栈的应用案例:后缀表达式求值实战,深入解析
发布时间: 2024-08-23 20:18:26 阅读量: 37 订阅数: 40 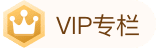
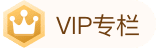
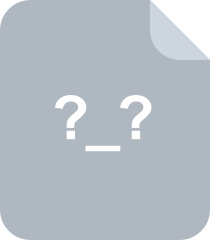
[3.7.1]--307)栈的应用:后缀表达式求值.srt
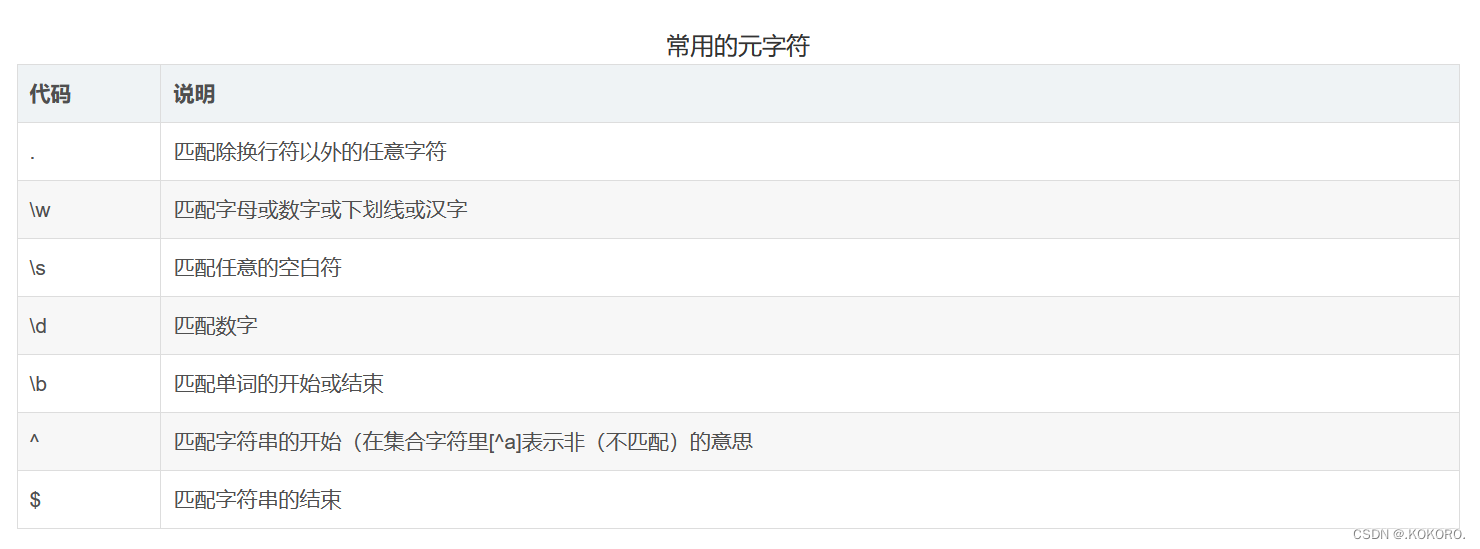
# 1. 栈的概念与操作
栈是一种先进后出(LIFO)的数据结构,其主要操作包括:
- **入栈(push)**:将元素添加到栈顶。
- **出栈(pop)**:从栈顶移除元素并返回该元素。
- **栈顶(top)**:返回栈顶元素,但不移除它。
- **栈空(empty)**:检查栈是否为空。
栈的这些操作使它成为许多计算机科学应用中的理想数据结构,例如后缀表达式求值、递归函数实现和浏览器历史记录管理。
# 2. 后缀表达式求值
### 2.1 后缀表达式简介
后缀表达式,也称为逆波兰表示法,是一种数学表达式表示法,其中运算符置于操作数之后。例如,表达式 `1 + 2 * 3` 的后缀表达式为 `1 2 3 * +`。
后缀表达式具有以下优点:
- **无需括号:** 后缀表达式中无需使用括号,因为运算符的优先级已由其位置确定。
- **易于求值:** 后缀表达式可以从左到右依次求值,无需考虑运算符优先级。
### 2.2 栈在后缀表达式求值中的应用
栈是一种后进先出(LIFO)的数据结构,非常适合用于后缀表达式的求值。
#### 2.2.1 栈的初始化
在开始求值之前,需要创建一个栈来存储操作数。栈的初始化过程如下:
```python
def init_stack():
stack = []
return stack
```
#### 2.2.2 栈的入栈和出栈操作
入栈操作将元素添加到栈顶,出栈操作从栈顶移除元素。
```python
def push(stack, element):
stack.append(element)
def pop(stack):
if len(stack) > 0:
return stack.pop()
else:
raise IndexError("Stack is empty")
```
#### 2.2.3 后缀表达式求值的具体步骤
后缀表达式的求值过程如下:
1. 从左到右遍历后缀表达式。
2. 如果遇到操作数,将其入栈。
3. 如果遇到运算符,从栈中弹出两个操作数,执行运算,并将结果入栈。
4. 重复步骤 2 和 3,直到表达式结束。
5. 栈顶元素即为表达式的值。
**代码示例:**
```python
def evaluate_postfix(expression):
stack = init_stack()
for token in expression:
if token.isdigit():
push(stack, int(token))
else:
op1 = pop(stack)
op2 = pop(stack)
result = calculate(op1, op2, token)
push(stack, result)
return pop(stack)
def calculate(op1, op2, operator):
if operator == '+':
return op1 + op2
elif operator == '-':
return op1 - op2
elif operator == '*':
return op1 * op2
elif operator == '/':
return op1 / op2
```
**代码逻辑分析:**
- `evaluate_postfix` 函数初始化一个栈,然后遍历后缀表达式。
- 如果遇到操作数,则将其入栈。
- 如果遇到运算符,则从栈中弹出两个操作数,执行运算,并将结果入栈。
- 函数 `calculate` 根据运算符执行相应的运算。
- 最后,栈顶元素即为表达式的值。
# 3. 栈在计算机科学中的其他应用
栈在计算机科学中除了后缀表达式求值之外,还有着广泛的应用,包括:
### 3.1 递归函数的实现
递归函数是一种通过自身调用来解决问题的函数。在递归函数的执行过程中,栈扮演着至关重要的角色。
当一个递归函数被调用时,它会创建一个新的栈帧,其中包含了函数的局部变量、参数和返回地址。当函数执行完毕后,它会返回到调用它的栈帧,并继续执行。
例如,以下是一个计算阶乘的递归函数:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
```
当调用 `factorial(5)` 时,栈会发生如下变化:
```
栈帧 1
局部变量:n = 5
参数:5
返回地址:
```
0
0
相关推荐
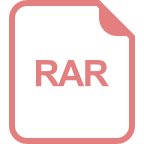
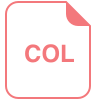
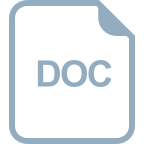
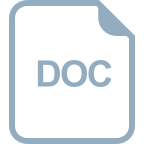
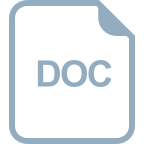
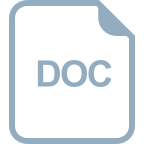
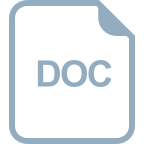