c语言用递归方法求n阶勒让德多项式的值,其公式为 fn(x)=1 (n二0) fn(x) fn(x)=× (n = 1) fn(x) =((2n-1)x - fn-1(x) - (n-1)fn-2(x))/n (n>1)
时间: 2024-11-15 21:33:53 浏览: 10
在C语言中,我们可以使用递归的方法来计算n阶勒让德多项式(Legendre Polynomials)的值。首先,我们需要定义一个函数`legendre_poly()`,根据给定的公式逐步计算。
```c
#include <stdio.h>
// 定义一个double类型的数组来存储前两个值,因为它们不需要计算
double legendre_cache[2] = {1, 1}; // f0(x) 和 f1(x)
// 递归函数计算勒让德多项式
double legendre_poly(int n, double x) {
if (n == 0 || n == 1) {
return legendre_cache[n]; // 基本情况,直接从缓存中返回
} else {
double numerator = (2 * n - 1) * x;
double denominator = n;
// 根据公式计算当前项,考虑到f_n-1(x)和f_n-2(x),如果n > 2,需要从缓存中获取
double fn_minus_1 = n == 2 ? legendre_cache[1] : legendre_poly(n - 1, x);
double fn_minus_2 = n >= 3 ? legendre_poly(n - 2, x) : 0; // 如果n=2,fn-2(x)为0
return (numerator - fn_minus_1 - (n - 1) * fn_minus_2) / denominator;
}
}
int main() {
int n;
double x;
printf("Enter the degree of the polynomial and the value of x: ");
scanf("%d %lf", &n, &x);
if (n >= 0) {
double result = legendre_poly(n, x);
printf("The value of P_%d(x) at x = %.2lf is %.2lf\n", n, x, result);
} else {
printf("Invalid input for n. Please enter a non-negative integer.\n");
}
return 0;
}
```
在这个程序中,我们维护了一个缓存数组`legendre_cache`来存储已经计算过的低阶勒让德多项式,避免重复计算。用户输入n和x后,调用`legendre_poly()`函数并打印结果。
如果你对某个点的勒让德多项式的计算有疑问,或者想了解如何处理其他特殊情况,请告诉我。
阅读全文
相关推荐
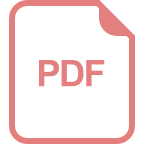
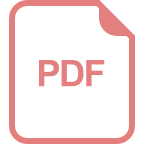
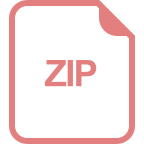















