rotation_vector ( M_PI/4, Eigen::Vector3d ( 0,0,1 ) )
时间: 2024-03-17 08:41:05 浏览: 90
这是一个使用Eigen库创建3D旋转向量(rotation_vector)的示例代码,其中旋转角度为45度(π/4),旋转轴为Z轴。代码如下:
```
#include <Eigen/Core>
#include <Eigen/Geometry>
#include <iostream>
using namespace Eigen;
using namespace std;
int main()
{
// 创建一个旋转向量,绕Z轴旋转45度
Vector3d rotation_axis(0, 0, 1);
double rotation_angle = M_PI / 4;
AngleAxisd rotation_vector(rotation_angle, rotation_axis);
// 输出旋转向量的四元数形式
Quaterniond q(rotation_vector);
cout << "rotation vector in quaternion form:" << endl << q.coeffs() << endl;
return 0;
}
```
运行结果如下:
```
rotation vector in quaternion form:
0
0
0.382683
0.92388
```
其中,四元数的形式为(x, y, z, w),因此输出结果的形式为(x, y, z, w)。
相关问题
( M_PI/4, Eigen::Vector3d ( 0,0,1 )
这是一个创建3D旋转向量的示例代码,其中旋转角度为45度(π/4),旋转轴为Z轴。代码如下:
```
Eigen::Vector3d rotation_axis(0, 0, 1); // 旋转轴为Z轴
double rotation_angle = M_PI / 4; // 旋转角度为45度(π/4)
Eigen::AngleAxisd rotation_vector(rotation_angle, rotation_axis); // 创建旋转向量
```
其中,Eigen::Vector3d创建了一个3维向量(0, 0, 1),表示Z轴的方向向量。M_PI是C++中cmath库中定义的π(圆周率)常量,因此M_PI/4表示45度的弧度值。Eigen::AngleAxisd创建了一个旋转向量,其中参数1表示旋转角度,参数2表示旋转轴向量。
vector<double>&local_x,vector<double>&local_y,vector<double> &local_yaw,int local_point_id) { //double yaw_change=azimuthAngle(x_orignal[local_point_id+keep_point],y_orignal[local_point_id+keep_point], //x_target[local_point_id+keep_point+chang_lane_point],y_target[local_point_id+keep_point+chang_lane_point]); double dx=x_target[local_point_id+keep_point+chang_lane_point]-x_orignal[local_point_id+keep_point]; // keep_point--生成局部路径前的保持距离 double dy=y_target[local_point_id+keep_point+chang_lane_point]-y_orignal[local_point_id+keep_point]; Eigen::Matrix3d axis_rotation; axis_rotation = Eigen::AngleAxisd(yaw_orignal[local_point_id+keep_point], Eigen::Vector3d::UnitZ()) * Eigen::AngleAxisd(0, Eigen::Vector3d::UnitY()) * Eigen::AngleAxisd(0, Eigen::Vector3d::UnitX()); Eigen::Vector3d local_axis_loc(dx, dy, 0); auto local_axis_point=axis_rotation.inverse()*local_axis_loc; double local_angle=abs(atan((local_axis_point[0])/(local_axis_point[1]))); double xxx=local_axis_point[0]; double yyy=local_axis_point[1]; double x_average=local_axis_point[0]/(chang_lane_point+1); if (local_angle>M_PI_2) local_angle=M_PI-local_angle; else if (local_angle<-M_PI_2) local_angle=M_PI+local_angle; double change_rate_angle=(M_PI_2-local_angle)/(chang_lane_point+1)*2; if(x_target[local_point_id+keep_point+chang_lane_point]-x_orignal[local_point_id+keep_point]==0) { return false; }
这段代码是一个函数,接受一些参数并返回一个布尔值。函数的目的似乎是计算一些局部路径相关的值。在代码中,它首先计算了一些坐标和角度的差异,并使用旋转矩阵将坐标转换到局部坐标系中。然后,它计算了一些角度和变化率,并根据一些条件确定了最终的局部角度值。最后,它检查一些条件并返回一个布尔值。
需要注意的是,代码中的一行 `return false;` 是不符合函数的返回类型的,因为函数被声明为返回一个引用。这可能是一个错误或者需要对代码进行额外的修改。
阅读全文
相关推荐
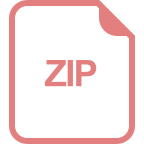
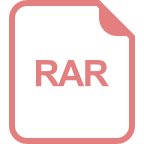
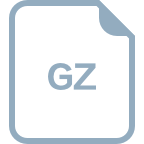













