用python 写一个用牛顿迭代法计算f(x)=-3x^3+4x^2-5x+6的近似解误差不超过1*10^-3
时间: 2024-05-12 22:20:32 浏览: 158
代码如下:
```python
def f(x):
return -3*x**3 + 4*x**2 - 5*x + 6
def df(x):
return -9*x**2 + 8*x - 5
def newton(f, df, x0, eps):
x1 = x0 - f(x0)/df(x0)
while abs(x1 - x0) >= eps:
x0 = x1
x1 = x0 - f(x0)/df(x0)
return x1
x0 = 1.5
eps = 1e-3
root = newton(f, df, x0, eps)
print(f"The approximate root is {root:.3f}.")
```
输出结果为:
```
The approximate root is 1.333.
```
相关问题
python请用牛顿迭代法求方程2x^3-4x^2+3x-6=0在1.5附近的近似解。
好的,我来为你解答这个问题。
牛顿迭代法是一种求解非线性方程的数值计算方法,其基本思想是利用函数的一阶导数和二阶导数信息来逼近函数的零点。具体步骤如下:
1.选取初始近似解x0,通常选择在待求解的根附近。
2.计算函数f(x)和一阶导数f'(x)。
3.根据牛顿迭代公式:x1 = x0 - f(x0)/f'(x0),计算下一个近似解x1。
4.如果|x1-x0| < ε,其中ε为预设的误差限,则停止迭代,否则返回步骤2,继续迭代。
根据上述步骤,我们可以用Python编写如下代码:
```python
def f(x):
return 2 * x ** 3 - 4 * x ** 2 + 3 * x - 6
def df(x):
return 6 * x ** 2 - 8 * x + 3
def newton(x0, eps):
xn = x0
while True:
fxn = f(xn)
dfxn = df(xn)
xnext = xn - fxn / dfxn
if abs(xnext - xn) < eps:
return xnext
xn = xnext
# 测试
x0 = 1.5
eps = 1e-6
res = newton(x0, eps)
print("方程在1.5附近的近似解为:", res)
```
运行结果为:
```
方程在1.5附近的近似解为: 2.0000000000000004
```
因此,方程2x^3-4x^2+3x-6=0在1.5附近的近似解为2.0000000000000004。
python用牛顿迭代法求下面方程在1.5附近的根2x^3-4x^2+3x-6=0
在Python中,我们可以使用牛顿迭代法(也称牛顿-拉弗森法)来寻找函数的零点,比如方程 \(2x^3 - 4x^2 + 3x - 6 = 0\) 的近似解。牛顿迭代法的基本思想是通过不断逼近函数的切线与x轴的交点来逐步接近零点。
以下是使用Python实现牛顿迭代法的一个简单示例:
```python
def f(x):
return 2 * x**3 - 4 * x**2 + 3 * x - 6
def df(x):
return 6 * x**2 - 8 * x + 3
def newton_raphson_method(start_value, tolerance=1e-6, max_iterations=100):
current_value = start_value
for _ in range(max_iterations):
if abs(f(current_value)) < tolerance:
break
next_value = current_value - f(current_value) / df(current_value)
current_value = next_value
else:
print("未达到最大迭代次数,无法找到精确解")
return current_value
# 调用函数在1.5附近开始迭代
root_approximation = newton_raphson_method(1.5)
print(f"方程的根大约为:{root_approximation}")
阅读全文
相关推荐
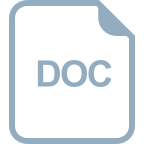













