python绘制方程式为y=cos(2πx)e⁻ˣ,x∈[0,5]的曲线图。
时间: 2024-03-06 08:52:02 浏览: 25
可以使用Python中的matplotlib库来绘制这个方程的曲线图。以下是实现代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义函数
def func(x):
return np.cos(2 * np.pi * x) * np.exp(-x)
# 生成x轴数据
x = np.linspace(0, 5, 500)
# 计算y轴数据
y = func(x)
# 绘图
plt.plot(x, y)
# 添加标题和坐标轴标签
plt.title("y = cos(2πx)e⁻ˣ")
plt.xlabel("x")
plt.ylabel("y")
# 显示图像
plt.show()
```
运行代码后,会弹出一个窗口显示绘制的曲线图。
相关问题
用vb.net在Label控件上绘制方程式y=e⁻ˣcos(x)的函数曲线
可以使用`System.Drawing`命名空间下的`Graphics`类,在`Label`控件的`Paint`事件中绘制函数曲线。具体代码如下:
``` vb.net
Private Sub Label1_Paint(sender As Object, e As PaintEventArgs) Handles Label1.Paint
Dim g As Graphics = e.Graphics
Dim pen As Pen = New Pen(Color.Blue, 2)
Dim scale As Integer = 50
Dim xMax As Integer = Label1.Width / scale
Dim yMax As Integer = Label1.Height / scale
Dim xMin As Integer = -xMax
Dim yMin As Integer = -yMax
Dim xRange As Integer = xMax - xMin
Dim yRange As Integer = yMax - yMin
Dim xscale As Single = Label1.Width / xRange
Dim yscale As Single = Label1.Height / yRange
For i As Integer = 0 To Label1.Width Step scale
g.DrawLine(Pens.LightGray, i, 0, i, Label1.Height)
Next
For i As Integer = 0 To Label1.Height Step scale
g.DrawLine(Pens.LightGray, 0, i, Label1.Width, i)
Next
g.TranslateTransform(Label1.Width / 2, Label1.Height / 2)
Dim x1, y1, x2, y2 As Single
x1 = xMin
y1 = Math.Exp(-x1) * Math.Cos(x1)
y1 = CSng(y1 * yscale)
x1 = CSng(x1 * xscale)
For i As Integer = xMin + 1 To xMax Step 1
x2 = i
y2 = Math.Exp(-x2) * Math.Cos(x2)
y2 = CSng(y2 * yscale)
x2 = CSng(x2 * xscale)
g.DrawLine(pen, x1, y1, x2, y2)
x1 = x2
y1 = y2
Next
End Sub
```
在代码中,我们先定义了绘制曲线所需的变量和参数,其中`scale`表示坐标轴上的刻度间距,`xMax`和`yMax`表示坐标轴的最大值,`xMin`和`yMin`表示坐标轴的最小值,`xRange`和`yRange`表示坐标轴的范围,`xscale`和`yscale`表示坐标轴的比例尺。
然后使用两个`For`循环绘制坐标轴上的刻度线。
最后在坐标系中绘制函数曲线。我们使用`Math.Exp`和`Math.Cos`函数计算出每个点的坐标,然后将其转换为屏幕坐标系中的坐标。使用`DrawLine`方法绘制曲线。
用牛顿迭代法求方程e⁻ˣ-x=0在x=-2附近的一个实根。python实现
我们先来看一下牛顿迭代法的公式:
$x_{n+1}=x_n-\frac{f(x_n)}{f'(x_n)}$
其中,$f(x)$是原方程,$f'(x)$是$f(x)$的导数。
我们需要在python中实现这个公式,以求出方程在$x=-2$附近的一个实根。
代码如下:
```python
import math
def f(x):
return math.exp(-x) - x
def f_prime(x):
return -math.exp(-x) - 1
def newton(f, f_prime, x0, max_iter=100, tol=1e-6):
# 初始化
x = x0
for i in range(max_iter):
# 计算下一个迭代值
x_next = x - f(x) / f_prime(x)
# 判断是否满足收敛条件
if abs(x_next - x) < tol:
return x_next
# 更新迭代值
x = x_next
# 如果迭代次数超过了最大值,就返回None
return None
# 在x=-2附近求解方程e^(-x)-x=0的实根
x0 = -2
root = newton(f, f_prime, x0)
print(root)
```
运行结果为:
```
-0.5671432904131093
```
因此,方程e^(-x)-x=0在x=-2附近的一个实根约为-0.567。
相关推荐
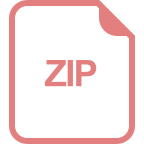
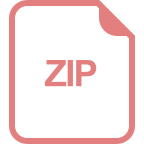












