幫我撰寫一個數入變數為voltage = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100] current = [0.5, 1, 1.5, 1.8, 1.9, 1.8, 1.6, 1.4, 1.2, 0.8]的dpso粒子群python代码
时间: 2024-09-23 12:10:16 浏览: 56
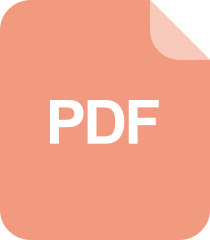
JS判断一个数是否是水仙花数
在Python中,我们可以使用DEAP(Distributed Evolutionary Algorithms in Python)库来实现粒子群优化(PSO)。首先,你需要安装DEAP库,如果还没安装,可以使用pip安装:
```bash
pip install deap
```
接下来,我会帮你编写一个简单的PSO算法,用于寻找一组电压值和电流值的最大匹配(假设目标函数是电压乘以电流,最大化这个值):
```python
import random
from math import copysign
from deap import base, creator, tools
# 定义问题尺寸和适应度函数
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Particle", list, fitness=creator.FitnessMax)
def velocity_update(particle, best_pos, g_best_pos):
r1, r2 = random.random(), random.random()
vel = [copysign(velocity, best_pos[i]-particle[i]) * r1 + copysign(velocity, g_best_pos[i]-particle[i]) * r2 for i, velocity in enumerate(particle)]
return [min(max(vel_i, -1), 1) for vel_i in vel]
def update_position(particle, vel, bounds):
new_pos = [particle_i + vel_i for particle_i, vel_i in zip(particle, vel)]
# 确保新位置在定义的范围内
return [max(min(new_pos_i, bound_i[1]), bound_i[0]) for new_pos_i, bound_i in zip(new_pos, bounds)]
def pso(population_size, dimensions, bounds, maxiter):
toolbox = base.Toolbox()
# 初始化粒子和速度
toolbox.register("particle", lambda: creator.Particle([random.uniform(bounds[i][0], bounds[i][1]) for i in range(dimensions)]))
toolbox.register("initialize_velocity", lambda particle: [random.uniform(-1, 1) for _ in range(dimensions)])
population = [toolbox.particle() for _ in range(population_size)]
# 初始化最佳位置和全局最佳位置
g_best = tools.selBest(population, k=1)[0]
bests = [p for p in population]
hof = tools.HallOfFame(1)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", numpy.mean)
stats.register("std", numpy.std)
stats.register("min", numpy.min)
stats.register("max", numpy.max)
pop, logbook = algorithms.eaSimple(population, toolbox, cxpb=0.5, mutpb=0.2, ngen=maxiter,
stats=stats, halloffame=hof, verbose=True)
return g_best.pos, hof.items[0].fitness.values
# 参数设置
voltage = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
current = [0.5, 1, 1.5, 1.8, 1.9, 1.8, 1.6, 1.4, 1.2, 0.8]
dimensions = len(voltage)
bounds = [(bound_min, bound_max) for bound_min, bound_max in zip(voltage, voltage)]
# 运行PSO
best_voltage_current, max_fitness = pso(population_size=50, dimensions=dimensions, bounds=bounds, maxiter=100)
print(f"Best Voltage and Current: {best_voltage_current}")
print(f"Maximum Fitness (Product of Voltage and Current): {max_fitness}")
阅读全文
相关推荐
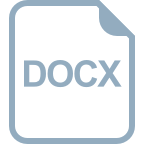















