#include <Servo.h> Servo myservo; // create servo object to control a servo int switchPin = 2; // switch input pin int servoPin = 9; // servo output pin int switchState = 0; // variable for reading the switch status int servoAngle = 0; // variable for storing the servo angle void setup() { myservo.attach(servoPin); // attaches the servo on pin 9 to the servo object pinMode(switchPin, INPUT); // set the switch pin as input Serial.begin(9600); // initialize serial communication at 9600 bits per second } void loop() { switchState = digitalRead(switchPin); // read the switch state if (switchState == HIGH) { // if the switch is pressed servoAngle = 90; // set the servo angle to 90 degrees myservo.write(servoAngle); // move the servo to the desired angle Serial.println("Switch is on!"); // print a message to the serial monitor } else { // if the switch is not pressed servoAngle = 0; // set the servo angle to 0 degrees myservo.write(servoAngle); // move the servo to the desired angle Serial.println("Switch is off!"); // print a message to the serial monitor } delay(100); // wait for 100 milliseconds }翻译一下这段代码
时间: 2024-03-12 15:44:58 浏览: 22
这段代码是一个基于Arduino的程序,使用了Servo库和一个开关按钮来控制一个舵机的旋转角度。以下是这段代码的翻译:
```
#include <Servo.h> // 引入Servo库
Servo myservo; // 创建一个舵机对象
int switchPin = 2; // 开关输入引脚
int servoPin = 9; // 舵机输出引脚
int switchState = 0; // 读取开关状态的变量
int servoAngle = 0; // 存储舵机角度的变量
void setup() {
myservo.attach(servoPin); // 把舵机引脚9连接到舵机对象
pinMode(switchPin, INPUT); // 把开关引脚设为输入
Serial.begin(9600); // 初始化串口通信,波特率为9600
}
void loop() {
switchState = digitalRead(switchPin); // 读取开关状态
if (switchState == HIGH) { // 如果开关被按下
servoAngle = 90; // 设置舵机角度为90度
myservo.write(servoAngle); // 移动舵机到所需角度
Serial.println("Switch is on!"); // 在串口监视器中打印一条消息
} else { // 如果开关没有被按下
servoAngle = 0; // 设置舵机角度为0度
myservo.write(servoAngle); // 移动舵机到所需角度
Serial.println("Switch is off!"); // 在串口监视器中打印一条消息
}
delay(100); // 延迟100毫秒
}
```
相关推荐
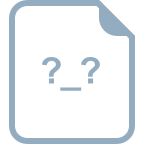














