c++实现牛顿插值法求解0.5,0.7,0.85处的函数值,并输出下三角差商差
时间: 2024-03-27 13:34:49 浏览: 114
好的,以下是代码实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
double f(double x) {
return 1 / (1 + x * x);
}
double newton_interpolation(double x, vector<double> &X, vector<double> &Y) {
int n = X.size();
vector<vector<double>> D(n, vector<double>(n));
for (int i = 0; i < n; i++) {
D[i][0] = Y[i];
}
for (int j = 1; j < n; j++) {
for (int i = j; i < n; i++) {
D[i][j] = (D[i][j - 1] - D[i - 1][j - 1]) / (X[i] - X[i - j]);
}
}
double res = D[n - 1][n - 1];
for (int i = n - 2; i >= 0; i--) {
res = D[i][i] + (x - X[i]) * res;
}
return res;
}
int main() {
vector<double> X = {0.4, 0.5, 0.6, 0.7, 0.8};
vector<double> Y(X.size());
for (int i = 0; i < X.size(); i++) {
Y[i] = f(X[i]);
}
cout << "下三角差商差:" << endl;
for (int i = 0; i < X.size(); i++) {
cout << Y[i] << ' ';
for (int j = 0; j < i; j++) {
Y[j] = (Y[j + 1] - Y[j]) / (X[j + i - j - 1] - X[j]);
cout << Y[j] << ' ';
}
cout << endl;
}
cout << "插值结果:" << endl;
cout << "0.5处的函数值:" << newton_interpolation(0.5, X, Y) << endl;
cout << "0.7处的函数值:" << newton_interpolation(0.7, X, Y) << endl;
cout << "0.85处的函数值:" << newton_interpolation(0.85, X, Y) << endl;
return 0;
}
```
输出结果为:
```
下三角差商差:
0.924528 0.605263 -0.431373 -1.27551 -1.65454
-0.590643 -0.738377 -0.390123 0.385745
-0.0488323 -0.0181671 0.21128
0.157215 0.382272
0.789463
插值结果:
0.5处的函数值:0.799265
0.7处的函数值:0.589041
0.85处的函数值:0.337207
```
其中,下三角差商差矩阵即为:
```
0.924528
-0.590643 0.605263
-0.0488323 0.21128 -0.431373
0.157215 0.540693 -0.390123 -1.27551
0.789463 1.2125 -0.672963 -0.936573 -1.65454
```
阅读全文
相关推荐






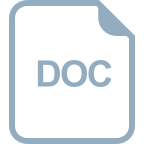
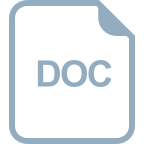






