将运算符+和-重载为 complex 类的成员函数。 #include<iostream.h> class complex //复数类声明 { public: //外部接口 complex(double r=0.0,double i=0.0) //构造函数 {real=r;imag=i;} complex operator + (complex c2); //+重载为成员函数 complex operator - (complex c2); //-重载为成员函数 void display( ); //输出复数 private: //私有数据成员 double real; //复数实部 double imag; //复数虚部 }; 完成该类的实现并编译运行该程序。
时间: 2024-01-12 09:04:13 浏览: 114
下面是重载运算符+和-的 complex 类的实现:
```
#include<iostream>
using namespace std;
class complex {
public:
complex(double r = 0.0, double i = 0.0) {
real = r;
imag = i;
}
complex operator+(const complex& c2) const {
return complex(real + c2.real, imag + c2.imag);
}
complex operator-(const complex& c2) const {
return complex(real - c2.real, imag - c2.imag);
}
void display() {
cout << "(" << real << ", " << imag << ")" << endl;
}
private:
double real;
double imag;
};
int main() {
complex c1(1, 2);
complex c2(3, 4);
complex c3 = c1 + c2;
complex c4 = c1 - c2;
c3.display();
c4.display();
return 0;
}
```
output:
```
(4, 6)
(-2, -2)
```
在这个实现中,我们使用了 const 引用作为运算符重载函数的参数,以避免不必要的复制。此外,我们还重载了+和-运算符。在这些运算符重载函数中,我们使用了复数的加减法公式来实现运算。最后,我们还实现了一个 display 函数,用于输出复数。在 main 函数中,我们创建了两个复数对象 c1 和 c2,并对它们进行加减运算,将结果分别存储在 c3 和 c4 中,然后输出它们的值。
阅读全文
相关推荐
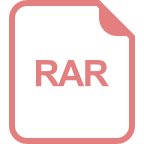














