ros发布节点信息python_ROS Twist和Odometry消息类型使用(Python)
时间: 2024-02-22 16:57:54 浏览: 192
在 Python 中使用 ROS 发布 Twist 和 Odometry 消息类型的步骤如下:
1. 导入必要的 ROS Python 库和消息类型:
```python
import rospy
from geometry_msgs.msg import Twist
from nav_msgs.msg import Odometry
```
2. 初始化 ROS 节点:
```python
rospy.init_node('my_node')
```
3. 创建 Twist 和 Odometry 的 Publisher:
```python
twist_pub = rospy.Publisher('cmd_vel', Twist, queue_size=10)
odom_pub = rospy.Publisher('odom', Odometry, queue_size=10)
```
4. 构造 Twist 和 Odometry 消息:
```python
twist_msg = Twist()
twist_msg.linear.x = 0.1
twist_msg.angular.z = 0.5
odom_msg = Odometry()
odom_msg.pose.pose.position.x = 1.0
odom_msg.pose.pose.position.y = 2.0
odom_msg.pose.pose.position.z = 0.0
odom_msg.pose.pose.orientation.x = 0.0
odom_msg.pose.pose.orientation.y = 0.0
odom_msg.pose.pose.orientation.z = 0.0
odom_msg.pose.pose.orientation.w = 1.0
```
5. 发布消息:
```python
twist_pub.publish(twist_msg)
odom_pub.publish(odom_msg)
```
完整的代码示例:
```python
import rospy
from geometry_msgs.msg import Twist
from nav_msgs.msg import Odometry
rospy.init_node('my_node')
twist_pub = rospy.Publisher('cmd_vel', Twist, queue_size=10)
odom_pub = rospy.Publisher('odom', Odometry, queue_size=10)
twist_msg = Twist()
twist_msg.linear.x = 0.1
twist_msg.angular.z = 0.5
odom_msg = Odometry()
odom_msg.pose.pose.position.x = 1.0
odom_msg.pose.pose.position.y = 2.0
odom_msg.pose.pose.position.z = 0.0
odom_msg.pose.pose.orientation.x = 0.0
odom_msg.pose.pose.orientation.y = 0.0
odom_msg.pose.pose.orientation.z = 0.0
odom_msg.pose.pose.orientation.w = 1.0
twist_pub.publish(twist_msg)
odom_pub.publish(odom_msg)
rospy.spin()
```
这里 `rospy.spin()` 是防止程序退出的语句,它会一直等待 ROS 节点的关闭信号。
阅读全文
相关推荐
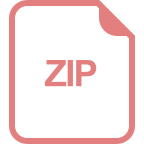
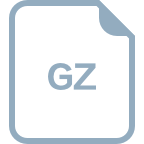
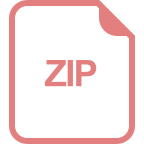
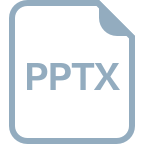
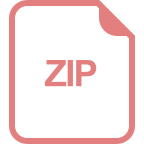
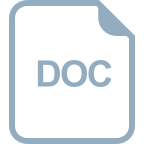
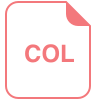











