已知直线1起点坐标x1,y1,终点坐标x2,y2,直线2起点坐标x3,y3,终点坐标x4,y4,使用C#编程求直线1直线2的交点,
时间: 2024-09-15 08:08:14 浏览: 59

已知起点和终点的坐标,切线角和曲率,求解五次多项式的C++源码
在C#中,你可以使用两点间距离公式以及两条直线平行的条件来找出两直线的交点。如果这两条直线不平行,则它们有一个唯一的交点。以下是基本步骤的实现:
```csharp
using System;
public class Point
{
public double X { get; set; }
public double Y { get; set; }
public Point(double x, double y)
{
X = x;
Y = y;
}
// 计算两点之间的距离
public double Distance(Point other)
{
return Math.Sqrt(Math.Pow(this.X - other.X, 2) + Math.Pow(this.Y - other.Y, 2));
}
}
public class Line
{
private Point _start;
private Point _end;
public Line(Point start, Point end)
{
_start = start;
_end = end;
}
public Point FindIntersectionWith(Line other)
{
if (IsParallel(other))
return null; // 直线平行无交点
// 线段公式计算交点
double m1 = (_end.Y - _start.Y) / (_end.X - _start.X);
double m2 = (other._end.Y - other._start.Y) / (other._end.X - other._start.X);
// 如果斜率相同,则平行,直接返回null
if (m1 == m2)
return null;
double xIntersection = (_start.Y - other._start.Y) / (m1 - m2);
double yIntersection = m1 * xIntersection + _start.X;
return new Point(xIntersection, yIntersection);
}
// 判断两条直线是否平行
private bool IsParallel(Line other)
{
return (_start.X - _end.X) * (other._start.Y - other._end.Y) == (_start.Y - _end.Y) * (other._start.X - other._end.X);
}
}
class Program
{
static void Main(string[] args)
{
Point p1 = new Point(0, 0);
Point p2 = new Point(2, 2); // 直线1: 从原点开始,斜率为1
Point p3 = new Point(4, 4); // 直线1: 终点坐标
Point p4 = new Point(1, 1);
Point p5 = new Point(3, 3); // 直线2: 同样斜率为1,与直线1平行
Line line1 = new Line(p1, p2);
Line line2 = new Line(p4, p5);
Point intersection = line1.FindIntersectionWith(line2);
if (intersection != null)
Console.WriteLine($"Lines intersect at ({intersection.X}, {intersection.Y})");
else
Console.WriteLine("Lines are parallel and have no intersection.");
}
}
```
上述代码中,我们首先定义了一个 `Point` 类来存储点的坐标,并实现了一种简单的方法来计算两点间的距离。然后在 `Line` 类里,我们计算了两条线的斜率,判断它们是否平行,若不平行则通过线段公式找到交点。最后,在 `Main` 函数中创建了两条直线实例并调用 `FindIntersectionWith` 方法求交点。
阅读全文
相关推荐
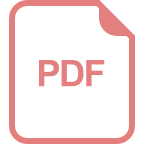
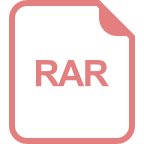















