堆排序在数据结构中的应用:揭秘堆排序在树形结构中的妙用,构建高效数据组织
发布时间: 2024-07-21 01:22:03 阅读量: 25 订阅数: 32 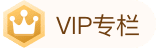
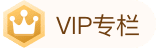
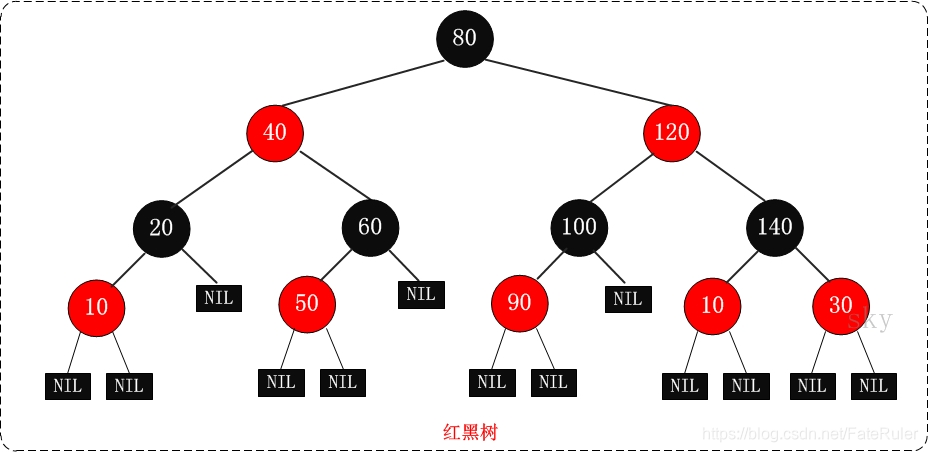
# 1. 堆排序的基本原理**
堆排序是一种基于二叉堆数据结构的排序算法。它利用堆的特性,将无序数据组织成一个完全二叉树,其中每个节点的值都大于或等于其子节点的值。通过不断调整堆,使根节点始终为最大值,从而实现排序。
堆排序的思想是:
1. 将输入数组构建成一个最大堆。
2. 将堆顶元素(最大值)与堆尾元素交换,并重新调整堆,使堆顶元素为新最大值。
3. 重复步骤 2,直到堆中只剩一个元素,此时数组已排序。
# 2. 堆排序的实现技巧
### 2.1 堆排序算法的步骤和实现
#### 2.1.1 构建最大堆
最大堆是一种完全二叉树,其中每个节点的值都大于或等于其子节点的值。构建最大堆的过程如下:
1. 将输入数组视为一棵完全二叉树,其中每个元素都是一个叶节点。
2. 从最后一个非叶节点开始(即数组中间的元素),依次对每个非叶节点执行以下操作:
- 与其左右子节点比较,将最大值移动到该节点。
- 重复上述步骤,直到该节点成为最大堆的一部分。
**代码块:**
```python
def build_max_heap(arr):
"""
构建最大堆。
参数:
arr: 输入数组。
"""
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
max_heapify(arr, i, n)
```
**逻辑分析:**
* `n // 2 - 1` 是最后一个非叶节点的索引。
* 外层循环从最后一个非叶节点开始,依次对每个非叶节点执行 `max_heapify` 操作。
* `max_heapify` 函数将当前节点与其左右子节点比较,并将最大值移动到当前节点。
#### 2.1.2 调整堆
调整堆是指在最大堆中插入或删除元素后,恢复堆的性质。
**插入元素:**
1. 将新元素插入到堆的末尾。
2. 与其父节点比较,如果新元素更大,则交换两者。
3. 重复上述步骤,直到新元素成为最大堆的一部分。
**代码块:**
```python
def insert_element(arr, element):
"""
插入元素。
参数:
arr: 输入数组。
element: 要插入的元素。
"""
arr.append(element)
i = len(arr) - 1
while i > 0 and arr[i] > arr[(i - 1) // 2]:
arr[i], arr[(i - 1) // 2] = arr[(i - 1) // 2], arr[i]
i = (i - 1) // 2
```
**逻辑分析:**
* 将新元素插入到堆的末尾。
* 与其父节点比较,如果新元素更大,则交换两者。
* 重复上述步骤,直到新元素成为最大堆的一部分。
**删除元素:**
1. 将根节点与最后一个元素交换。
2. 删除最后一个元素。
3. 对根节点执行 `max_heapify` 操作,恢复堆的性质。
**代码块:**
```python
def delete_element(arr):
"""
删除根节点。
参数:
arr: 输入数组。
"""
if len(arr) == 0:
return
arr[0], arr[-1] = arr[-1], arr[0]
arr.pop()
max_heapify(arr, 0, len(arr))
```
**逻辑分析:**
* 将根节点与最后一个元素交换。
* 删除最后一个元素。
* 对根节点执行 `max_heapify` 操作,恢复堆的性质。
#### 2.1.3 排序
堆排序的排序过程如下:
1. 构建最大堆。
2. 依次删除根节点,并将其插入到排序后的数组中。
3. 对剩余的堆执行 `max_heapify` 操作,恢复堆的性质。
**代码块:**
```python
def heap_sort(arr):
"""
堆排序。
参数:
arr: 输入数组。
"""
build_max_heap(arr)
for i in range(len(arr) - 1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
max_heapify(arr, 0, i)
```
**逻辑分析:**
* 构建最大堆。
* 依次删除根节点,并将其插入到排序后的数组中。
* 对剩余的堆执行 `max_heapify` 操作,恢复堆的性质。
# 3. 堆排序在树形结构中的应用
### 3.1 堆排序在二叉堆中的应用
#### 3.1.1 二叉堆的定义和性质
二叉堆是一种完全二叉树,其中每个节点的值都大于或等于其子节点的值。它具有以下性质:
- **完全二叉树:**二叉堆中的所有层(除了最后一层)都完全填充,最后一层中的节
0
0
相关推荐
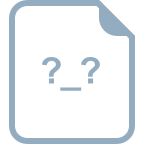





