public void keyPressed(KeyEvent e) { lbl.setText("键盘按键输入数字"); for(int i=0;i<key.length;i++){ for(int j=0;j<key[i].length();j++){ if(btn[i][j].getText().equals(String.valueOf( Character.toUpperCase(e.getKeyChar())))){ btn[i][j].setBackground(Color.red); ta.append(btn[i][j].getText()); } } } }
时间: 2023-06-17 11:08:31 浏览: 104
这是一个 Java Swing 中的键盘事件监听器,当用户按下键盘上的某个键时,会触发该方法。该方法会根据用户输入的字符,将对应的按钮背景色设置为红色,并将该字符显示在文本区域中。其中,btn 是一个二维数组,用于存储数字键的按钮,key 是一个字符串数组,用于存储数字键的字符值。通过遍历 btn 数组和 key 数组,可以匹配用户输入的字符和对应的按钮,并进行相应的操作。
相关问题
package test; import java.awt.*; import javax.swing.*; import java.awt.event.*; import java.awt.event.InputEvent; import java.awt.event.KeyEvent; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.JButton; import java.awt.BorderLayout; import java.awt.GridLayout; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; import java.util.Random; public class Test3{ public static void main (String args[]) { Win win=new Win(); win.setTitle("输入序列号"); win.setBounds(10,10,460,360); } } class Police implements KeyListener,FocusListener{ public void keyPressed(KeyEvent e) { JTextField t=(JTextField)e.getSource(); if (t.getCarePosition()>=6) t.transferFocus(); } public void keyTyped(KeyEvent e) {} public void keyReleased(KeyEvent e) {} public void focusGained(FocusEvent e) { JTextField text=(JTextField)e.getSource(); text.setText(null); } public void focusLost(FocusEvent e){} } class Win extends JFrame { JTextField text[]=new JTextField[3]; Police police; JButton b; Win() { setLayout(new FlowLayout()); police = new Police(); for(int i=0;i<3;i++) { text[i]=new JTextField(7); text[i].addKeyListener(police); //监视键盘事件 text[i].addFocusListener(police); add(text[i]); } b=new JButton("确定"); add(b); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); text[0].requestFocusInWindow(); } }哪里有错误
代码的缩进格式不够规范,需要进行调整。此外,代码中的一些注释也需要进行调整,以保证清晰易读。以下是修改后的代码:
```
package test;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.util.Random;
public class Test3{
public static void main (String args[]) {
Win win=new Win();
win.setTitle("输入序列号");
win.setBounds(10,10,460,360);
}
}
class Police implements KeyListener,FocusListener{
public void keyPressed(KeyEvent e) {
JTextField t=(JTextField)e.getSource();
if (t.getCarePosition()>=6)
t.transferFocus();
}
public void keyTyped(KeyEvent e) {}
public void keyReleased(KeyEvent e) {}
public void focusGained(FocusEvent e) {
JTextField text=(JTextField)e.getSource();
text.setText(null);
}
public void focusLost(FocusEvent e){}
}
class Win extends JFrame {
JTextField text[]=new JTextField[3];
Police police;
JButton b;
Win() {
setLayout(new FlowLayout());
police = new Police();
for(int i=0;i<3;i++) {
text[i]=new JTextField(7);
text[i].addKeyListener(police); //监视键盘事件
text[i].addFocusListener(police);
add(text[i]);
}
b=new JButton("确定");
add(b);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
text[0].requestFocusInWindow();
}
}
```
package Shixun4_8; import javax.swing.*; import java.awt.*; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; class FrameWithKeyEvent extends JFrame implements KeyListener { JButton b[]=new JButton[3]; int x,y; FrameWithKeyEvent(){ setSize(300,300); setVisible(true); setDefaultCloseOperation(EXIT_ON_CLOSE); setLayout(new FlowLayout()); for(int i=0;i<=2;i++) { b[i]=new JButton(" "+i); b[i].addKeyListener(this); add(b[i]); } validate(); } public void keyPressed(KeyEvent e) { JButton button=(JButton)e.getSource(); x=button.getBounds().x; y=button.getBounds().y; if(e.getKeyCode()==KeyEvent.VK_UP) { y=y-2; if(y<=0)y=300; button.setLocation(x,y); } else if(e.getKeyCode()== KeyEvent.VK_DOWN) { y=y+2; if(y>=300)y=0; button.setLocation(x,y); } else if(e.getKeyCode()==KeyEvent.VK_LEFT) { x=x-2; if(x<=0)x=300; button.setLocation(x,y); } else if(e.getKeyCode()==KeyEvent.VK_RIGHT) { x=x+2; if(x>=300)x=0; button.setLocation(x,y); } } public void keyReleased(KeyEvent e) {} public void keyTyped(KeyEvent e) {} } public class Shixun4_8 { public static void main(String[] args) { new FrameWithKeyEvent(); } }逐行代码解释
首先,这是一个Java程序,包含一个名为Shixun4_8的public class。
第1-2行导入了javax.swing和java.awt包。
第4-23行定义了一个名为FrameWithKeyEvent的类,它扩展了JFrame并实现了KeyListener接口。该类包含三个按钮和一个构造函数。
在构造函数中,首先调用setSize()方法来设置窗口的大小为300x300像素。接着,调用setVisible()方法使窗口可见,调用setDefaultCloseOperation()方法以便在关闭窗口时退出程序,调用setLayout()方法来设置按钮的布局方式为FlowLayout。然后,使用for循环创建三个按钮,并将每个按钮添加到窗口中。最后,调用validate()方法以确保窗口的内容被正确布局。
在FrameWithKeyEvent类中,实现了三个方法:keyPressed()、keyReleased()和keyTyped(),这些方法都是从KeyListener接口继承而来的。
在keyPressed()方法中,首先使用getSource()方法获取触发事件的组件,然后获取该组件的位置坐标x和y。根据按下的键的不同,分别对x和y进行加减操作,以实现按钮的移动。如果移动到了边缘,就将位置坐标重置为相反的边缘位置。最后,使用setLocation()方法将按钮移动到新的位置。
在keyReleased()和keyTyped()方法中,由于我们不需要响应这两个事件,因此这两个方法的实现为空。
最后,在main()方法中,创建了一个FrameWithKeyEvent对象,以启动程序。
阅读全文
相关推荐
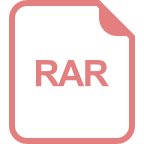
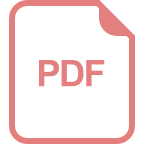
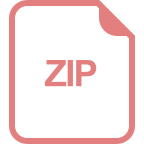














