高斯赛德尔python
时间: 2024-11-15 13:15:00 浏览: 21
高斯-塞德尔法是一种迭代求解线性方程组的算法,在Python中通常用于数值计算库,如NumPy或SciPy。这个方法基于以下思想:对于一组系数矩阵A和向量b构成的Ax=b系统,从当前的猜测解开始,逐步更新每个未知数的值,使其更接近真实的解。
在Python中,你可以通过以下步骤实现高斯-塞德尔法:
```python
import numpy as np
# 定义函数来应用高斯-塞德尔法
def gauss_seidel(A, b, iterations, tolerance=1e-6):
# 检查输入的有效性
if not (np.allclose(np.diag(A), A.diagonal()) and np.linalg.norm(A - A.T) < 1e-8):
raise ValueError("Matrix must be diagonally dominant for Gauss-Seidel to converge.")
n = len(b)
x = np.zeros(n) # 初始猜测解
delta = np.inf # 当前误差
for _ in range(iterations):
# 计算下一个猜测值
for i in range(n):
sum_temp = np.sum(A[i, :i] * x[:i]) + np.sum(A[i, i+1:] * x[i+1:])
x[i] = (b[i] - sum_temp) / A[i, i]
# 更新误差
delta_new = np.max(np.abs(x - x_previous)) # x_previous保存上一次迭代的解
if delta_new < tolerance:
break
else:
delta = delta_new
x_previous = x.copy()
return x, delta
# 示例用法
A = np.array([[4, 1], [1, 3]])
b = np.array([5, 7])
solution, convergence_rate = gauss_seidel(A, b, 100)
print(f"Solution: {solution}")
print(f"Gauss-Seidel converged after {iterations} iterations with error of {convergence_rate}")
```
阅读全文
相关推荐
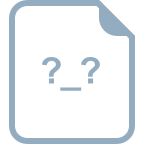
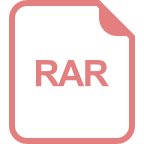
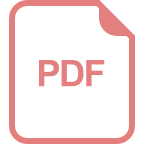















