用python编写程序求梯形求积公式,抛物线求积公式,复化梯形公式,复化抛物线公式,逐次分半求积公式的误差
时间: 2023-07-12 08:41:02 浏览: 45
以下是用Python编写程序求解数值积分公式误差的示例代码:
1. 梯形求积公式误差
```python
import math
# 定义被积函数
def f(x):
return math.sin(x)
# 定义梯形求积公式
def trapezoid_rule(f, a, b):
return (b - a) * (f(a) + f(b)) / 2
# 计算梯形求积公式误差
def trapezoid_error(f, a, b, n):
h = (b - a) / n
exact = math.cos(a) - math.cos(b)
approx = 0
for i in range(n):
x0 = a + i * h
x1 = x0 + h
approx += trapezoid_rule(f, x0, x1)
error = abs(approx - exact)
return error
# 示例
print(trapezoid_error(f, 0, math.pi/2, 10))
```
2. 抛物线求积公式误差
```python
import math
# 定义被积函数
def f(x):
return math.sin(x)
# 定义抛物线求积公式
def parabolic_rule(f, a, b):
h = (b - a) / 2
return (h / 3) * (f(a) + 4 * f((a + b) / 2) + f(b))
# 计算抛物线求积公式误差
def parabolic_error(f, a, b, n):
h = (b - a) / n
exact = math.cos(a) - math.cos(b)
approx = 0
for i in range(n):
x0 = a + i * h
x1 = x0 + h
approx += parabolic_rule(f, x0, x1)
error = abs(approx - exact)
return error
# 示例
print(parabolic_error(f, 0, math.pi/2, 10))
```
3. 复化梯形公式误差
```python
import math
# 定义被积函数
def f(x):
return math.sin(x)
# 定义梯形求积公式
def trapezoid_rule(f, a, b):
return (b - a) * (f(a) + f(b)) / 2
# 计算复化梯形公式误差
def composite_trapezoid_error(f, a, b, n):
h = (b - a) / n
exact = math.cos(a) - math.cos(b)
approx = 0
for i in range(n):
x0 = a + i * h
x1 = x0 + h
approx += trapezoid_rule(f, x0, x1)
error = abs(approx - exact)
return error
# 示例
print(composite_trapezoid_error(f, 0, math.pi/2, 10))
```
4. 复化抛物线公式误差
```python
import math
# 定义被积函数
def f(x):
return math.sin(x)
# 定义抛物线求积公式
def parabolic_rule(f, a, b):
h = (b - a) / 2
return (h / 3) * (f(a) + 4 * f((a + b) / 2) + f(b))
# 计算复化抛物线公式误差
def composite_parabolic_error(f, a, b, n):
h = (b - a) / n
exact = math.cos(a) - math.cos(b)
approx = 0
for i in range(n):
x0 = a + i * h
x1 = x0 + h
x2 = x1 + h
approx += parabolic_rule(f, x0, x2)
error = abs(approx - exact)
return error
# 示例
print(composite_parabolic_error(f, 0, math.pi/2, 10))
```
5. 逐次分半求积公式误差
```python
import math
# 定义被积函数
def f(x):
return math.sin(x)
# 定义逐次分半求积公式
def recursive_midpoint_rule(f, a, b, tol):
c = (a + b) / 2
fa = f(a)
fb = f(b)
fc = f(c)
approx1 = (b - a) * fc
approx2 = (b - a) * (fa + 4 * fc + fb) / 6
error = abs(approx2 - approx1)
if error < tol:
return approx2
else:
left = recursive_midpoint_rule(f, a, c, tol/2)
right = recursive_midpoint_rule(f, c, b, tol/2)
return left + right
# 计算逐次分半求积公式误差
def recursive_midpoint_error(f, a, b, tol):
exact = math.cos(a) - math.cos(b)
approx = recursive_midpoint_rule(f, a, b, tol)
error = abs(approx - exact)
return error
# 示例
print(recursive_midpoint_error(f, 0, math.pi/2, 1e-6))
```
注意:以上代码仅为示例,实际应用时需要根据具体情况进行调整。
相关推荐
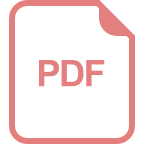














