用gpio写stm32 4*4矩阵键盘代码
时间: 2023-12-09 11:04:11 浏览: 101
以下是使用GPIO编写的STM32 4x4矩阵键盘代码,假设矩阵键盘的行线连接到GPIOB的0-3引脚,列线连接到GPIOB的4-7引脚:
```c
#include "stm32f10x.h"
// 矩阵键盘的按键状态,0表示未按下,1表示按下
uint8_t keyState[4][4] = {0};
// 初始化GPIO
void initGPIO() {
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOB时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置GPIOB的0-3引脚为推挽输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 配置GPIOB的4-7引脚为上拉输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
// 读取矩阵键盘状态
void readKeypad() {
// 循环扫描每一列
for (int col = 0; col < 4; col++) {
// 将当前列置为低电平,其他列置为高电平
GPIOB->ODR &= ~(0xf << 4);
GPIOB->ODR |= (1 << (4 + col));
// 延时一段时间,等待行电平稳定
for (volatile int i = 0; i < 100; i++);
// 读取每一行的状态
for (int row = 0; row < 4; row++) {
uint8_t state = (GPIOB->IDR & (1 << row)) ? 0 : 1;
if (state != keyState[row][col]) {
// 按键状态发生改变,触发事件
if (state == 1) {
// 按下按键
// TODO: 处理按键按下事件
} else {
// 松开按键
// TODO: 处理按键松开事件
}
keyState[row][col] = state;
}
}
}
}
int main() {
initGPIO();
while (1) {
readKeypad();
}
return 0;
}
```
代码中使用了循环扫描的方式读取矩阵键盘的状态,每次扫描一列,将当前列置为低电平,其他列置为高电平,并读取每一行的状态。如果某个按键状态发生改变,则触发相应的事件。注意需要在`TODO`部分处理按键事件。
阅读全文
相关推荐




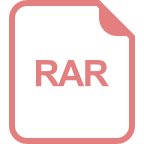
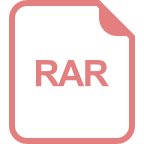
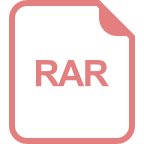
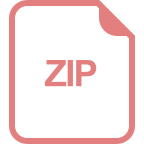
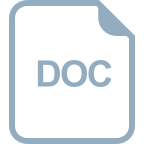



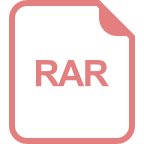
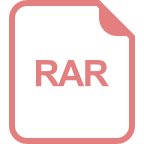

