设有n个元素的集合a[0]~a[n-1]是有序的,设计算法从这n个元素中找出为x的特定元素用c++代码写出来
时间: 2024-10-18 20:22:52 浏览: 28
在C++中,如果数组已经按照升序排列,我们可以使用二分查找法来寻找特定元素`x`。以下是简单的二分查找算法实现:
```cpp
#include <iostream>
using namespace std;
// 二分查找函数
int binarySearch(int arr[], int left, int right, int x) {
if (right >= left) {
int mid = left + (right - left) / 2;
// 如果中间元素正好是目标值,返回其索引
if (arr[mid] == x)
return mid;
// 如果目标值小于中间元素,则在左半部分继续搜索
else if (arr[mid] > x)
return binarySearch(arr, left, mid - 1, x);
// 否则,在右半部分继续搜索
else
return binarySearch(arr, mid + 1, right, x);
}
// 如果没有找到,返回-1表示目标不在数组中
return -1;
}
int main() {
int n;
cout << "Enter the size of the array: ";
cin >> n;
int a[n];
cout << "Enter the elements in ascending order: ";
for(int i=0; i<n; i++) {
cin >> a[i];
}
int x;
cout << "Enter the target value to search: ";
cin >> x;
int result = binarySearch(a, 0, n-1, x);
if(result != -1)
cout << "Element found at index " << result << endl;
else
cout << "Element not found" << endl;
return 0;
}
```
阅读全文
相关推荐
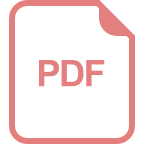
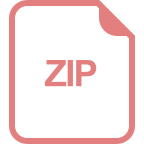
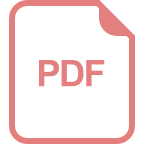















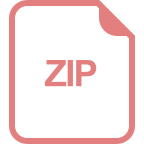
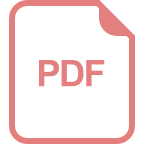