Java设计模式精讲
发布时间: 2024-08-30 06:24:14 阅读量: 113 订阅数: 47 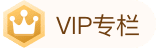
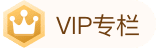
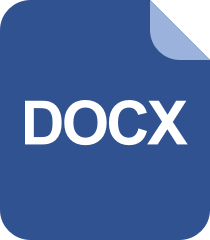
Java设计模式精讲1
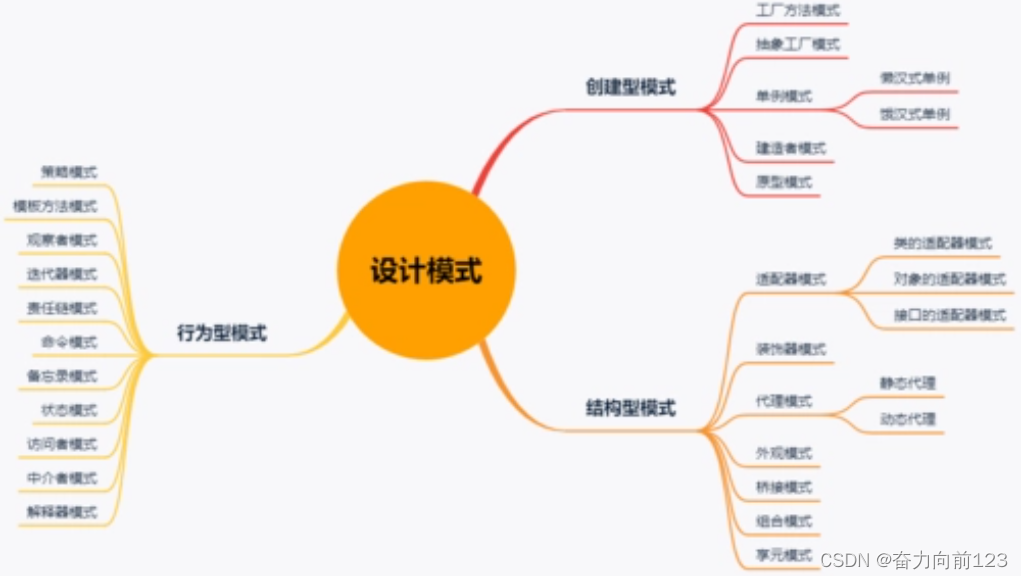
# 1. 设计模式概述
设计模式是软件工程中用于解决特定问题的一种公认、反复使用的设计方案。它是一套被反复使用、多数人知晓、经过分类编目、代码设计经验的总结,是软件设计中普遍适用的经验法则。本章将从设计模式的起源、分类和重要性三个维度进行初步探讨。
## 1.1 设计模式的起源
设计模式的概念最早由建筑设计师Christopher Alexander提出,他在其著作《A Pattern Language: Towns, Buildings, Construction》中,通过对建筑和城市规划的观察,总结出了一系列可复用的建筑模式。在软件工程领域,Erich Gamma、Richard Helm、Ralph Johnson和John Vlissides,也就是后来被誉为“四人帮(Gang of Four, GoF)”的学者们,在其著作《Design Patterns: Elements of Reusable Object-Oriented Software》中将设计模式引入,并将其系统化。
## 1.2 设计模式的分类
设计模式通常分为三类:创建型模式、结构型模式和行为型模式。创建型模式涉及对象实例化的过程,结构型模式关注类或对象组合的结构,行为型模式则关注对象之间的通信。
- **创建型模式**:提供了一种创建对象的最佳方式,比如单例模式、建造者模式、工厂方法模式等。
- **结构型模式**:处理类或对象的组合,比如适配器模式、装饰器模式、代理模式等。
- **行为型模式**:关注对象之间的职责划分,比如观察者模式、策略模式、模板方法模式等。
## 1.3 设计模式的重要性
设计模式对于软件开发人员而言具有极其重要的意义。首先,它们为解决常见问题提供了一组经过验证的模板。其次,设计模式能够帮助开发人员编写出更清晰、可维护、可扩展的代码。此外,通过熟悉这些模式,开发人员可以更容易地阅读和理解他人编写的代码,加速团队协作。
设计模式不仅有助于解决特定设计问题,而且还能促进软件架构的稳定性和适应性,进而提升软件的整体质量。在接下来的章节中,我们将深入探讨各种设计模式的具体实现、应用场景以及它们的优缺点。
# 2. 创建型模式
### 2.1 单例模式
#### 2.1.1 单例模式的概念和原则
单例模式(Singleton Pattern)是一种常用的软件设计模式,该模式的主要目的是确保一个类只有一个实例,并提供一个全局访问点来访问这个唯一的对象。单例模式通常用于管理配置信息,或者在创建对象需要大量资源时,如数据库连接。
单例模式有三个基本要点:
1. 单一职责:确保一个类只有一个对象被创建。
2. 全局访问点:类提供一个静态方法或属性来获取这个唯一的对象。
3. 延迟加载:实例化对象通常在第一次使用该对象时进行。
单例模式的原则是控制实例的创建,保证全局只有一个实例存在,并且提供一个全局访问点。
#### 2.1.2 单例模式的实现方式及应用实例
单例模式有多种实现方式,包括懒汉式、饿汉式、登记式等,但它们都遵循上述三个要点。
**饿汉式单例:**
```java
public class Singleton {
private static final Singleton instance = new Singleton();
// 私有构造函数,防止外部通过new创建对象
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
```
饿汉式单例在类加载时就完成了初始化,确保了对象的唯一性,但可能造成内存的浪费。
**懒汉式单例:**
```java
public class Singleton {
private static Singleton instance;
// 私有构造函数
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
懒汉式单例在第一次调用`getInstance()`时才初始化实例,节省资源。但需要注意线程安全问题。
#### 2.1.3 单例模式的优缺点和适用场景
**优点:**
1. 控制实例的创建,减少系统的内存开销。
2. 全局访问点使得外部调用更加方便。
**缺点:**
1. 单例模式违背了单一职责原则,扩展困难。
2. 如果使用不当,可能会引起序列化和反序列化的问题。
**适用场景:**
1. 创建对象需要占用大量的系统资源,如数据库连接池。
2. 需要定义大量的静态常量和静态方法(如工具类)。
3. 当一个类频繁地进行创建和销毁时,可以使用单例来避免资源的浪费。
### 2.2 建造者模式
#### 2.2.1 建造者模式的定义与结构
建造者模式(Builder Pattern)是一种创建型设计模式,它提供了一种创建对象的最佳方式。当一个对象的创建过程复杂,需要多个步骤和多个参数时,可以使用建造者模式。
建造者模式主要有以下几个角色:
1. **产品(Product)**:最终要创建的复杂对象。
2. **建造者(Builder)**:定义创建产品的接口。
3. **具体建造者(Concrete Builder)**:实现Builder接口,构建和装配各个部件。
4. **指挥者(Director)**:构建一个使用Builder接口的对象。
5. **客户端(Client)**:创建Director对象,并与之交互。
#### 2.2.2 建造者模式的实现步骤及实践案例
实现步骤:
1. 创建产品类。
2. 创建抽象建造者类和具体建造者类。
3. 创建指挥者类。
4. 客户端使用指挥者和建造者类来构建产品。
**实现示例:**
```java
// 产品类
class Product {
private String partA;
private String partB;
// ...
public void setPartA(String partA) { this.partA = partA; }
public void setPartB(String partB) { this.partB = partB; }
// ...
}
// 抽象建造者类
abstract class Builder {
protected Product product = new Product();
public abstract void buildPartA();
public abstract void buildPartB();
public Product build() {
return product;
}
}
// 具体建造者类
class ConcreteBuilder extends Builder {
@Override
public void buildPartA() {
// 组装partA
}
@Override
public void buildPartB() {
// 组装partB
}
}
// 指挥者类
class Director {
public void construct(Builder builder) {
builder.buildPartA();
builder.buildPartB();
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Director director = new Director();
Builder builder = new ConcreteBuilder();
director.construct(builder);
Product product = builder.build();
// 使用product对象
}
}
```
#### 2.2.3 建造者模式与其他模式的比较
建造者模式与其他创建型模式的比较主要在于它们的使用场景和构建方式:
- **与工厂方法模式的比较:**
工厂方法模式用于创建一个产品,而建造者模式用于创建一个复杂的产品,建造者模式更关注对象的组装过程。
- **与抽象工厂模式的比较:**
抽象工厂模式是为了创建一系列相关或相互依赖的对象,而建造者模式主要用于创建一个复杂的对象,侧重于创建过程。
- **与原型模式的比较:**
原型模式通过复制现有的对象来创建新的对象,而建造者模式是通过逐步构建一个复杂的对象来创建。
建造者模式通过不同的具体建造者来实现产品的构建,具有较高的灵活性和可扩展性,适用于创建过程复杂、需要逐步构建的产品。
# 3. 结构型模式
结构型模式涉及如何组合类和对象以获得更大的结构。本章节中,将对几种结构型模式进行深入探讨。适配器模式、装饰器模式、代理模式是结构型设计模式中常见的几种类型,它们各有千秋,解决不同类型的问题。
## 3.1 适配器模式
适配器模式是一种结构型设计模式,用来将一个类的接口转换成客户期望的另一个接口。适配器模式使得原本由于接口不兼容而不能一起工作的那些类可以一起工作。
### 3.1.1 适配器模式的适用背景和组成
适配器模式适用的背景包括以下几个方面:
- 当你需要使用一个已经存在的类,而其接口不符合你的需求时;
- 当你想要创建一个可以复用的类,该类可以与其他不相关的或未来引进的类一起工作时;
- 当你想要提供一个统一的接口来使用多个不同的接口时。
适配器模式通常由以下几个角色组成:
- 目标接口(Target):定义客户所需的接口;
- 适配者(Adaptee):包含一些有用的方法,但接口不符合Target;
- 适配器(Adapter):将Adaptee的接口转换成Target接口。
### 3.1.2 适配器模式的代码示例和具体应用
适配器模式的代码实现可以是类适配器模式或对象适配器模式。这里给出一个对象适配器模式的简单示例:
```java
// 目标接口
public interface Target {
void request();
}
// 适配者类
public class Adaptee {
public void specificRequest() {
System.out.println("Adaptee specific request method.");
}
}
// 适配器类
public class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void request() {
adaptee.specificRequest();
System.out.println("Adapter converts Adaptee's interface to Target's interface.");
}
}
// 客户端代码
public class Client {
public static void main(String[] args) {
Adaptee adaptee = new Adaptee();
Target adapter = new Adapter(adaptee);
adapter.request();
}
}
```
在实际应用中,适配器模式可以用于处理不同库或框架之间的接口兼容问题,或者当第三方库的接口更新但你无法修改原有代码时,通过适配器来兼容新的接口。
### 3.1.3 适配器模式在实际开发中的注意事项
适配器模式虽然非常有用,但是它也引入了一个间接的层,这可能会影响性能。因此,在系统中不宜大量使用适配器模式,尤其是当性能要求较高的系统中。同时,适配器模式也可能让系统更复杂,特别是适配器和适配者之间关系复杂时,会让整体设计变得难以理解和维护。
## 3.2 装饰器模式
装饰器模式允许向一个现有的对象添加新的功能,同时又不改变其结构。这种模式创建了一个装饰类,用来包装原有的类,并在保持类方法签名完整性的前提下,提供了额外的功能。
### 3.2.1 装饰器模式的目的和工作方式
装饰器模式的目的是动态地给一个对象添加一些额外的职责。它的行为保持了对象类的封装性和继承性,允许用户扩展对象的新功能。
工作方式是将对象放入一个包含行为的特殊封装类中,然后通过该封装类来调用原有对象的方法,并在此基础上增加新行为。
### 3.2.2 装饰器模式的具体实现和典型应用
装饰器模式的具体实现通常包括以下部分:
- 组件接口:定义一个对象接口,可以给这些对象动态地添加职责;
- 具体组件:实现了组件接口的具体对象;
- 装饰者:持有一个组件接口对象的引用,并实现与组件接口一致的接口;
- 具体装饰者:向组件添加职责。
一个简单的装饰器模式代码示例:
```java
// 组件接口
public interface Component {
void operation();
}
// 具体组件
public class ConcreteComponent implements Component {
@Override
public void operation() {
System.out.println("ConcreteComponent operation");
}
}
// 装饰者
public abstract class Decorator implements Component {
protected Component component;
public Decorator(Component component) {
***ponent = component;
}
@Override
public void operation() {
component.operation();
}
}
// 具体装饰者
public class ConcreteDecorator extends Decorator {
public ConcreteDecorator(Component component) {
super(component);
}
private void addedB
```
0
0
相关推荐







