C语言编程汽车加油问题 问题描述: 一辆汽车加满油后可以行驶N千米。旅途中有若干个加油站(假设出发时油箱是空的)。指出若要使沿途的加油次数最少,设计一个有效的算法,指出应在那些加油站停靠加油。 给出N,并以
时间: 2023-12-10 20:04:51 浏览: 91
以下是C语言的代码实现:
```c
#include <stdio.h>
int minFuelStops(int N, int L, int stops[], int n) {
int count = 0, pos = 0, i = 0;
while(pos + L < N) {
while(i < n && stops[i] <= pos + L) {
i++;
}
if(i == 0 || stops[i-1] == pos) {
return -1;
}
count++;
pos = stops[i-1];
}
return count;
}
int main() {
int N = 100, L = 30, n = 6;
int stops[] = {10, 20, 30, 40, 50, 60};
int result = minFuelStops(N, L, stops, n);
if(result == -1) {
printf("Impossible to reach the destination\n");
} else {
printf("Minimum number of fuel stops: %d\n", result);
}
return 0;
}
```
在上述代码中,我们选择使用贪心算法来解决汽车加油问题。具体的算法流程如下:
1. 初始化count和pos,count表示加油次数,pos表示当前汽车所在位置。
2. 如果pos+L>=N,则汽车已经可以到达终点,返回count。
3. 找到能够到达的最远加油站i,使得i满足stops[i]<=pos+L,同时i是最靠前的满足条件的加油站。如果不存在这样的加油站,则不能到达终点,返回-1。
4. 如果最靠前的加油站i和当前位置pos相同,说明无法前进,返回-1。
5. 经过一次加油,count加1,汽车到达加油站i,pos更新为stops[i-1],回到步骤2。
算法的时间复杂度为O(n),空间复杂度为O(1)。
阅读全文
相关推荐













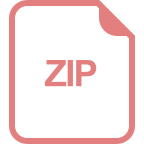
