【递归与迭代的较量】:倒插法排序实现效率与资源利用对比
发布时间: 2024-09-14 00:42:48 阅读量: 29 订阅数: 41 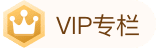
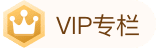
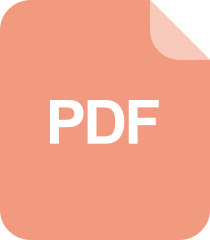
快速排序算法Python实现:详解分治法原理与高效排序步骤
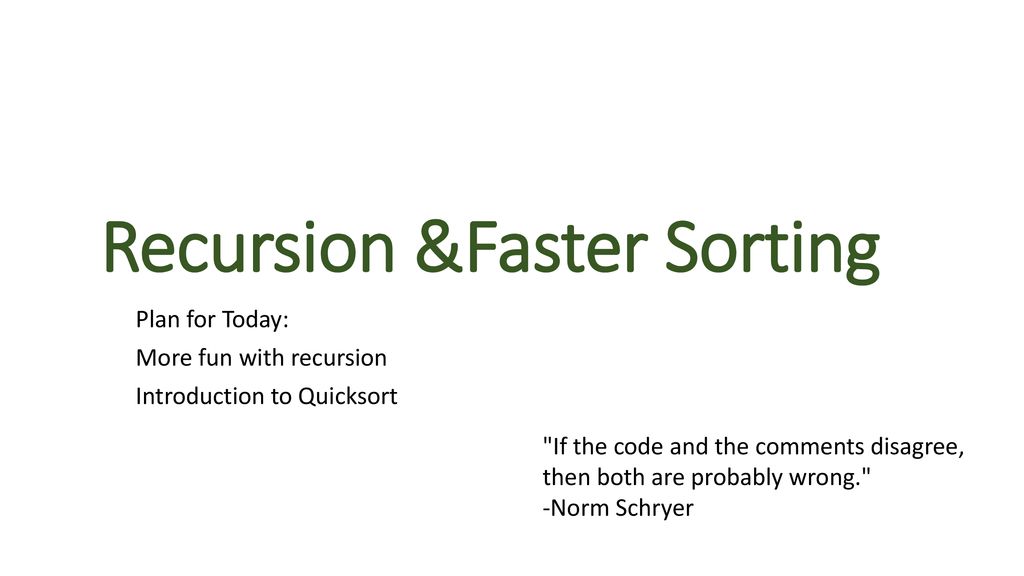
# 1. 倒插法排序概述
倒插法排序(Insertion Sort)是一种简单直观的排序算法。它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。此算法适宜于小规模数据集合,因为其算法简单,易于实现且效率较高。
## 算法理解
尽管倒插法排序在最坏情况下的时间复杂度为O(n^2),但它在部分数据几乎已经排序的情况下表现得非常高效。原因在于它对几乎已经有序的数据具有O(n)的时间复杂度,因此对于那些部分有序的中小型数据集来说,倒插法排序可能是更优的选择。
倒插法排序的基本步骤如下:
1. 从第一个元素开始,该元素可以认为已经被排序
2. 取出下一个元素,在已经排序的元素序列中从后向前扫描
3. 如果该元素(已排序)大于新元素,将该元素移到下一位置
4. 重复步骤3,直到找到已排序的元素小于或者等于新元素的位置
5. 将新元素插入到该位置后
6. 重复步骤2~5
## 应用场景
在实际的软件开发中,倒插法排序适用于以下场景:
- 数据量较少时的快速排序处理
- 部分有序的数组排序
- 实时系统中,数据到达后需要立即排序
在下一章中,我们将探讨递归的原理及其实现方式,它提供了一种不同的编程思路来理解和实现排序算法。
# 2. 递归原理及其实现
### 2.1 递归的基本概念
#### 2.1.1 递归定义和特点
递归是一种编程技术,它允许函数调用自身。这一过程可以继续,直到达到某种终止条件,然后控制权逐步返回到原始调用者。递归的关键在于它能够简化问题的复杂性,将大问题分解成小问题,直至达到简单直观的情况。
递归具有以下特点:
- **自引用**:函数调用自身。
- **基准情形(Base Case)**:解决最简单实例的代码,防止无限递归。
- **递归情形(Recursive Case)**:复杂问题调用自身以简化问题。
递归在算法设计中非常有用,特别是在处理具有自然层次结构或递归定义的问题时,例如树的遍历、分治算法和排序算法。
```python
def factorial(n):
# 基准情形
if n == 1:
return 1
# 递归情形
else:
return n * factorial(n - 1)
print(factorial(5)) # 输出 120
```
#### 2.1.2 递归的调用栈和内存消耗
每个递归调用都会在其所在的调用栈上创建一个新的帧,包含局部变量和返回地址。当递归深度很大时,会消耗大量内存,甚至导致栈溢出错误。
递归调用栈的工作原理如下:
- 当函数调用自身时,当前的局部变量和返回地址被压入栈中。
- 当函数返回时,栈顶的帧被弹出,控制权返回到前一个帧。
- 最后,只有最初的帧留在栈中,函数执行完成。
```mermaid
graph TD
A[开始调用函数factorial(5)] --> B[创建帧1]
B --> C[调用factorial(4)]
C --> D[创建帧2]
D --> E[调用factorial(3)]
E --> F[创建帧3]
F --> G[调用factorial(2)]
G --> H[创建帧4]
H --> I[调用factorial(1)]
I --> J[创建帧5]
J --> K[执行完毕,返回上一层]
K --> L[返回帧4]
L --> M[返回帧3]
M --> N[返回帧2]
N --> O[返回帧1]
O --> P[执行完毕]
```
### 2.2 递归式倒插法排序的实现
#### 2.2.1 算法描述与逻辑流程
递归式倒插法排序利用递归函数对数组进行分割和合并。以下是算法的基本步骤:
1. 将数组分成两半。
2. 递归调用排序函数,排序两个子数组。
3. 合并排序后的子数组。
以下是递归式倒插法排序的逻辑流程图:
```mermaid
graph TD
A[开始倒插法排序] --> B[分割数组]
B --> C[递归排序左子数组]
B --> D[递归排序右子数组]
C --> E[合并左子数组]
D --> F[合并右子数组]
E --> G[合并两排序好的子数组]
F --> G
G --> H[完成排序]
```
```python
def recursive_insertion_sort(arr, n):
if n <= 1:
return
else:
recursive_insertion_sort(arr, n - 1)
last = arr[n - 1]
j = n - 2
while j >= 0 and arr[j] > last:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = last
# 示例数组
arr = [12, 11, 13, 5, 6]
recursive_insertion_sort(arr, len(arr))
print(arr) # 输出已排序数组
```
#### 2.2.2 递归调用的优化技巧
递归实现的倒插法排序可以采用以下优化技巧:
- **尾递归优化**:尾递归是一种特殊的递归形式,当前函数的最后一次调用是自身。通过尾递归优化,编译器可以将递归转换为循环,节省栈空间。
- **迭代与递归结合**:当递归深度较大时,可以考虑将递归转换为迭代,以避免栈溢出。
```python
def recursive_insertion_sort(arr, n):
if n <= 1:
return
else:
recursive_insertion_sort(arr, n - 1)
last = arr[n - 1]
j = n - 2
while j >= 0 and arr[j] > last:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = last
```
### 2.3 递归实现的性能分析
#### 2.3.1 时间复杂度和空间复杂度
递归实现的倒插法排序的时间复杂度与传统的倒插法排序相同,为O(n^2),其中n为数组长度。每次插入操作最多涉及n-1次比较,而这样的插入操作会发生在整个数组长度减1次。
空间复杂度分析如下:
- 递归栈空间:递归深度为n,因此空间复杂度为O(n)。
- 辅助空间:由于是原地排序算法,不需要额外空间,空间复杂度为O(1)。
因此,递归实现的倒插法排序的空间复杂度为O(n)。
#### 2.3.2 实际运行时间的测试与比较
为了测试递归式倒插法排序的实际运行时间,可以编写一段代码来测量不同长度数组的排序时间。可以使用Python的`time`模块来测量时间。
```python
import time
# 测试函数
def test_sort(n):
arr = [i for i in range(n, 0, -1)]
start_t
```
0
0
相关推荐
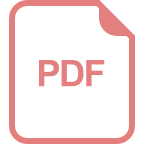
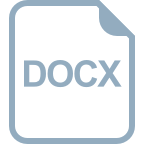
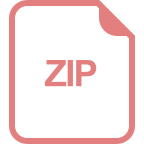
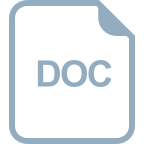
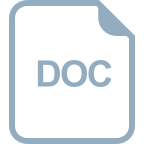
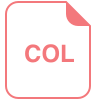
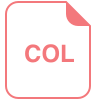
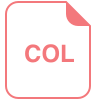