msp430f5529舵机控制代码
时间: 2023-07-27 11:08:23 浏览: 170
Sure! Here's an example code for controlling a servo motor using MSP430F5529 microcontroller:
```c
#include <msp430.h>
#define PWM_PERIOD 20000 // PWM period in microseconds
#define SERVO_MIN_PULSE 1000 // Servo minimum pulse duration in microseconds
#define SERVO_MAX_PULSE 2000 // Servo maximum pulse duration in microseconds
void delay_us(unsigned int us)
{
unsigned int i;
for(i=0; i < us/2; i++)
__delay_cycles(1);
}
void servo_init()
{
TA0CTL = TASSEL_2 + MC_0; // SMCLK, stop mode
TA0CCR0 = PWM_PERIOD - 1; // PWM period
TA0CCTL1 = OUTMOD_7; // Reset/Set mode for CCR1
TA0CCR1 = SERVO_MIN_PULSE; // Initial pulse duration
P1DIR |= BIT2; // P1.2 as TA0.1 output
P1SEL |= BIT2; // P1.2 as TA0.1
TA0CTL |= MC_1; // Start timer in up mode
}
void servo_set_angle(unsigned int angle)
{
unsigned int pulse_width = SERVO_MIN_PULSE + (SERVO_MAX_PULSE - SERVO_MIN_PULSE) * angle / 180;
TA0CCR1 = pulse_width;
delay_us(1000); // Delay to allow the servo to move
}
int main(void)
{
WDTCTL = WDTPW | WDTHOLD; // Stop watchdog timer
servo_init(); // Initialize servo motor
while(1)
{
servo_set_angle(0); // Set servo angle to 0 degrees
__delay_cycles(1000000); // Delay for 1 second
servo_set_angle(90); // Set servo angle to 90 degrees
__delay_cycles(1000000); // Delay for 1 second
servo_set_angle(180); // Set servo angle to 180 degrees
__delay_cycles(1000000); // Delay for 1 second
}
}
```
In this code, the servo motor is connected to Pin P1.2 (TA0.1) of MSP430F5529 microcontroller. The `servo_init()` function initializes the Timer_A0 module for PWM generation, and the `servo_set_angle()` function sets the desired angle of the servo motor by adjusting the pulse width.
Please note that the actual servo control values may vary depending on the specific servo motor used. You may need to adjust the `SERVO_MIN_PULSE` and `SERVO_MAX_PULSE` values according to your servo's specifications. Also, make sure to configure the clock settings appropriately in your main code.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
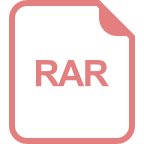





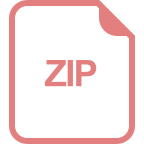








