【进阶篇】使用Matplotlib的子图功能创建多个子图,并进行自定义布局
发布时间: 2024-06-24 16:20:11 阅读量: 122 订阅数: 163 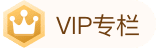
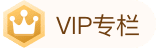
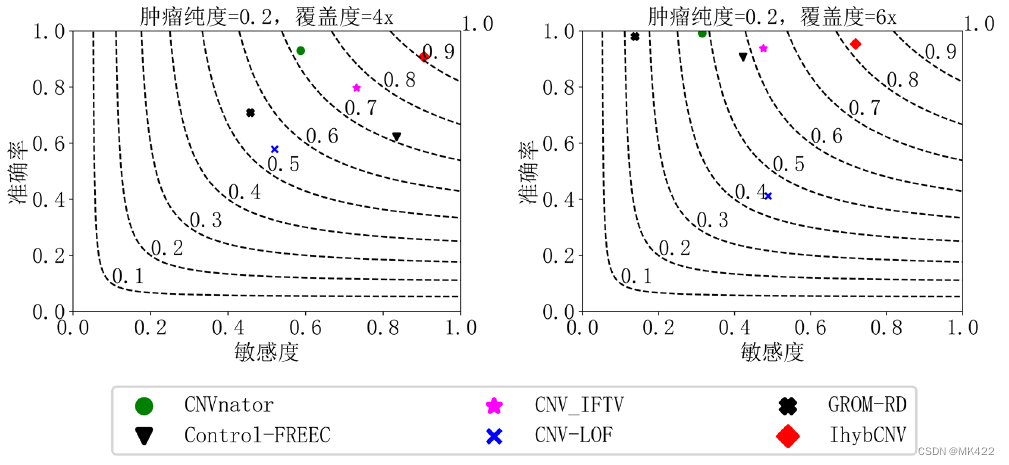
# 2.1 子图的创建和布局
### 2.1.1 subplot()函数
`subplot()`函数是创建子图最基本的方法。它接受三个参数:
- `nrows`:子图的行数
- `ncols`:子图的列数
- `index`:子图在网格中的索引
例如,以下代码创建了一个包含两行两列的子图网格,并激活左上角的子图:
```python
import matplotlib.pyplot as plt
fig, axs = plt.subplots(2, 2)
ax = axs[0, 0]
```
### 2.1.2 gridspec模块
`gridspec`模块提供了更灵活的子图布局方式。它允许创建更复杂的网格结构,并控制子图之间的间距和大小。
以下代码使用`gridspec`模块创建了一个包含两行三列的子图网格,其中第一行的高度是第二行的两倍:
```python
import matplotlib.pyplot as plt
from matplotlib.gridspec import GridSpec
fig = plt.figure()
gs = GridSpec(2, 3, height_ratios=[2, 1])
ax1 = fig.add_subplot(gs[0, :])
ax2 = fig.add_subplot(gs[1, 0])
ax3 = fig.add_subplot(gs[1, 1])
ax4 = fig.add_subplot(gs[1, 2])
```
# 2. 子图布局与定制
### 2.1 子图的创建和布局
#### 2.1.1 subplot()函数
subplot()函数是创建子图的最基本方法。它接受三个参数:
- `nrows`:子图的行数
- `ncols`:子图的列数
- `index`:子图在网格中的索引
例如,以下代码创建了一个包含两个子图的网格,第一个子图位于第一行第一列,第二个子图位于第一行第二列:
```python
import matplotlib.pyplot as plt
# 创建一个包含 2 行 2 列的子图网格
fig, axes = plt.subplots(2, 2)
# 访问第一个子图
ax1 = axes[0, 0]
# 访问第二个子图
ax2 = axes[0, 1]
```
#### 2.1.2 gridspec模块
gridspec模块提供了更灵活的子图布局控制。它允许用户指定子图的大小、位置和间距。
要使用gridspec,需要先创建一个`GridSpec`对象,然后使用`add_subplot()`方法添加子图。
例如,以下代码创建了一个包含两个子图的网格,第一个子图占据网格的左半部分,第二个子图占据右半部分:
```python
import matplotlib.pyplot as plt
from matplotlib.gridspec import GridSpec
# 创建一个包含 1 行 2 列的子图网格
fig = plt.figure()
gs = GridSpec(1, 2)
# 添加第一个子图
ax1 = fig.add_subplot(gs[0, 0])
# 添加第二个子图
ax2 = fig.add_subplot(gs[0, 1])
```
### 2.2 子图的自定义
#### 2.2.1 标题、标签和刻度
可以自定义子图的标题、标签和刻度以提高可读性和信息性。
- **标题:**可以使用`set_title()`方法设置子图的标题。
- **标签:**可以使用`set_xlabel()`和`set_ylabel()`方法设置x轴和y轴的标签。
- **刻度:**可以使用`set_xticks()`和`set_yticks()`方法设置x轴和y轴的刻度。
例如,以下代码设置了子图的标题、x轴标签和y轴标签:
```python
ax.set_title("My Plot")
ax.set_xlabel("X-axis")
ax.set_ylabel("Y-axis")
```
#### 2.2.2 图例和注释
图例和注释可以帮助解释子图中的数据。
- **图例:**可以使用`legend()`方法添加图例。
- **注释:**可以使用`annotate()`方法添加注释。
例如,以下代码添加了一个图例和一个注释:
```python
# 添加图例
ax.legend()
# 添加注释
ax.annotate("This is an annotation", xy=(0.5, 0.5), xytext=(0.6, 0.6))
```
# 3. 子图中的数据可视化
### 3.1 绘制不同类型的图表
#### 3.1.1 折线图和散点图
折线图和散点图是可视化数据趋势和关系的常用图表类型。Matplotlib 提供了 `plot()` 函数来绘制这些图表。
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('折线图')
plt.show()
# 绘制散点图
plt.scatter(x
```
0
0
相关推荐








