Dropout Technique and Multilayer Perceptrons (MLPs): Strategies for Overfitting Prevention, Enhancing Model Generalization Ability, and Improving Prediction Accuracy
发布时间: 2024-09-15 08:06:05 阅读量: 37 订阅数: 31 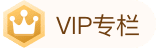
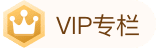
# Dropout Technique and Multilayer Perceptrons (MLPs): Strategies to Combat Overfitting, Enhance Generalization, and Improve Prediction Accuracy
## 1. Theoretical Foundations of the Dropout Technique
Dropout is a widely-used regularization technique in deep learning that improves model generalization by randomly deactivating neurons within the neural network during training.
### 1.1 Random Deactivation Mechanism of Dropout
The principle of Dropout is to randomly discard a portion of neurons during the training process, preventing them from participating in forward and backward propagation. This forces the model to learn more robust features, as it cannot rely on specific neurons.
### 1.2 Hyperparameter Settings for Dropout
The hyperparameter for Dropout is the dropout rate, which determines the proportion of neurons that are dropped out. Typically, the dropout rate ranges from 0.2 to 0.5, depending on the dataset and the complexity of the model.
## 2. Application of the Dropout Technique in Multilayer Perceptrons (MLPs)
### 2.1 Principles and Mechanisms of Dropout in MLPs
#### 2.1.1 Random Deactivation Mechanism of Dropout
Dropout is a regularization technique that prevents overfitting by randomly deactivating some nodes in the neural network during training. In Dropout, each neuron has a probability p of being deactivated in each training batch, which means that the output of that neuron will be set to 0.
**Code Block:**
```python
import numpy as np
def dropout(x, p):
"""
Applies Dropout to the input tensor x.
Parameters:
x: Input tensor.
p: Dropout probability.
"""
# Generate a random mask with the same shape as x, with elements of 0 or 1.
mask = np.random.binomial(1, p, size=x.shape)
# Apply the mask to x, setting the output of deactivated neurons to 0.
return x * mask
```
**Logical Analysis:**
The `dropout` function takes an input tensor `x` and a dropout probability `p`. It generates a random mask with the same shape as `x`, where elements are either 0 or 1, indicating whether a neuron is deactivated. Then, it applies the mask to `x`, setting the output of deactivated neurons to 0.
#### 2.1.2 Hyperparameter Settings for Dropout
The hyperparameter for Dropout is the dropout probability p. Values for p usually range from 0.2 to 0.5. Higher values of p result in stronger regularization but may reduce the accuracy of the model.
### 2.2 Practical Application of Dropout in MLPs
#### 2.2.1 Application of Dropout in MLP Training
In MLP training, Dropout can be applied to hidden layers or the output layer. By randomly deactivating neurons in the hidden layers during training, Dropout prevents overfitting and promotes feature extraction.
**Code Block:**
```python
import tensorflow as tf
# Create an MLP model with Dropout.
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10, activation='softmax')
])
```
0
0