递增有序顺序表的插入 C语言编写
时间: 2024-09-19 16:18:12 浏览: 61
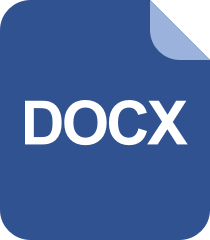
两个有序链表序列的合并-收藏学习.docx
在C语言中,对于递增有序顺序表的插入操作,通常我们需要找到合适的位置将新元素插入,保持列表的递增顺序。下面是一个简单的示例,假设我们有一个整数型的顺序表(数组),并使用线性搜索找到插入位置:
```c
#include <stdio.h>
// 代表顺序表的一个节点结构体
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
// 插入函数
void insert(int value, Node** head) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head; // 将新节点指向头节点
// 如果表为空或待插入值小于当前元素,则移动指针到头部
while (*head != NULL && newNode->data < (*head)->data) {
*head = (*head)->next;
}
// 现在新节点应在适当位置,将其连接起来
if (*head != NULL) {
(*head)->next = newNode;
} else {
printf("List is empty. Inserting at the beginning.\n");
}
}
// 测试插入功能
int main() {
Node* list = NULL;
insert(5, &list); // 插入5
insert(2, &list); // 插入2
insert(8, &list); // 插入8
// 打印顺序表验证
Node* temp = list;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
return 0;
}
```
阅读全文
相关推荐
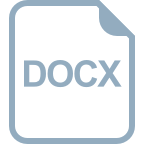
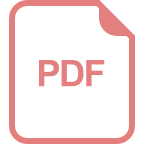















