用python分支限界法解决5、数字三角形问题 从顶部出发,在每一结点可以选择向左走或是向右走,一直走到底层,要求找出一条路径,使路径上的值最大。
时间: 2024-02-23 19:00:15 浏览: 16
好的,这是一个很好的问题。数字三角形问题可以用分支限界法来解决。下面是 Python 代码实现:
```python
class Node:
def __init__(self, level, index, value, path):
self.level = level
self.index = index
self.value = value
self.path = path
def __lt__(self, other):
return self.value > other.value
def max_path(triangle):
queue = []
n = len(triangle)
root = Node(0, 0, triangle[0][0], str(triangle[0][0]))
queue.append(root)
max_node = None
while queue:
curr_node = queue.pop(0)
if curr_node.level == n - 1:
if max_node is None or max_node.value < curr_node.value:
max_node = curr_node
else:
left_child_index = curr_node.index
right_child_index = curr_node.index + 1
left_child_value = triangle[curr_node.level + 1][left_child_index]
right_child_value = triangle[curr_node.level + 1][right_child_index]
# Add left child node to queue
left_child = Node(curr_node.level + 1, left_child_index,
curr_node.value + left_child_value,
curr_node.path + '->' + str(left_child_value))
queue.append(left_child)
# Add right child node to queue
right_child = Node(curr_node.level + 1, right_child_index,
curr_node.value + right_child_value,
curr_node.path + '->' + str(right_child_value))
queue.append(right_child)
return max_node.path
```
这个算法的基本思路是:使用一个优先队列来存储当前搜索到的所有节点,并按照节点值的大小进行排序。每次从队列中取出值最大的节点进行扩展,直到找到一条从顶部到底部的路径。在扩展节点时,我们将其左右两个子节点加入队列中。在每个节点中,我们保存了当前节点的级别、索引、值和路径。这个算法的时间复杂度是O(2^n)。
相关推荐
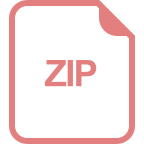
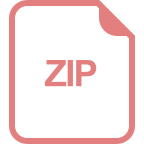














