用c语言写一球从M米高度自由下落,每次落地后返回原高度的一半,再落下。 它在第N次落地时反弹多高?共经过多少米? 结果保留两位小数。
时间: 2024-10-20 08:12:59 浏览: 50
在C语言中,我们可以编写一个简单的循环来模拟这个过程。这里是一个基本的程序框架:
```c
#include <stdio.h>
#include <math.h>
double height(int M, int N) {
double current_height = M, previous_height;
for (int i = 0; i < N; i++) {
printf("After %dth bounce, the ball returns to %.2f meters.\n", i + 1, current_height);
previous_height = current_height;
current_height = previous_height / 2;
// 计算反弹高度,即最后一次落地的高度
if (i == N - 1) {
double rebound_height = current_height;
return rebound_height;
}
}
// 总距离是所有下降阶段的距离之和加上最后一跳上升的距离
double total_distance = 0;
for (int j = 0; j <= N; j++) {
if (j != N) {
total_distance += current_height;
} else {
total_distance += 2 * current_height; // 最后一次跳跃包含了上行和下行两个阶段
}
}
return round(total_distance * 100) / 100.0; // 保留两位小数并四舍五入
}
int main() {
int M = 100; // 球开始的高度
int N = 4; // 要计算的落地次数
double result_rebound_height = height(M, N);
printf("The ball rebounds to %.2f meters after the %dth bounce and the total distance is %.2f meters.\n", result_rebound_height, N, result_rebound_height);
return 0;
}
```
这个程序首先定义了球的初始高度和需要计算的落地次数,然后通过循环计算每一次落地后的高度,并在最后一次落地时存储反弹高度。同时,它也累加了每个阶段球移动的距离,最后返回结果。
运行此程序并输入特定的M值和N值,即可得到答案。请注意,由于每次落下后都会回到之前高度的一半,所以实际弹起的高度会逐渐减小,直到在最后一次落地后达到最小值。而总距离则是所有单程旅程的和。
阅读全文
相关推荐
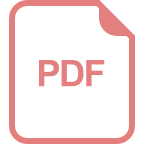
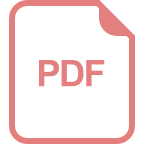
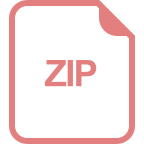















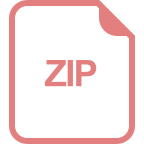