c++设计求n!(n为正整数)的非递归算法和递归算法
时间: 2024-09-24 22:04:57 浏览: 65
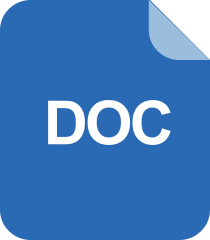
算法设计与分析实验一分治与递归
在 C++ 中,计算阶乘(n!)可以采用递归算法和非递归算法。
**非递归算法(也称为迭代法)**:
```cpp
#include <iostream>
using namespace std;
long long factorial(int n) {
if (n == 0 || n == 1)
return 1; // 阶乘的基本情况
long long result = 1;
for (int i = 2; i <= n; ++i) {
result *= i;
}
return result;
}
int main() {
int n;
cout << "Enter a positive integer: ";
cin >> n;
cout << "Factorial of " << n << " is: " << factorial(n);
return 0;
}
```
这个算法通过循环从1乘到n,逐步累积结果,直到n=1的情况。
**递归算法**:
```cpp
#include <iostream>
using namespace std;
long long factorial(int n) {
if (n == 0 || n == 1)
return 1; // 递归的基础情况
else
return n * factorial(n - 1); // 递归调用自身,每次减小n
}
int main() {
int n;
cout << "Enter a positive integer: ";
cin >> n;
cout << "Factorial of " << n << " is: " << factorial(n);
return 0;
}
```
在这个算法中,函数不断调用自身,直到达到基本情况才返回值。
阅读全文
相关推荐
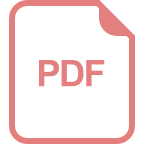
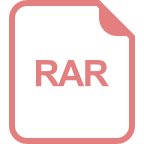




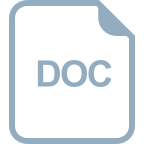
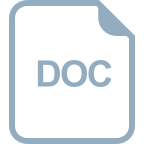









