软件设计模式中的递归力量:策略模式与模板方法的递归实现
发布时间: 2024-09-12 20:20:43 阅读量: 80 订阅数: 29 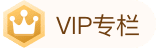
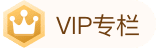
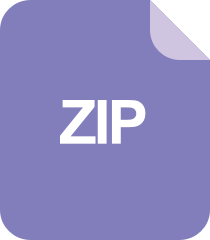
【Java设计模式-源码】好奇递归模板模式(CRTP):独特地利用多态性
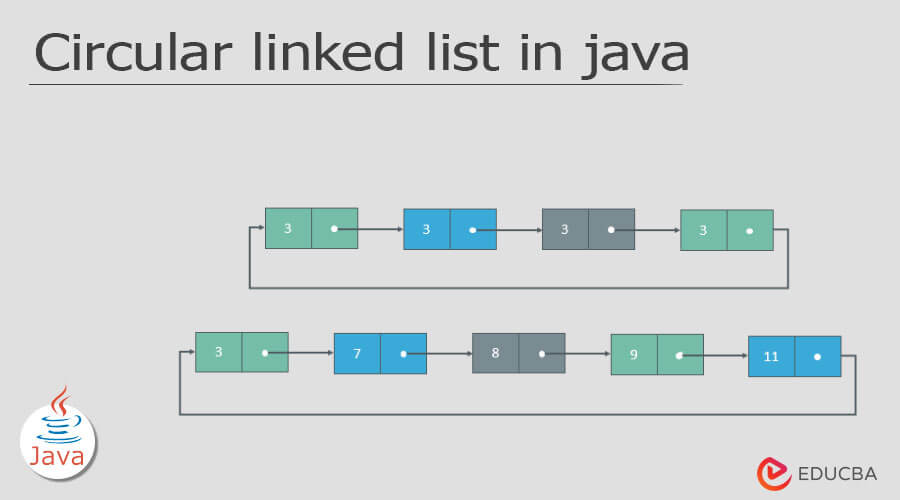
# 1. 递归思想的软件设计原则
递归作为编程和软件设计中一种重要的概念,其思想贯穿于许多设计模式和算法中。了解递归的核心原则,可以帮助开发者更好地利用递归解决复杂问题,并在软件设计中采用更优雅的解决方案。
递归思想的核心在于将大问题分解为小问题,并通过自我调用的方式解决问题。在软件设计中,递归原则促进了模块化和可复用性的提高。递归设计模式提供了处理可变行为和扩展性的新视角,使设计更加灵活和高效。
理解和掌握递归设计原则,不仅可以帮助开发者提高解决编程问题的能力,还能优化设计模式的实现,使得软件系统更加健壮和易于维护。接下来的章节将深入探讨递归设计模式的实现和应用。
# 2. 策略模式的基础与递归实现
## 2.1 策略模式的基本概念
### 2.1.1 策略模式的定义和结构
策略模式是一种行为设计模式,它定义了一系列算法,并将每一个算法封装起来,使它们可以互相替换,且算法的变化不会影响到使用算法的客户端。在软件设计中,策略模式是通过定义一系列的算法来实现一个行为,并将这些算法分别封装起来,使它们可以互换使用。策略模式让算法的变化独立于使用算法的客户端。
策略模式包含三个主要角色:
- **策略(Strategy)**:定义了算法的行为,它负责执行在上下文中指定的算法。
- **上下文(Context)**:持有对策略对象的引用,并负责调用在策略对象中定义的算法。
- **具体策略(ConcreteStrategy)**:实现了特定算法的类。
策略模式的结构图可以表示为:
```
+----------------+ +-----------------+
| <<Interface>> | | <<Interface>> |
| Strategy |<------| Context |
+----------------+ +-----------------+
^ |
| |
+-----------------+ +-----------------+
| ConcreteStrategy| | ConcreteStrategy|
| - implementation| | - implementation|
+-----------------+ +-----------------+
```
### 2.1.2 策略模式的适用场景
策略模式适用于以下场景:
- 有许多相关的类,仅仅是行为有差异的情况。
- 需要使用一个算法的不同变体。
- 算法需要在运行时选择不同的情况。
- 有多个行为,且在类族中这些行为以多个条件语句的形式出现,将它们转换为策略模式,将减少这些条件语句。
## 2.2 策略模式的递归实现
### 2.2.1 递归策略的定义和好处
递归策略是将策略模式应用于需要递归调用的算法。在递归策略模式中,策略接口通常包含一个实现递归算法的方法,这个方法会调用自身来实现递归逻辑。
递归策略的好处包括:
- **简化代码逻辑**:递归策略使得复杂的递归逻辑更加模块化,更易于理解和维护。
- **提高代码复用性**:同样的递归逻辑可以在不同的算法中重用,避免重复编码。
- **提升灵活性**:可以轻松地更改或添加新的递归算法,而不需要修改客户端代码。
### 2.2.2 递归策略在复杂场景中的应用
在复杂场景中,例如在树形结构数据的处理、图形渲染或者复杂的数据结构遍历等问题中,递归策略模式可以提供一个清晰且灵活的方式来实现复杂的递归逻辑。例如,在文件系统的遍历过程中,可以使用递归策略来遍历文件夹及其子文件夹。
## 2.3 策略模式的案例分析
### 2.3.1 策略模式的经典案例解读
考虑一个经典的排序问题,我们有多种排序算法如快速排序、归并排序和冒泡排序等。这些算法可以作为策略模式中的具体策略,而排序的上下文则负责调用这些算法。这样,当排序策略需要改变时(例如,从快速排序转向归并排序),只需更改上下文中的策略引用即可,而无需修改排序逻辑。
### 2.3.2 递归策略的实际编程演练
以归并排序为例,我们可以定义一个策略接口,包含一个递归合并排序的方法:
```java
public interface SortingStrategy {
void sort(int[] array);
}
public class MergeSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
if (array.length <= 1) return;
int mid = array.length / 2;
int[] left = new int[mid];
int[] right = new int[array.length - mid];
System.arraycopy(array, 0, left, 0, mid);
System.arraycopy(array, mid, right, 0, array.length - mid);
sort(left);
sort(right);
merge(array, left, right);
}
private void merge(int[] result, int[] left, int[] right) {
int i = 0, j = 0, k = 0;
while (i < left.length && j < right.length) {
if (left[i] <= right[j]) {
result[k++] = left[i++];
} else {
result[k++] = right[j++];
}
}
while (i < left.length) {
result[k++] = left[i++];
}
while (j < right.length) {
result[k++] = right[j++];
}
}
}
public class Context {
private SortingStrategy strategy;
public Context(SortingStrategy strategy) {
this.strategy = strategy;
}
public void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
public void executeStrategy(int[] array) {
strategy.sort(array);
}
}
```
在这个例子中,`MergeSortStrategy` 是一个具体策略,实现了归并排序算法,并且使用了递归逻辑来拆分数组,然后合并排序好的子数组。`Context` 类用来封装排序的上下文,调用具体的策略。这样,如果我们想使用不同的排序算法,只需要更换 `Context` 中的策略即可。
```java
int[] array = {3, 6, 2, 5, 1, 8};
Context context = new Context(new MergeSortStrategy());
context.executeStrategy(array);
// 执行结果将会是数组排序后的新状态。
```
接下来我们继续进入下一章:模板方法模式的基础与递归实现。
# 3. 模板方法模式的基础与递归实现
在软件设计领域中,模板方法模式是行为型设计模式之一,它定义了操作中的算法骨架,将一些步骤延迟到子类中。模板方法使得子类可以在不改变算法结构的情况下,重新定义算法中的某些特定步骤。本章节将深入探讨模板方法模式的基础知识及其递归实现方式,并通过案例分析展示其在实际编程中的应用。
## 3.1
0
0
相关推荐
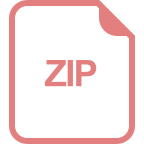
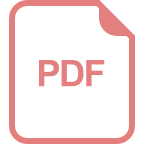
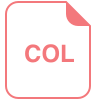
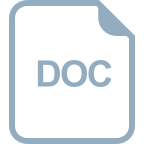
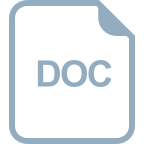
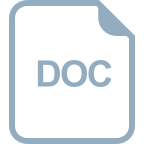
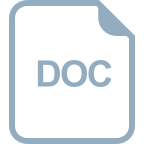
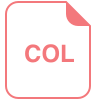