堆排序算法的扩展应用:探索堆排序的更多可能性,拓展算法应用范围
发布时间: 2024-07-21 01:27:54 阅读量: 24 订阅数: 32 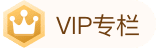
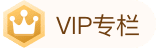
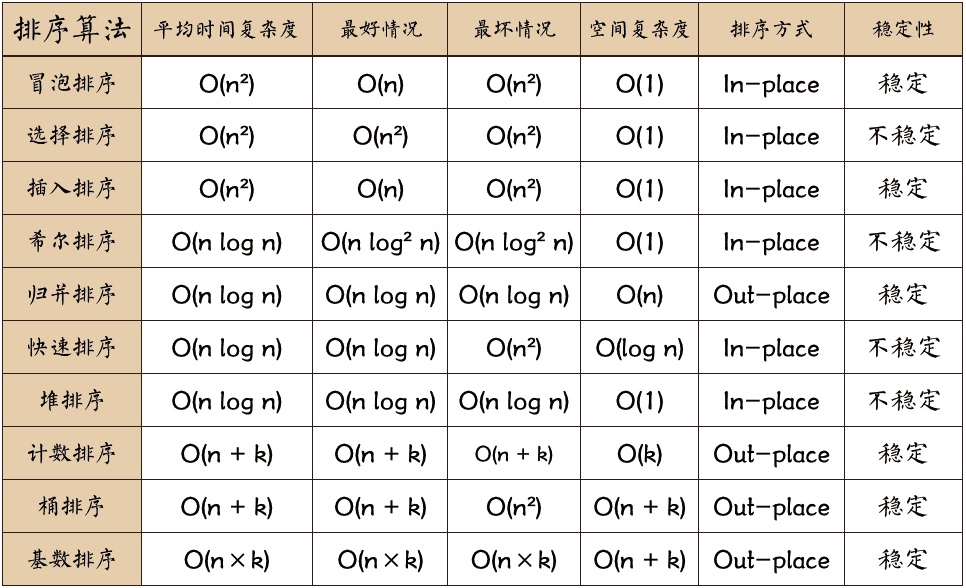
# 1. 堆排序算法基础**
堆排序是一种高效的排序算法,它基于二叉堆数据结构。二叉堆是一种完全二叉树,其中每个节点的值都大于或等于其子节点的值。
堆排序算法通过构建一个二叉堆,然后从堆中依次弹出最大元素,从而对数组进行排序。构建二叉堆的时间复杂度为 O(n),弹出最大元素的时间复杂度为 O(log n),因此堆排序的总时间复杂度为 O(n log n)。
# 2. 堆排序算法的扩展应用
堆排序算法是一种高效的排序算法,其时间复杂度为 O(n log n)。除了基本的排序功能外,堆排序算法还可以在数据结构和算法等领域中得到广泛的应用。
### 2.1 堆排序在数据结构中的应用
#### 2.1.1 优先队列的实现
堆排序算法可以用来实现优先队列,即一种遵循先入先出 (FIFO) 原则的数据结构,其中优先级最高的元素始终位于队列的开头。
**实现方式:**
1. 使用一个最大堆来存储元素,其中根节点始终包含优先级最高的元素。
2. 当插入新元素时,将其插入到堆的末尾,然后使用上浮操作将其移动到适当的位置。
3. 当删除元素时,删除根节点,然后使用下沉操作将其替换为堆中优先级最低的元素。
**代码块:**
```python
class MaxHeap:
def __init__(self):
self.heap = []
def insert(self, value):
self.heap.append(value)
self._upheap(len(self.heap) - 1)
def _upheap(self, index):
parent_index = (index - 1) // 2
while index > 0 and self.heap[index] > self.heap[parent_index]:
self.heap[index], self.heap[parent_index] = self.heap[parent_index], self.heap[index]
index = parent_index
def delete_max(self):
if len(self.heap) == 0:
return None
max_value = self.heap[0]
self.heap[0] = self.heap[-1]
self.heap.pop()
self._downheap(0)
return max_value
def _downheap(self, index):
left_index = 2 * index + 1
right_index = 2 * index + 2
largest_index = index
if left_index < len(self.heap) and self.heap[left_index] > self.heap[largest_index]:
largest_index = left_index
if right_index < len(self.heap) and self.heap[right_index] > self.heap[largest_index]:
largest_index = right_index
if largest_index != index:
self.heap[index], self.heap[largest_index] = self.heap[largest_index], self.heap[index]
self._downheap(largest_index)
```
**逻辑分析:**
* `insert` 函数使用上浮操作将新元素插入堆中,确保优先级最高的元素始终位于根节点。
* `delete_max` 函数使用下沉操作删除根节点,并将其替换为堆中优先级最低的元素。
* `_upheap` 和 `_downheap` 函数分别执行上浮和下沉操作,以维护堆的性质。
#### 2.1.2 堆排序树的构建
堆排序算法还可以用来构建堆排序树,这是一种完全二叉树,其中每个节点的值都大于或等于其子节点的值。
**实现方式:**
1. 将输入数组视为堆排序树的叶节点。
2. 从最后一个非叶节点开始,使用下沉操作将每个节点移动到其适当的位置。
**代码块:**
```python
def build_heap_sort_tree(array):
for i in range(len(array) // 2 - 1, -1, -1):
_downheap(array, i, len(array))
```
**逻辑分析:**
* `build_heap_sort_tree` 函数从最后一个非叶节点开始,使用下沉操作将每个节点移动到其适当的位置。
* `_downheap` 函数与优先队列中的 `_downheap` 函数类似,它将节点与其子节点进行比较,并将其移动到优先级最高的位置。
### 2.2 堆排序在算法中的应用
#### 2.2.1 快速排序的优化
堆排序算法可以用来优化快速排序算法。快速排序是一种基于分治思想的排序算法,其时间复杂度为 O(n log n)。然而,在某些情况下,快速排序的性能可能会退化为 O(n^2)。
**优化方式:**
1. 将快速排序中的分区操作替换为堆排序。
2. 在分区操作中,将子数组构建为一个堆,然后使用堆排序算法对子数组进行排序。
**代码块:**
```python
def heap_sort_partition(array, low, high):
# 构建堆
for i in range((low + high) // 2 - 1, -1, -1):
_downheap(array, i, high + 1)
# 排序
for i in range(high, low, -1):
array[i], array[low] = array[low], array[i]
_downheap(array, low, i)
```
**逻辑分析:**
* `heap_sort_partition` 函数首先将子数组构建为一个堆。
* 然后,它使用堆排序算法对堆进行排序。
* 最后,它将堆的根节点(即最大值)与子数组的第一个元素进行交换。
#### 2.2.2 外部排序算法的实现
堆排序算法可以用来实现外部排序算法,这是一种用于处理海量数据的排序算法。外部排序算法将数据存储在外部存储设备(如磁盘)上,并使用有限的内存对数据进行排序。
**实现方式:**
1. 将数据分成多个较小的块。
2. 使用堆排序算法对每个块进行排序。
3. 将排序后的块合并为一个排序后的文件。
**代码块:**
```python
def external_sort(input_file, output_file, block_size):
# 将数据分成块
block
```
0
0
相关推荐
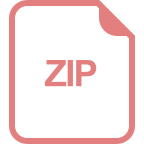
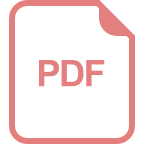
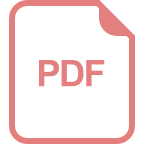





