Parallelization and Multi-layer Perceptrons (MLP): Accelerating Training, Enhancing Efficiency, Shortening Model Development Cycle
发布时间: 2024-09-15 08:11:15 阅读量: 28 订阅数: 27 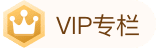
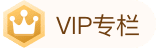
# 1. Introduction to Parallelization and Multilayer Perceptrons (MLPs)
Parallelization is a technique that enhances computational speed by utilizing multiple processing units simultaneously. In machine learning, parallelization is used to accelerate the training of neural networks, including Multilayer Perceptrons (MLPs).
An MLP is a feedforward neural network consisting of multiple hidden layers, each containing several neurons. In traditional training, the weights and biases of each neuron are updated independently, which can result in slow training. Parallelization significantly reduces training time by distributing training tasks across multiple processing units.
# 2. Theoretical Foundations of Parallelizing MLP Training
### 2.1 Data Parallelism and Model Parallelism
**2.1.1 Data Parallelism**
Data parallelism is a technique that divides the training dataset into multiple subsets and processes these subsets in parallel on different computing nodes. Each node is responsible for training a copy of the model using its subset of data. After training, the model parameters from each node are aggregated to produce the final model.
**2.1.2 Model Parallelism**
Model parallelism is a technique that divides the model into multiple sub-models and processes these sub-models in parallel on different computing nodes. Each node is responsible for training a sub-model using the entire training dataset. After training, the sub-model parameters from each node are aggregated to produce the final model.
### 2.2 Communication Optimization
**2.2.1 Communication Patterns**
In parallelized MLP training, there is a significant ***mon communication patterns include:
***Fully connected communication:** Each computing node communicates with every other computing node.
***Ring communication:** Each computing node communicates only with its neighboring computing nodes.
***Tree communication:** Computing nodes are organized into a tree structure, where each node communicates only with its parent and child nodes.
**2.2.2 Communication Optimization Algorithms**
To reduce the communication overhead in parallelized MLP training, the following communication optimization algorithms can be used:
***Parameter Server:** Store model parameters on separate parameter servers, allowing computing nodes to communicate only with the parameter servers.
***Gradient Compression:** Compress gradients before communication to reduce the amount of data transferred.
***Asynchronous Update:** Allow computing nodes to update model parameters at different times to reduce communication latency.
### Code Block 1: Implementation of Data Parallelism
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torch.distributed as dist
# Initialize distributed environment
dist.init_process_group(backend='nccl', init_method='env://')
# Define model
model = nn.Linear(100, 10)
# Parallelize model across different computing nodes
model = nn.parallel.DistributedDataParallel(model)
# Define optimizer
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Data partitioning
train_dataset = ... # Assuming a large dataset
train_sampler = torch.utils.data.distributed.DistributedSampler(train_dataset)
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=16, sampler=train_sampler)
# Train model
for epoch in range(10):
for batch in train_loader:
# Forward propagation
output = model(batch['x'])
# Compute loss
loss = nn.MSELoss(output, batch['y'])
# Backward propagation
loss.backward()
# Update model parameters
optimizer.step()
# Synchronize model parameters
dist.barrier()
```
**Logical Analysis:**
* This code block demonstrates how to perform data-parallel MLP training using PyTorch.
* The `dist.init_process_group()` function initializes the distributed environment.
* The `nn.parallel.DistributedDataParallel()` function parallelizes the model across different computing nodes.
*
0
0
相关推荐
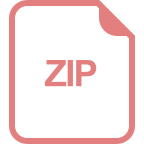
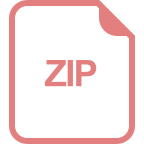
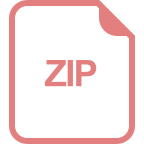





