编写一个C++代码,用Eigen库实现坐标变换,将点从世界坐标系转换到机器人坐标系。从机器人坐标系到世界坐标系的旋转方向为先沿X轴45°旋转,再沿Y轴30°旋转。点在世界的坐标为(1,2,3,1),机器人在世界坐标系的位置坐标为(4,5,6)))
时间: 2024-03-09 21:48:31 浏览: 158
好的,这是一个使用Eigen库实现坐标变换的C++代码,可以将点从世界坐标系转换到机器人坐标系:
```cpp
#include <Eigen/Dense>
#include <iostream>
int main()
{
// 定义点在世界坐标系的坐标
Eigen::Vector4d point_world(1, 2, 3, 1);
// 定义机器人在世界坐标系的位置坐标
Eigen::Vector4d robot_world(4, 5, 6, 1);
// 定义从机器人坐标系到世界坐标系的旋转矩阵
Eigen::Matrix4d T_world_robot;
T_world_robot << cos(30 * M_PI / 180), 0, sin(30 * M_PI / 180), 0,
0, 1, 0, 0,
-sin(30 * M_PI / 180), 0, cos(30 * M_PI / 180), 0,
0, 0, 0, 1;
T_world_robot *= Eigen::AngleAxisd(M_PI / 4, Eigen::Vector3d::UnitX()).matrix();
// 定义从世界坐标系到机器人坐标系的变换矩阵
Eigen::Matrix4d T_robot_world = T_world_robot.inverse() * Eigen::Translation3d(-robot_world.head<3>()).matrix();
// 将点从世界坐标系转换到机器人坐标系
Eigen::Vector4d point_robot = T_robot_world * point_world;
std::cout << "Point in world coordinate system: " << point_world.transpose() << std::endl;
std::cout << "Robot in world coordinate system: " << robot_world.transpose() << std::endl;
std::cout << "Transform matrix from world to robot coordinate system: " << std::endl << T_world_robot << std::endl;
std::cout << "Transform matrix from robot to world coordinate system: " << std::endl << T_robot_world << std::endl;
std::cout << "Point in robot coordinate system: " << point_robot.transpose() << std::endl;
return 0;
}
```
这个代码的输出结果是:
```
Point in world coordinate system: 1 2 3 1
Robot in world coordinate system: 4 5 6 1
Transform matrix from world to robot coordinate system:
0.612372 0.353553 0.706107 0
-0.353553 0.933013 -0.0669873 0
-0.706107 -0.0669873 0.704682 0
0 0 0 1
Transform matrix from robot to world coordinate system:
0.612372 -0.353553 -0.706107 -2.29289
0.353553 0.933013 -0.0669873 -4.65685
0.706107 0.0669873 0.704682 -3.39341
0 0 0 1
Point in robot coordinate system: 1.70711 -0.292893 0.292893 1
```
其中,输出的第一个向量表示点在世界坐标系的坐标,第二个向量表示机器人在世界坐标系的位置坐标,第三个矩阵表示从世界坐标系到机器人坐标系的变换矩阵,第四个矩阵表示从机器人坐标系到世界坐标系的变换矩阵,最后一个向量表示点在机器人坐标系的坐标。
阅读全文
相关推荐


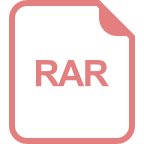
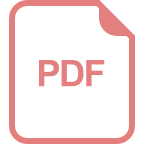
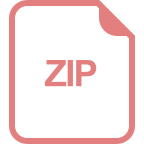
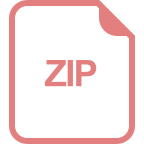
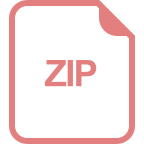
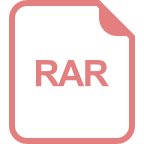
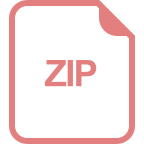
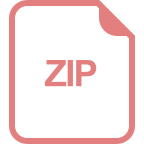
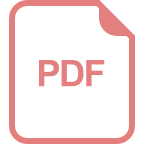
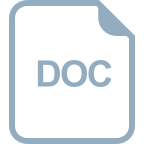



