c++实现三次bezier曲线动画
时间: 2023-09-14 14:12:06 浏览: 42
三次贝塞尔曲线是一种平滑的曲线,由4个控制点定义,其中第一个和第四个点是曲线的端点,第二个和第三个点是控制点。在C++中实现三次贝塞尔曲线动画的步骤如下:
1. 定义4个点,包括起点、终点和两个控制点。
```c++
const int NUM_POINTS = 4;
const int START_X = 50, START_Y = 50;
const int END_X = 400, END_Y = 400;
const int CONTROL1_X = 100, CONTROL1_Y = 200;
const int CONTROL2_X = 300, CONTROL2_Y = 300;
```
2. 使用循环计算曲线上的点,并将其保存在数组中。
```c++
const int NUM_SEGMENTS = 100;
int curvePoints[NUM_SEGMENTS][2];
for (int i = 0; i < NUM_SEGMENTS; i++) {
float t = (float)i / (float)(NUM_SEGMENTS-1);
float oneMinusT = 1.0 - t;
float oneMinusTSquared = oneMinusT * oneMinusT;
float oneMinusTCubed = oneMinusTSquared * oneMinusT;
float tSquared = t * t;
float tCubed = tSquared * t;
float x = oneMinusTCubed * START_X +
3 * oneMinusTSquared * t * CONTROL1_X +
3 * oneMinusT * tSquared * CONTROL2_X +
tCubed * END_X;
float y = oneMinusTCubed * START_Y +
3 * oneMinusTSquared * t * CONTROL1_Y +
3 * oneMinusT * tSquared * CONTROL2_Y +
tCubed * END_Y;
curvePoints[i][0] = (int)x;
curvePoints[i][1] = (int)y;
}
```
3. 在循环中将曲线上的点绘制到屏幕上。
```c++
for (int i = 0; i < NUM_SEGMENTS-1; i++) {
int x1 = curvePoints[i][0], y1 = curvePoints[i][1];
int x2 = curvePoints[i+1][0], y2 = curvePoints[i+1][1];
line(x1, y1, x2, y2);
}
```
完整的代码示例如下:
```c++
#include <graphics.h>
const int NUM_POINTS = 4;
const int START_X = 50, START_Y = 50;
const int END_X = 400, END_Y = 400;
const int CONTROL1_X = 100, CONTROL1_Y = 200;
const int CONTROL2_X = 300, CONTROL2_Y = 300;
const int NUM_SEGMENTS = 100;
int curvePoints[NUM_SEGMENTS][2];
int main() {
initwindow(500, 500);
// 计算曲线上的点
for (int i = 0; i < NUM_SEGMENTS; i++) {
float t = (float)i / (float)(NUM_SEGMENTS-1);
float oneMinusT = 1.0 - t;
float oneMinusTSquared = oneMinusT * oneMinusT;
float oneMinusTCubed = oneMinusTSquared * oneMinusT;
float tSquared = t * t;
float tCubed = tSquared * t;
float x = oneMinusTCubed * START_X +
3 * oneMinusTSquared * t * CONTROL1_X +
3 * oneMinusT * tSquared * CONTROL2_X +
tCubed * END_X;
float y = oneMinusTCubed * START_Y +
3 * oneMinusTSquared * t * CONTROL1_Y +
3 * oneMinusT * tSquared * CONTROL2_Y +
tCubed * END_Y;
curvePoints[i][0] = (int)x;
curvePoints[i][1] = (int)y;
}
// 绘制曲线
for (int i = 0; i < NUM_SEGMENTS-1; i++) {
int x1 = curvePoints[i][0], y1 = curvePoints[i][1];
int x2 = curvePoints[i+1][0], y2 = curvePoints[i+1][1];
line(x1, y1, x2, y2);
}
getch();
closegraph();
return 0;
}
```
相关推荐
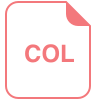













