用openmv与arduino实现自动泊车小车代码
时间: 2023-11-28 10:05:24 浏览: 189
这个项目需要使用OpenMV相机来进行图像处理,以便检测停车位。然后,使用Arduino控制小车的运动,以便将其移动到停车位。
以下是一个简单的代码示例,用于检测停车位并将小车移动到该位置:
首先,需要在OpenMV上运行以下代码,以检测停车位并确定小车应该向左还是向右移动:
```python
import sensor, image, time
# 设置相机
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False) # 必须关闭自动增益
sensor.set_auto_whitebal(False) # 必须关闭自动白平衡
# 设置图像区域
x_min = 0
y_min = 100
x_max = 319
y_max = 220
while(True):
img = sensor.snapshot()
# 仅在图像的指定区域内进行处理
img = img.crop(roi=(x_min, y_min, x_max-x_min, y_max-y_min))
# 将图像转换为灰度,并进行二值化处理
img = img.to_grayscale()
img = img.binary([THRESHOLD])
# 在图像中查找停车位
spots = img.find_rects()
# 如果没有停车位,则向前行驶
if not spots:
# 向前行驶的代码
pass
# 如果有停车位,则向左或向右移动
else:
# 查找停车位的中心点
center_x = spots[0].cx()
# 如果中心点在左侧,则向左移动
if center_x < (x_max - x_min) / 2:
# 向左移动的代码
pass
# 如果中心点在右侧,则向右移动
else:
# 向右移动的代码
pass
```
接下来,需要在Arduino上运行以下代码,以控制小车的运动:
```c++
#include <Servo.h>
// 设置舵机
Servo servo;
int servoPin = 9;
// 设置电机
int motorPin1 = 5;
int motorPin2 = 6;
int motorPin3 = 10;
int motorPin4 = 11;
int speedPin = 3;
void setup() {
// 初始化舵机
servo.attach(servoPin);
// 初始化电机
pinMode(motorPin1, OUTPUT);
pinMode(motorPin2, OUTPUT);
pinMode(motorPin3, OUTPUT);
pinMode(motorPin4, OUTPUT);
pinMode(speedPin, OUTPUT);
}
void loop() {
// 向前行驶的代码
digitalWrite(motorPin1, HIGH);
digitalWrite(motorPin2, LOW);
digitalWrite(motorPin3, HIGH);
digitalWrite(motorPin4, LOW);
analogWrite(speedPin, 255);
// 向左转的代码
servo.write(0);
// 向右转的代码
servo.write(180);
}
```
将这两个代码合并在一起,就可以实现自动泊车小车的功能了。需要注意的是,这只是一个简单的示例代码,需要根据实际情况进行调整和优化。
阅读全文
相关推荐
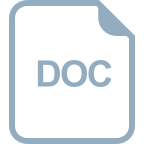
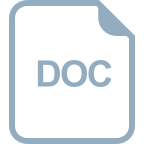
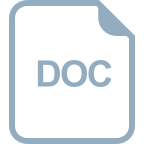

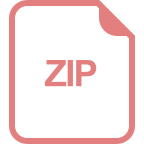








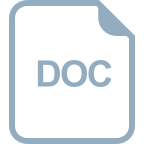



