留格式的逻辑
return "Legacy format: " + message;
}
}
```
以上代码中,`ModernLogger` 是新系统的日志接口,`LegacyLogger` 是遗留系统的日志接口。通过适配器 `LegacyLoggerAdapter`,我们可以实现两个系统的日志整合。
## 3.2 装饰器模式的实现与应用
### 3.2.1 装饰器模式与继承的对比
装饰器模式是一种结构型设计模式,允许向一个现有的对象添加新的功能,同时又不改变其结构
0
0
相关推荐
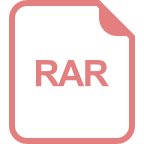
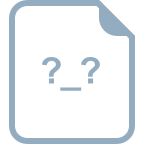
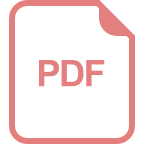
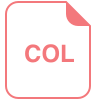
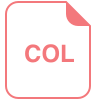
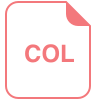
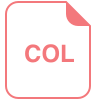

